setup syntactic sugar
The biggest advantage is that all declaration parts can be used directly without return.
Note: Some functions are not yet complete, such as: name and render need to be added separately. Tags are written according to the compositionAPI method
// You can also add <script></script>
setup syntactic sugar unique
<script setup> import { ref ,reactive,toRefs } from 'vue' const a = 1; const num = ref(99) // 基本数据类型 const user = reactive({ // 引用数据类型 age:11 }) // 解构能获取响应式属性 {}解构 toRefs保留响应式 const { age } = toRefs(user) // 导出 defineExpose({ a }) // props const props = defineProps({ foo: String }) // 事件 const emit = defineEmits(['change', 'delete']) // 自定义指令 const vMyDirective = { created(el, binding, vnode, prevVnode) { // 下面会介绍各个参数的细节 console.log('创建了') }, } </script>
defineProps under setup defineEmits is related to the component application
// 子组件 <template> <div class="hello"> <h2 id="nbsp-msg-nbsp">{{ msg }}</h2> <slot name="btn"> </slot> <button @click="chickMe"></button> </div> </template> <script setup> import { useSlots, useAttrs } from 'vue'; const slots = useSlots() const attrs = useAttrs() const props = defineProps({ msg: String }) const emit = defineEmits(['change']) console.log(slots, attrs) const chickMe = ()=>{ emit('change','abc') } </script> // 父组件 <template> <div class="home" > <HelloWorld msg="hello" atr="attrs" @change="changeP "> <template #btn> <div>我是 btn:{{ obj.text }}</div> </template> </HelloWorld> </div> </template> <script setup> import HelloWorld from '../components/HelloWorld.vue'; import { ref ,reactive,toRefs } from 'vue' const obj = reactive({ id: 0, text: '小红' }) const changeP=(e)=>{ console.log(e) } </script> 、
defineExpose is related to the component application
// 子组件 <template> <div class="hello"> 123 </div> </template> <script setup> const testPose =()=>{ console.log('子组件暴露方法') } defineExpose({ testPose }) </script> // 父组件 <template> <div class="home" v-test> <HelloWorld ref="helloSon"></HelloWorld> <button @click="testEpose"></button> </div> </template> <script setup> import HelloWorld from '../components/HelloWorld.vue'; import { ref } from 'vue' // setup函数的话可以从context上查找 const helloSon = ref(null); const testEpose = () => { helloSon.value.testPose(); } </script>
custom directive
created: in Called before the bound element's attribute or event listener is applied. This is useful when a directive needs to be appended to an event listener before the normal v-on event listener is called.
beforeMount: Called when the directive is bound to the element for the first time and before the parent component is mounted.
mounted: Called after the parent component of the bound element is mounted. Most of the custom instructions are written here.
beforeUpdate: Called before updating the VNode containing the component.
updated: Called after the containing component’s VNode and the VNodes of its subcomponents are updated.
beforeUnmount: Called before unmounting the parent component of the bound element
unmounted: When the directive is unbound from the element and the parent component has been unmounted , it is only called once.
import { createApp } from 'vue'; const Test = createApp(); Test.directive('my-directive', { // 在绑定元素的 attribute 前 // 或事件监听器应用前调用 created(el, binding, vnode, prevVnode) { // 下面会介绍各个参数的细节 console.log('创建了') }, // 在元素被插入到 DOM 前调用 beforeMount(el, binding, vnode, prevVnode) { }, // 在绑定元素的父组件 // 及他自己的所有子节点都挂载完成后调用 mounted(el, binding, vnode, prevVnode) { }, // 绑定元素的父组件更新前调用 beforeUpdate(el, binding, vnode, prevVnode) { }, // 在绑定元素的父组件 // 及他自己的所有子节点都更新后调用 updated(el, binding, vnode, prevVnode) { }, // 绑定元素的父组件卸载前调用 beforeUnmount(el, binding, vnode, prevVnode) { }, // 绑定元素的父组件卸载后调用 unmounted(el, binding, vnode, prevVnode) { } }) export default Test.directive('my-directive');
el
: The element to which the directive is bound. This can be used to directly manipulate the DOM.binding
: An object containing the following properties.value
: The value passed to the directive. For example, inv-my-directive="1 1"
, the value is2
.oldValue
: The previous value, only available inbeforeUpdate
andupdated
. It is available whether the value changes or not.arg
: The arguments passed to the directive (if any). For example, inv-my-directive:foo
, the parameter is"foo"
.modifiers
: An object containing modifiers (if any). For example, inv-my-directive.foo.bar
, the modifier object is{ foo: true, bar: true }
.instance
: The component instance using this directive.dir
: The definition object of the instruction.vnode
: Represents the underlying VNode of the bound element.prevNode
: The VNode representing the element to which the directive is bound in the previous rendering. Only available inbeforeUpdate
andupdated
hooks.
