Computed properties
We know that some data in data can be displayed directly through interpolation syntax in the template, but in some cases, we may need to perform some transformations on the data before displaying it, or we need to Combining multiple data for display
Using expressions in templates can be very convenient, but they are originally designed for simple operations. Putting too much logic in the template will make the template It is too heavy and difficult to maintain, and if it is used in multiple places, there will be a lot of duplicate code
So we hope to separate the business logic and UI interface. One way is to extract the logic into a method, but this approach has the following disadvantages
All the data usage process will become a method call
Multiple acquisitions Data needs to call the method multiple times to execute the corresponding logic without caching
In fact, For any complex logic that contains responsive data, you should use calculated properties
<div id="app"> <!-- 计算属性的使用和普通状态的使用方式是一致的 --> <h3 id="nbsp-fullname-nbsp">{{ fullname }}</h3> </div> <script> Vue.createApp({ data() { return { firstname: 'Klaus', lastname: 'Wang' } }, computed: { fullname() { return this.firstname + ' ' + this.lastname } } }).mount('#app')
Cache
Computed properties will be cached based on their dependencies. When the data does not change, calculated properties do not need to be recalculated
But if When the dependent data changes, the calculated properties will still be recalculated when used
And the interface will be re-rendered using the latest calculated property values
getters and setters
Computed properties In most cases, only one getter method is needed, so we will write the calculated properties directly as a function
<div id="app"> <!-- 计算属性的使用和普通状态的使用方式是一致的 --> <h3 id="nbsp-fullname-nbsp">{{ fullname }}</h3> <button @click="change">change</button> </div> <script> Vue.createApp({ data() { return { firstname: 'Klaus', lastname: 'Wang' } }, methods: { change() { this.fullname = 'Alex Li' } }, computed: { // 计算属性的完整写法 fullname: { get() { return this.firstname + ' ' + this.lastname }, set(v) { this.firstname = v.split(' ')[0] this.lastname = v.split(' ')[1] } } } }).mount('#app') </script>
Listener
is defined in the object returned by data The data is bound to the template through interpolation syntax, etc. When the data changes, the template will automatically update to display the latest data
But in some cases, we hope that in the code logic To monitor changes in certain data, you need to use the listener watch at this time
Vue.createApp({ data() { return { info: { name: 'Klaus' } } }, watch: { // 可以使用watch监听响应式数据的改变 // 对应有两个参数 // 参数一 --- 新值 // 参数二 --- 旧值 info(newV, oldV) { // 如果监听的值是对象,获取到的新值和旧值是对应对象的代理对象 console.log(newV, oldV) // 代理对象 转 原生对象 // 1. 使用浅拷贝获取一个新的对象,获取的新的对象为原生对象 console.log({...newV}) // 2. 使用Vue.toRaw方法获取原生对象 console.log(Vue.toRaw(newV)) } }, methods: { change() { this.info = { name: 'Steven' } } } }).mount('#app')
Configuration options
Properties | Description |
---|---|
deep | Whether to turn on deep monitoring The value is boolean When it is not turned on, if the object is monitored, only the reference of the object occurs The watch callback will only be triggered when it changes After starting, if the object is monitored, then as long as any attribute in the object changes, the watch callback will be triggered |
immediate | Whether to start monitoring immediately By default, the watch monitoring will not be triggered for the first rendering. Only when the value changes, the watch monitoring will be triggered. After setting immediate to true, the watch monitoring will be triggered for the first time. Rendering will also trigger watch monitoring. At this time, the value of oldValue is undefined |
Vue.createApp({ data() { return { info: { name: 'Klaus' } } }, watch: { info: { // 开启了深度监听后,当info的属性发生改变的时候,就会触发对应的watch回调 // 注意: 和直接修改info引用不同的是,如果直接修改的是对象的属性 // 那么此时newV和oldV是同一个对象的引用, 此时也就获取不到对应的旧值 handler(newV, oldV) { console.log(newV, oldV) console.log(newV === oldV) // => true }, deep: true, immediate: true } }, methods: { change() { this.info.name = 'Steven' } } }).mount('#app')
Other writing methods
Directly monitor the object properties
watch: { 'info.name'(newV, oldV){ console.log(newV, oldV) } }
String writing method
Vue.createApp({ data() { return { info: { name: 'Klaus' } } }, watch: { // watch的值如果是一个字符串的时候 // 会自动以该字符串作为函数名去methods中查找对应的方法 'info.name': 'watchHandler' }, methods: { change() { this.info.name = 'Steven' }, watchHandler(newV, oldV){ console.log(newV, oldV) } } }).mount('#app')
Array writing method
Vue.createApp({ data() { return { info: { name: 'Klaus' } } }, watch: { 'info.name': [ 'watchHandler', function handle() { console.log('handler2') }, { handler() { console.log('handler3') } } ] }, methods: { change() { this.info.name = 'Steven' }, watchHandler(){ console.log('handler1') } } }).mount('#app')
$watch
Vue.createApp({ data() { return { info: { name: 'Klaus' } } }, created() { /* $watch 参数列表 参数一 --- 侦听源 参数二 --- 侦听回调 参数三 --- 配置对象 */ this.$watch('info.name', (newV, oldV) => console.log(newV, oldV), { immediate: true }) }, methods: { change() { this.info.name = 'Steven' } } }).mount('#app')
The above is the detailed content of How to use computed properties and listeners in Vue3. For more information, please follow other related articles on the PHP Chinese website!
