


How to solve the serialization and deserialization problems of Json in SpringBoot
Control json serialization/deserialization
1. Usage of @JsonIgnoreProperties
@JsonIgnoreProperties(value = { "prop1","prop2" })
is used to modify the Pojo class and ignore the specified properties during serialization and deserialization. One or more attributes can be ignored.
@JsonIgnoreProperties(ignoreUnknown = true)
is used to modify the Pojo class and ignore those attributes that cannot be set during deserialization, including those that cannot be set in the constructor and have no corresponding setter methods.
2. @JsonProperty annotation
If the name of the json field is inconsistent with the property name of the Pojo, you can use @JsonProperty to annotate the getter() or setter() method. This annotation sets the json corresponding In addition, @JsonProperty is often used to annotate the formal parameters of the constructor. At this time, the constructor should be annotated with @JsonCreator.
3. @JsonCreator annotation
If the Pojo class definition has Parameter constructor, but if no parameterless constructor is provided, an error will be reported during deserialization. There are two methods:
Method 1: Add a parameterless constructor
Method 2: Add the @JsonCreator annotation to this parameterized constructor, and the parameters need to be annotated with @JsonProperty.
4. @JsonSetter and @JsonGetter annotations
If the name of the json field is inconsistent with the property name of the Pojo, you can use @JsonGetter to annotate the getter() and @JsonSetter to annotate the setter() method. These two Each annotation can specify a property name. Both annotations can be replaced with @JsonProperty.
5. @JsonAnySetter annotation
Generally, the object property names are determined, such as the Car object , there are named attributes such as brand/price, but sometimes we also need to set some extended attributes for the Car object. The names of these extended attributes are not yet determined. Map
public class Car { public String brand; private Map<String, String> properties; @JsonAnySetter public void add(String key, String value) { properties.put(key, value); } }
Once the class is added with @JsonAnySetter, the following json data can be deserialized into the Map:
{ "brand":"Benz", "attr2":"val2", "attr1":"val1" }
6. The @JsonAnyGetter annotation
corresponds to the @JsonAnySetter annotation. If you want to convert the class To serialize the Map K/V attributes into json, you need to add a @JsonAnyGetter method to the class. This method can directly return the KV map.
public class Car { public String brand; private Map<String, String> properties; @JsonAnyGetter public Map<String, String> getProperties() { return properties; } }
The serialized json is:
{ "brand":"Benz", "attr2":"val2", "attr1":"val1" }
7. @JsonFormat annotation
Usually clear the time format used for serialization of Date/Time properties.
public class Event { public String name; @JsonFormat( shape = JsonFormat.Shape.STRING, pattern = "dd-MM-yyyy hh:mm:ss") public Date eventDate; }
8.@JsonSerialize and @JsonDeserialize annotation
@JsonSerialize annotation You can set a special serialization function for class attributes. The @JsonDeserialize annotation is used to customize the deserialization function for json attributes
SpringBoot serialization rules
Include.Include.ALWAYS
DefaultInclude.NON_DEFAULT
The attribute is the default value and is not serializedInclude.NON_EMPTY
If the attribute is empty ("") or NULL, it will not be serialized.Include.NON_NULL
If the attribute is NULL, it will not be serialized.
1. Global settings
/** * 〈返回json空值去掉null和""〉 〈功能详细描述〉 * * @author gogym * @version 2017年10月13日 * @see JacksonConfig * @since */ @Configuration public class JacksonConfig { @Bean @Primary @ConditionalOnMissingBean(ObjectMapper.class) public ObjectMapper jacksonObjectMapper(Jackson2ObjectMapperBuilder builder) { ObjectMapper objectMapper = builder.createXmlMapper(false).build(); // 通过该方法对mapper对象进行设置,所有序列化的对象都将按改规则进行系列化 // Include.Include.ALWAYS 默认 // Include.NON_DEFAULT 属性为默认值不序列化 // Include.NON_EMPTY 属性为 空("") 或者为 NULL 都不序列化,则返回的json是没有这个字段的。这样对移动端会更省流量 // Include.NON_NULL 属性为NULL 不序列化,就是为null的字段不参加序列化 //objectMapper.setSerializationInclusion(Include.NON_EMPTY); objectMapper.setSerializationInclusion(JsonInclude.Include.NON_EMPTY); return objectMapper; } }
or
spring.jackson.default-property-inclusion=non_null spring: jackson: default-property-inclusion: non_null
2. Local settings
on the entity class or field that needs to be set Add annotation
@JsonInclude(Include.NON_NULL)
3. Customize local serialization
(1), customize a serialization tool class, you need to implement StdSerializer
public class ClientObjectSerialize extends JsonSerializer<CreditBorrowerRepaymentRequestDto>{ @Override public void serialize(CreditBorrowerRepaymentRequestDto dto, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException, JsonProcessingException { jsonGenerator.writeStartObject(); try { Field[] fields = dto.getClass().getDeclaredFields(); for (Field field : fields) { field.setAccessible(true); if(null == field.get(dto)){ continue; } jsonGenerator.writeFieldName(field.getName()); jsonGenerator.writeObject(field.get(dto)); } } catch (Exception e) { e.printStackTrace(); } jsonGenerator.writeEndObject(); } }
(2) Use annotations to act on entity classes
@JsonSerialize(using = ClientObjectSerialize.class) public class CreditBorrowerRepaymentRequestDto{ }
(3) Can act on entity object fields, process NULL values, or convert
@JsonSerialize(using = ClientStringSerialize.class) private String name; @JsonSerialize(using = ClientDtaeSerialize.class) private Date date; public class ClientStringSerialize extends JsonSerializer<String> { @Override public void serialize(String string, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { if(string == null){ jsonGenerator.writeString(string + "[NULL]"); }else{ jsonGenerator.writeString(string); } } } public class ClientDtaeSerialize extends JsonSerializer<Date> { @Override public void serialize(Date createDate, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeString(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(createDate)); } }
4. Customization Global null conversion serialization
SpringBoot returns null value processing in Json data, converts string type null value to "", converts collection array type null value to [], converts original data type null value to 0, convert the Boolean type null value to false, and convert the entity object null value to {}.
