Vue3 global component communication provide/inject source code analysis
1. Foreword
As the name suggests, the grandfather-grandson component has a deeper reference relationship than the communication between parent-child components (also called "generation-separated components"):
C component is introduced into In component B, component B is introduced into component A for rendering. At this time, A is the grandpa level of C (there may be more hierarchical relationships). If you use props, you can only pass them level by level, which is too cumbersome. , so we need a more direct method of communication.
The relationship between them is as follows. Grandson.vue is not directly mounted under Grandfather.vue. There is at least one Son.vue between them (there may be multiple):
Grandfather.vue └─Son.vue └─Grandson.vue
Because of the consistency of the relationship between superiors and subordinates, the scheme of grandfather-grandson component communication is also applicable to parent-child component communication. You only need to replace the grandfather-grandson relationship with a father-son relationship.
2. provide / inject
This feature has two parts: Grandfather.vue has a provide option to provide data, and Grandson.vue has an inject option to start using the data. .
Grandfather.vue passes value to Grandson.vue through provide (can include defined functions)
Grandson.vue passes value to Grandfather through inject .vue triggers the event execution of the grandpa component
No matter how deep the component hierarchy is, the component that initiates provide can be used as a dependency provider for all its subordinate components
The content of this part The changes are very big, but it is actually very simple to use. Don't panic, there are also the same places:
The parent component does not need to know which child components use the property it provides
Subcomponents do not need to know where the inject property comes from
In addition, one thing to remember is that the provide and inject bindings are not responsive. This is intentional, but if a listenable object is passed in, the object's properties will still be responsive.
3. Initiate provide
Let’s first review the usage of 2.x:
export default { // 定义好数据 data () { return { tags: [ '中餐', '粤菜', '烧腊' ] } }, // provide出去 provide () { return { tags: this.tags } } }
The old version of provide usage is similar to data, both are configured as a return object function.
3.x’s new version of provide is quite different in usage from 2.x.
In 3.x, provide needs to be imported and enabled in setup, and is now a completely new method.
Every time you want to provide a piece of data, you must call it separately.
Every time you call, you need to pass in 2 parameters:
Parameters | Type | Description |
---|---|---|
key | string | The name of the data |
any | Value of data |
// 记得导入provide import { defineComponent, provide } from 'vue' export default defineComponent({ // ... setup () { // 定义好数据 const msg: string = 'Hello World!'; // provide出去 provide('msg', msg); } })The operation is very simple, right, but it should be noted that provide is not a response style, if you want to make it responsive, you need to pass in responsive data 4, receive inject Let’s first review the usage of 2.x:
export default { inject: ['tags'], mounted () { console.log(this.tags); } }The usage of the old version of inject is similar to that of props. The usage of the new version of 3.x inject is also quite different from that of 2.x. In 3.x, inject is the same as provide. It also needs to be imported first and then enabled in setup. It is also a brand new method.
Every time you want to inject a piece of data, you must call it separately.
Every time you call, you only need to pass in 1 parameter:
Type | Description | |
---|---|---|
string | The data name corresponding to provide |
The above is the detailed content of Vue3 global component communication provide/inject source code analysis. For more information, please follow other related articles on the PHP Chinese website!

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
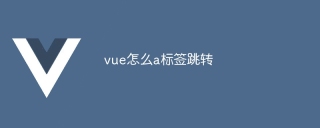
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
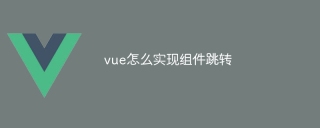
There are the following methods to implement component jump in Vue: use router-link and <router-view> components to perform hyperlink jump, and specify the :to attribute as the target path. Use the <router-view> component directly to display the currently routed rendered components. Use the router.push() and router.replace() methods for programmatic navigation. The former saves history and the latter replaces the current route without leaving records.
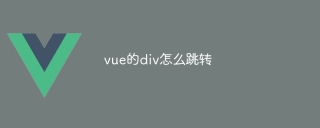
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
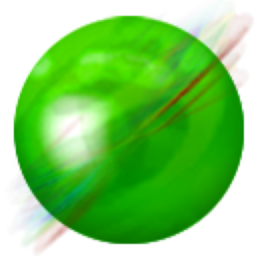
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
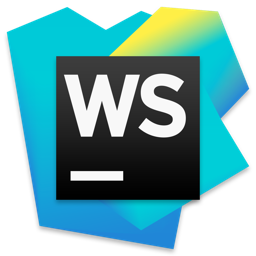
WebStorm Mac version
Useful JavaScript development tools
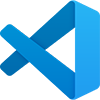
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft