How to modify the date format in JavaScript?
There are many ways to represent dates in JavaScript, such as date objects and date strings. In actual development, we often need to format the date into a specified form. This article will introduce how to modify the date format using JavaScript.
- Date object
The Date object is a standard object for handling dates and times in JavaScript. We can use it to represent a specific date and time:
let currentDate = new Date(); console.log(currentDate); // 输出当前日期
The output result is:
Fri Oct 15 2021 14:10:30 GMT+0800 (中国标准时间)
- Date formatting
In order to format the date into the specified form, we need to use some methods and properties of the Date object. For example:
- getDate(): Get the day in the date
- getMonth(): Get the month in the date (starting from 0)
- getFullYear(): Get the year in the date
- getHours(): Get the hours in the date (24-hour format)
- getMinutes(): Get the minutes in the date
- getSeconds(): Get the seconds in the date
- toLocaleDateString(): Convert the date to a local time string
- toLocaleTimeString(): Convert the time to a local time string
Based on these methods, we can customize the date formatting method, as follows:
function dateTimeFormatter(date) { const year = date.getFullYear(); const month = (date.getMonth() + 1).toString().padStart(2, '0'); const day = date.getDate().toString().padStart(2, '0'); const hours = date.getHours().toString().padStart(2, '0'); const minutes = date.getMinutes().toString().padStart(2, '0'); const seconds = date.getSeconds().toString().padStart(2, '0'); return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`; } const date = new Date(); console.log(dateTimeFormatter(date)); // 输出自定义格式化日期
The output result is:
2021-10-15 14:24:12
- Date string to date object
If we have a date string and need to convert it to a date object, we can use the parse() method of the Date object:
const dateString = '2021-10-15T14:30:00'; const date = new Date(Date.parse(dateString)); console.log(date);
The output result is:
Fri Oct 15 2021 14:30:00 GMT+0800 (中国标准时间)
- Date object to date string
If we have a date object and need to convert it to a date string, we can use the toISOString() and toLocaleString() methods of the Date object :
const date = new Date(); console.log(date.toISOString()); // 输出 ISO 格式的日期字符串 console.log(date.toLocaleString()); // 输出本地化日期时间字符串
The output results are:
2021-10-15T06:38:27.752Z 2021/10/15 上午6:38:27
- Moment.js library
In addition to using native methods and functions in JavaScript, we also You can use the Moment.js library to handle dates and times. The library provides a rich API and tools to easily solve problems such as date formatting, date calculations, and time zone settings.
Example:
const date = moment(new Date()).format('YYYY-MM-DD HH:mm:ss'); console.log(date);
The output result is consistent with the custom formatted date output:
2021-10-15 15:17:24
Summary:
In JavaScript, we can use The native Date object and some methods and properties are used to handle dates and times. You can also use the Moment.js library to conveniently handle dates and times. Whether using JavaScript or the Moment.js library, we need to understand common date processing methods and related APIs to better handle dates and times.
The above is the detailed content of Modify date format javascript. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

Reactisafrontendlibrary,focusedonbuildinguserinterfaces.ItmanagesUIstateandupdatesefficientlyusingavirtualDOM,andinteractswithbackendservicesviaAPIsfordatahandling,butdoesnotprocessorstoredataitself.

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
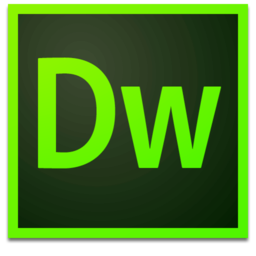
Dreamweaver Mac version
Visual web development tools
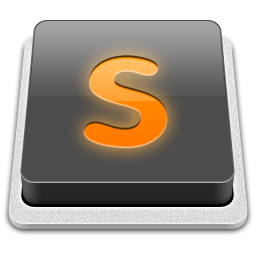
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
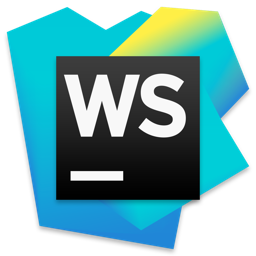
WebStorm Mac version
Useful JavaScript development tools