Application
<template> <div class="home" v-test> </div> </template> //setup 外部调用 <script> // 指令必须 vXxx 这样书写 import vTest from './TestDirective' export default defineComponent({ directives: { test:vTest, }, setup(props) { // console.log('Test',vTest) return { }; } }) </script> //或者 setup内部 <script setup> import vTest from './TestDirective' </script>
Object literal
<div v-demo="{ color: 'white', text: 'hello!' }"></div> app.directive('demo', (el, binding) => { console.log(binding.value.color) // => "white" console.log(binding.value.text) // => "hello!" })
The above is the detailed content of How to use setup and custom instructions in Vue3. For more information, please follow other related articles on the PHP Chinese website!
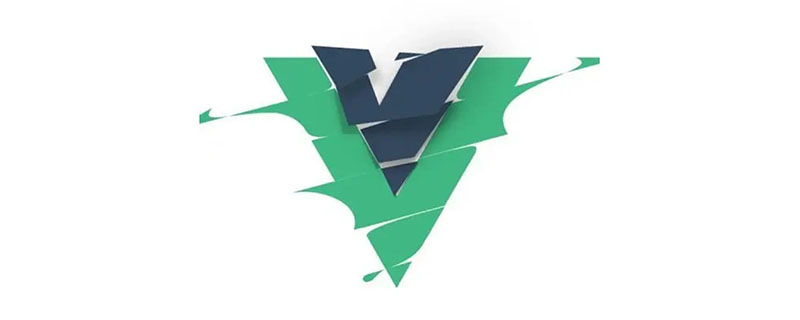
前端有没有现成的库,可以直接用来绘制 Flowable 流程图的?下面本篇文章就跟小伙伴们介绍一下这两个可以绘制 Flowable 流程图的前端库。
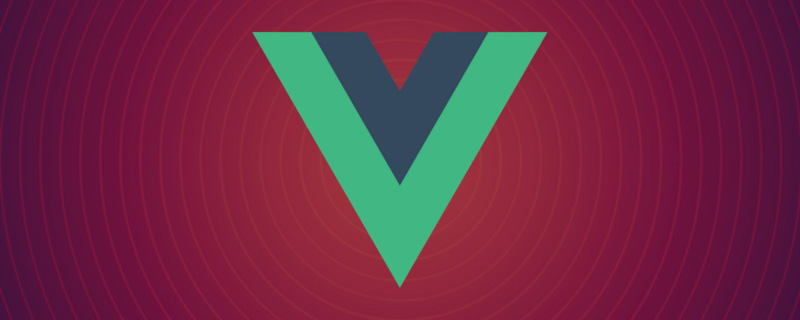
vue不是前端css框架,而是前端JavaScript框架。Vue是一套用于构建用户界面的渐进式JS框架,是基于MVVM设计模式的前端框架,且专注于View层。Vue.js的优点:1、体积小;2、基于虚拟DOM,有更高的运行效率;3、双向数据绑定,让开发者不用再去操作DOM对象,把更多的精力投入到业务逻辑上;4、生态丰富、学习成本低。
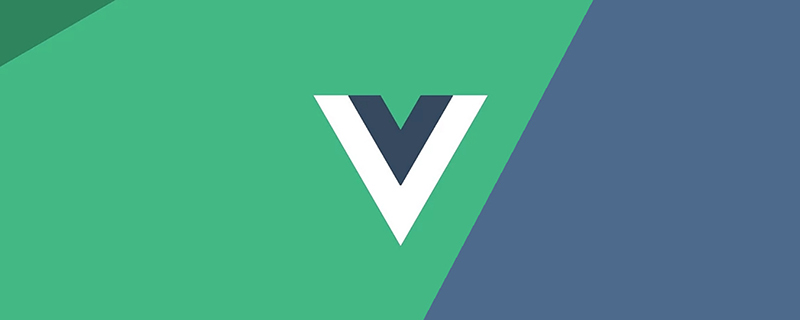
Vue3如何更好地使用qrcodejs生成二维码并添加文字描述?下面本篇文章给大家介绍一下Vue3+qrcodejs生成二维码并添加文字描述,希望对大家有所帮助。
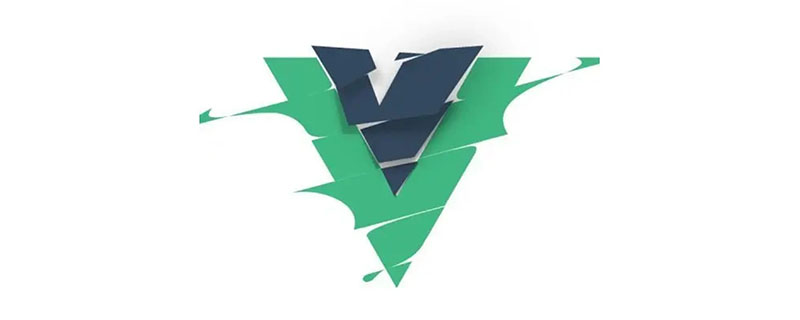
本篇文章我们来了解 Vue2.X 响应式原理,然后我们来实现一个 vue 响应式原理(写的内容简单)实现步骤和注释写的很清晰,大家有兴趣可以耐心观看,希望对大家有所帮助!


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
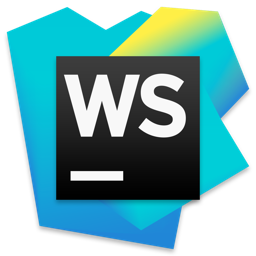
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