(1)Custom null value serialization processor
import com.fasterxml.jackson.core.JsonGenerator; import com.fasterxml.jackson.databind.JsonSerializer; import com.fasterxml.jackson.databind.SerializerProvider; import java.io.IOException; /** * 自定义null值序列化处理器 */ public class CustomizeNullJsonSerializer { /** * 处理数组集合类型的null值 */ public static class NullArrayJsonSerializer extends JsonSerializer<Object> { @Override public void serialize(Object value, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeStartArray(); jsonGenerator.writeEndArray(); } } /** * 处理字符串类型的null值 */ public static class NullStringJsonSerializer extends JsonSerializer<Object> { @Override public void serialize(Object value, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeString(""); } } /** * 处理数值类型的null值 */ public static class NullNumberJsonSerializer extends JsonSerializer<Object> { @Override public void serialize(Object value, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeNumber(0); } } /** * 处理boolean类型的null值 */ public static class NullBooleanJsonSerializer extends JsonSerializer<Object> { @Override public void serialize(Object value, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeBoolean(false); } } /** * 处理实体对象类型的null值 */ public static class NullObjectJsonSerializer extends JsonSerializer<Object> { @Override public void serialize(Object value, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeStartObject(); jsonGenerator.writeEndObject(); } } }
(2)Serializer modifier
import com.fasterxml.jackson.databind.BeanDescription; import com.fasterxml.jackson.databind.SerializationConfig; import com.fasterxml.jackson.databind.ser.BeanPropertyWriter; import com.fasterxml.jackson.databind.ser.BeanSerializerModifier; import java.util.Collection; import java.util.List; /** * <pre class="brush:php;toolbar:false"> * 此modifier主要做的事情为: * 1.当序列化类型为数组集合时,当值为null时,序列化成[] * 2.String类型值序列化为"" * **/ public class MyBeanSerializerModifier extends BeanSerializerModifier { @Override public List
(3)Configure Jackson entity
import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.SerializerProvider; import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Primary; import org.springframework.http.converter.json.Jackson2ObjectMapperBuilder; /** * 配置Jackson实体 */ @Configuration public class JacksonConfig { @Bean @Primary @ConditionalOnMissingBean(ObjectMapper.class) public ObjectMapper jacksonObjectMapper(Jackson2ObjectMapperBuilder builder) { ObjectMapper objectMapper = builder.createXmlMapper(false).build(); /** 为objectMapper注册一个带有SerializerModifier的Factory */ objectMapper.setSerializerFactory(objectMapper.getSerializerFactory() .withSerializerModifier(new MyBeanSerializerModifier())); SerializerProvider serializerProvider = objectMapper.getSerializerProvider(); serializerProvider.setNullValueSerializer(new CustomizeNullJsonSerializer .NullObjectJsonSerializer()); return objectMapper; } }
The above is the detailed content of How to solve the serialization and deserialization problems of Json in SpringBoot. For more information, please follow other related articles on the PHP Chinese website!
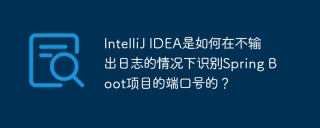
Start Spring using IntelliJIDEAUltimate version...
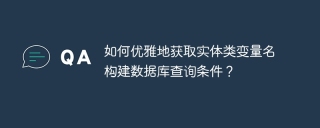
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
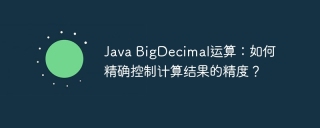
Java...
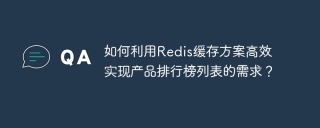
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
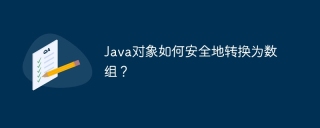
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
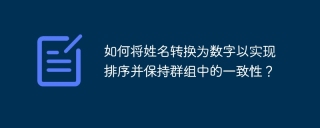
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
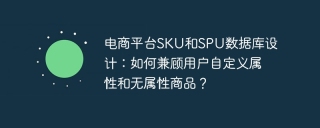
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
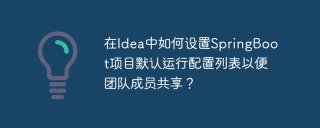
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
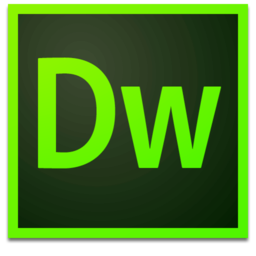
Dreamweaver Mac version
Visual web development tools
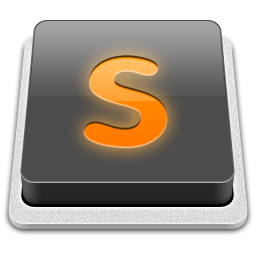
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
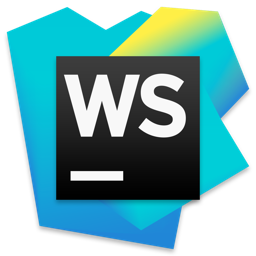
WebStorm Mac version
Useful JavaScript development tools