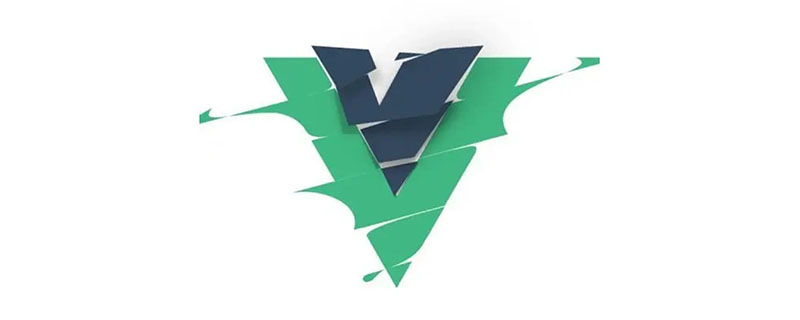
前端有没有现成的库,可以直接用来绘制 Flowable 流程图的?下面本篇文章就跟小伙伴们介绍一下这两个可以绘制 Flowable 流程图的前端库。
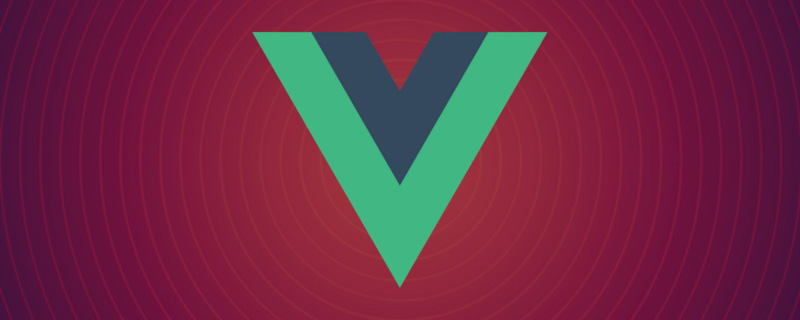
vue不是前端css框架,而是前端JavaScript框架。Vue是一套用于构建用户界面的渐进式JS框架,是基于MVVM设计模式的前端框架,且专注于View层。Vue.js的优点:1、体积小;2、基于虚拟DOM,有更高的运行效率;3、双向数据绑定,让开发者不用再去操作DOM对象,把更多的精力投入到业务逻辑上;4、生态丰富、学习成本低。
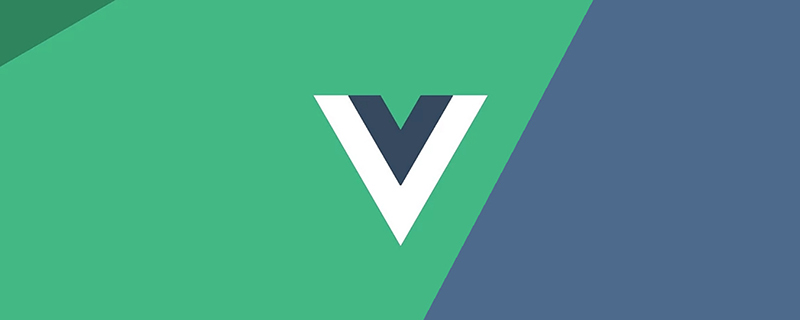
Vue3如何更好地使用qrcodejs生成二维码并添加文字描述?下面本篇文章给大家介绍一下Vue3+qrcodejs生成二维码并添加文字描述,希望对大家有所帮助。
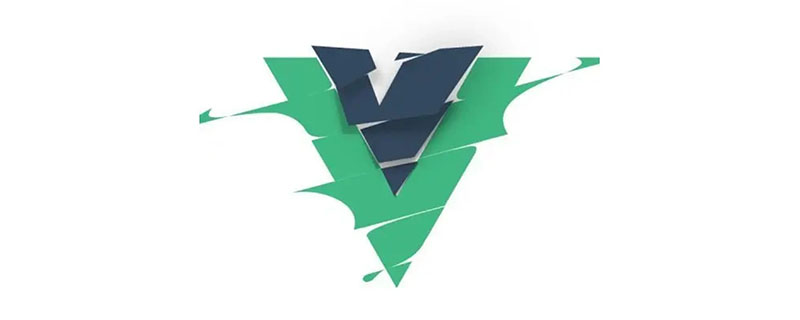
本篇文章我们来了解 Vue2.X 响应式原理,然后我们来实现一个 vue 响应式原理(写的内容简单)实现步骤和注释写的很清晰,大家有兴趣可以耐心观看,希望对大家有所帮助!


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
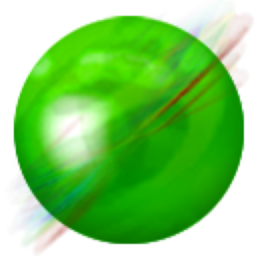
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
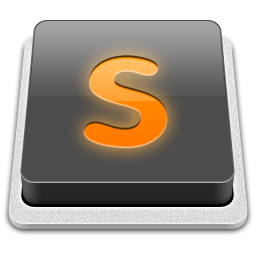
SublimeText3 Mac version
God-level code editing software (SublimeText3)
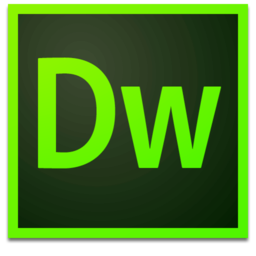
Dreamweaver Mac version
Visual web development tools
