When programming in Golang, sometimes you may encounter the need to remove newline characters from a string. The newline character refers to the special symbol that represents a newline in a string. Common ones are
and
. When processing data or strings, these newline symbols will affect the parsing and processing of data and need to be removed in some way. This article will introduce several ways to remove line breaks in Golang.
1. strings.Replace method
The strings package in Golang provides a Replace method that can be used to replace specific characters or character sets in a string. Using this method, you can easily replace newline characters with spaces or other characters. The following is an example code for using the Replace method to remove newlines in a string:
package main import ( "fmt" "strings" ) func main() { str := "Hello World " fmt.Println("原字符串:", str) fmt.Println("去掉换行:", strings.Replace(str, " ", "", -1)) }
Run the above code, the output result is:
原字符串:Hello World 去掉换行:HelloWorld
When using the Replace method, you need to pay attention to the target character or characters to be replaced. The set needs to completely match the newline character in the string, otherwise it cannot be replaced normally. At the same time, since the strings.Replace method replaces all matching items that appear in the string, the last -1 means matching all items.
2. strings.Trim method
In addition to the Replace method, Golang's strings package also provides a Trim method, which can be used to remove specific characters or character sets at the beginning and end of a string. You can also use this method to remove newlines in a string. The sample code is as follows:
package main import ( "fmt" "strings" ) func main() { str := "Hello World " fmt.Println("原字符串:", str) fmt.Println("去掉换行:", strings.Trim(str, " ")) }
Run the above code, the output result is also:
原字符串:Hello World 去掉换行:HelloWorld
The second parameter in the Trim method indicates the characters or character sets that need to be removed. Multiple characters can be specified. Separate each character with a comma. When removing leading and trailing spaces from a string, you can use strings.Trim(str, " ").
3. bytes.Replace method
In addition to the methods provided by the strings package, the bytes package also provides some similar methods that can be used to operate byte type data. Similar to the strings.Replace method, the bytes package's Replace method can also be used to replace specific characters or character sets in byte data. The sample code is as follows:
package main import ( "bytes" "fmt" ) func main() { str := "Hello World " b := []byte(str) fmt.Println("原字节:", b) fmt.Println("去掉换行:", string(bytes.Replace(b, []byte(" "), []byte(""), -1))) }
The output result is also:
原字节:[72 101 108 108 111 10 87 111 114 108 100 10] 去掉换行:HelloWorld
In the above code, the string is first converted into a byte array, and then replaced using the bytes.Replace method. Since byte data needs to be replaced, the characters to be replaced need to be specified as byte array type, and the replacement result also needs to be converted to string type.
4. ReplaceAll method of regexp package
In addition to the methods provided by the above two packages, Golang's regexp package also provides a ReplaceAll method, which can be used to replace strings based on regular expressions. a specific character or set of characters in . More flexible string processing can be achieved through the regexp package. The sample code is as follows:
package main import ( "fmt" "regexp" ) func main() { str := "Hello World " reg, _ := regexp.Compile(" ") fmt.Println("原字符串:", str) fmt.Println("去掉换行:", reg.ReplaceAllString(str, "")) }
The output result is also:
原字符串:Hello World 去掉换行:HelloWorld
In the above code, the Compile method of the regexp package is first used to create a regular expression object, and then the ReplaceAllString method of the object is used to modify the characters. String replacement operation. Since the ReplaceAllString method already performs replacement based on regular expressions, there is no need to specify the replacement character set, but directly uses "
" as the regular expression.
Summary
The above introduces several ways to remove newlines in Golang: using the Replace and Trim methods of the strings package, using the Replace method of the bytes package, and using the ReplaceAll method of the regexp package. Each method has its own advantages and disadvantages, and the appropriate method needs to be selected based on actual needs for specific applications. At the same time, it should be noted that the replacement character or character set needs to match the actual character in the string, otherwise it cannot be replaced normally.
The above is the detailed content of golang remove newlines. For more information, please follow other related articles on the PHP Chinese website!
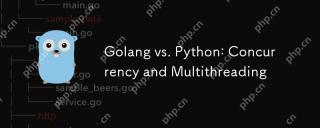
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
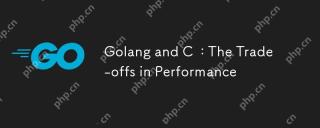
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
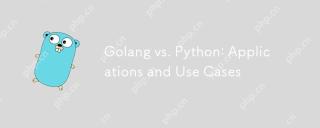
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
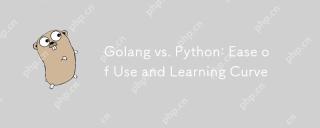
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
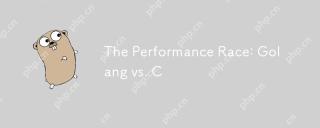
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
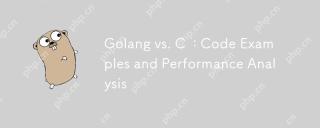
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
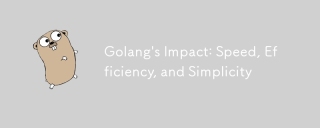
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
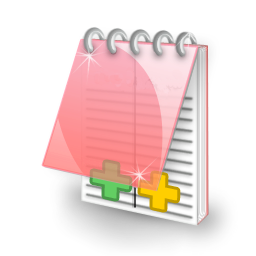
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.