JavaScript is a programming language widely used in web development. It provides many built-in functions and methods for working with various data types such as strings, mathematical operations, arrays, objects, dates and times. In this article, we will discuss some important internal methods of JavaScript.
1. String method
- indexOf(string) method: This method returns the index value of the first occurrence of the specified string in the string. If the specified string does not exist, Returns -1.
Example:
let str = "Hello World!"; let result = str.indexOf("World"); console.log(result); // 输出6
- slice(beginIndex, endIndex) method: This method returns a new string, including the original string from beginIndex to endIndex (exclusive) characters between. If endIndex is omitted, it goes from beginIndex to the end of the string.
Example:
let str = "Hello World!"; let result = str.slice(0, 5); console.log(result); // 输出Hello
- replace(oldString, newString) method: This method replaces the specified string in the original string with a new string and returns a new character string.
Example:
let str = "Hello World!"; let result = str.replace("World", "JavaScript"); console.log(result); // 输出Hello JavaScript!
2. Mathematical methods
- Math.round(number) method: This method rounds the specified number to the nearest integer.
Example:
let num = 3.141592654; let result = Math.round(num); console.log(result); // 输出3
- Math.floor(number) method: This method returns the largest integer less than or equal to the specified number.
Example:
let num = 3.141592654; let result = Math.floor(num); console.log(result); // 输出3
- Math.random() method: This method returns a random number between 0 and 1.
Example:
let result = Math.random(); console.log(result); // 输出0到1之间的随机数
3. Array method
- push(element) method: This method adds one or more elements to the end of the array. and returns the new array length.
Example:
let array = [1, 2, 3]; let result = array.push(4, 5); console.log(array); // 输出[1, 2, 3, 4, 5] console.log(result); // 输出5
- pop() method: This method deletes the element at the end of the array and returns the deleted element.
Example:
let array = [1, 2, 3]; let result = array.pop(); console.log(array); // 输出[1, 2] console.log(result); // 输出3
- splice(startIndex, deleteCount, element1, element2, ...) method: This method is used to delete elements from the array and can be replaced Or add new elements. The parameter startIndex defines the starting index of the element to be deleted. deleteCount defines the number of elements to be deleted. The following parameters element1, element2, etc. are the elements to be added to the array.
Example:
let array = [1, 2, 3, 4, 5]; let result = array.splice(2, 2, 6, 7); console.log(array); // 输出[1, 2, 6, 7, 5] console.log(result); // 输出[3, 4]
4. Object method
- Object.keys(object) method: This method returns an array containing all the keys of the object Property name.
Example:
let obj = { name: "Tom", age: 18, gender: "Male" }; let result = Object.keys(obj); console.log(result); // 输出[name, age, gender]
- Object.values(object) method: This method returns an array containing all property values of the object.
Example:
let obj = { name: "Tom", age: 18, gender: "Male" }; let result = Object.values(obj); console.log(result); // 输出[Tom, 18, Male]
- Object.assign(target, source) method: This method merges the properties of multiple objects into one target object. If a property with the same name already exists in the target object, the value of the property is overwritten.
Example:
let target = { name: "Alice", age: 20 }; let source = { name: "Bob", gender: "Male" }; let result = Object.assign(target, source); console.log(result); // 输出{name: "Bob", age: 20, gender: "Male"}
5. Date and time methods
- new Date() method: This method is used to create a Date representing the current time object.
Example:
let now = new Date(); console.log(now); // 输出表示当前时间的Date对象
- Date.parse(string) method: This method converts a date and time string into a millisecond-level timestamp.
Example:
let timestamp = Date.parse("2022-01-01T00:00:00"); console.log(timestamp); // 输出所表示日期时间的毫秒级时间戳
- Date.getFullYear() method: This method returns the year representing the date.
Example:
let now = new Date(); let year = now.getFullYear(); console.log(year); // 输出当前年份
The above introduces several internal methods of JavaScript, which are important tools for writing JavaScript programs. Mastering these methods will not only help improve programming efficiency, but also help write more efficient code. In practical applications, we should choose appropriate methods according to specific needs and use them rationally.
The above is the detailed content of javascript internal method. For more information, please follow other related articles on the PHP Chinese website!
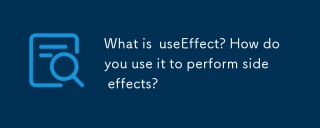
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
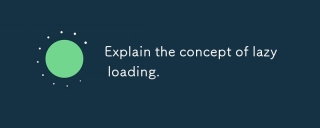
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
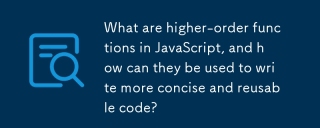
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
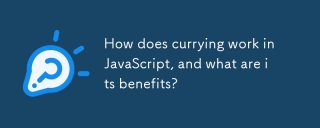
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
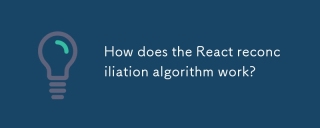
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
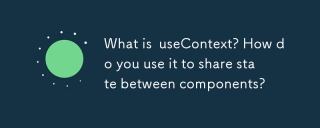
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
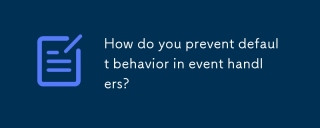
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
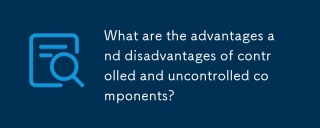
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
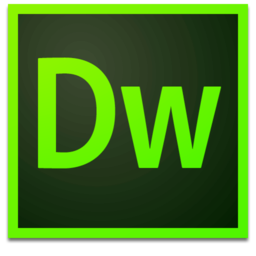
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
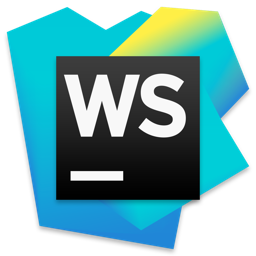
WebStorm Mac version
Useful JavaScript development tools
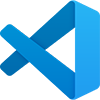
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
