During the development process, we often need to deal with static resources, such as CSS files, JavaScript files, etc. In Golang, these static resources can also be easily processed. This article will introduce how to set up static resources in Golang.
1. What are static resources?
Static resources refer to files that have not been processed and parsed on the server side, such as pictures, CSS, JavaScript and other files. These files can be returned directly through user requests. Parse and render to the browser.
2. Use http.FileServer to set static resources
In Golang, you can use http.FileServer to set static resources. http.FileServer provides a simple method to serve files in a specified file directory to HTTP clients. The specific usage is as follows:
package main import ( "net/http" ) func main() { http.Handle("/", http.FileServer(http.Dir("./public/"))) http.ListenAndServe(":8080", nil) }
In the above code, http.Dir("./public/") indicates the directory where the static resource to be set is located. "/" means providing static resources when setting the access root path. After the setting is completed, you can visit localhost:8080 through the browser to check whether the setting is successful.
3. Use http.StripPrefix to set multiple static resources
If you want to set multiple static resources in the same server, you can use http.StripPrefix to set it. For example, to set static resources in the three directories of js, css, and images, the code is as follows:
package main import ( "net/http" ) func main() { http.Handle("/js/", http.StripPrefix("/js/", http.FileServer(http.Dir("./public/js")))) http.Handle("/css/", http.StripPrefix("/css/", http.FileServer(http.Dir("./public/css")))) http.Handle("/images/", http.StripPrefix("/images/", http.FileServer(http.Dir("./public/images")))) http.ListenAndServe(":8080", nil) }
In the above code, http.StripPrefix is used to safely remove the specified prefix string from the access path. For example, if the access path is "/js/main.js", then http.StripPrefix will remove the "/js/" prefix and then access the "./public/js/main.js" file. In this way, a variety of static resources can be set up.
4. Use custom Handler to set static resources
In addition to using http.FileServer and http.StripPrefix, you can also customize Handler to process static resources. For example:
package main import ( "net/http" ) func main() { http.HandleFunc("/js/", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "./public"+r.URL.Path) }) http.HandleFunc("/css/", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "./public"+r.URL.Path) }) http.HandleFunc("/images/", func(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "./public"+r.URL.Path) }) http.ListenAndServe(":8080", nil) }
In the above code, when accessing the "/js/", "/css/", "/images/" paths, the corresponding Handler will be called and http.ServeFile will be used to provide it. Static resources.
5. Use the third-party library gin to set static resources
If you are using gin in the Golang web framework, you can easily set static resources. For example:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.Static("/js", "./public/js") r.Static("/css", "./public/css") r.Static("/images", "./public/images") r.Run(":8080") }
In the above code, the gin framework is used to set static resources. When accessing the "/js", "/css", and "/images" paths, the corresponding static resources will be provided.
6. Summary
The above is the method of setting static resources in Golang, including using http.FileServer, http.StripPrefix, custom Handler and gin framework, etc. Choosing the appropriate method during development can easily handle static resources and improve development efficiency.
The above is the detailed content of How to set static in golang. For more information, please follow other related articles on the PHP Chinese website!
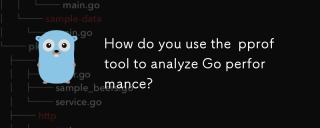
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
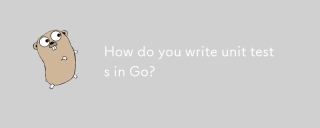
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
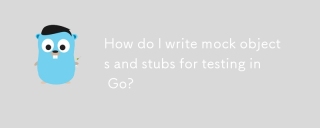
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
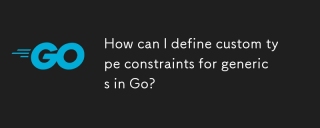
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
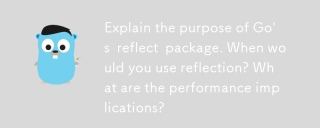
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
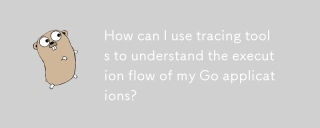
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
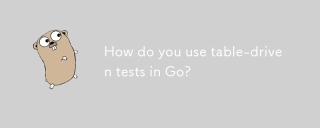
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
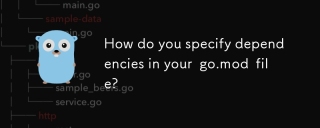
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
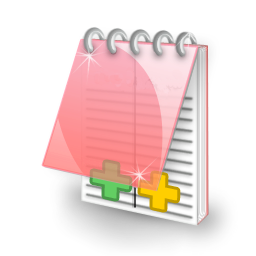
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
