With the rapid development of the Internet, the demand in the field of web development continues to increase. In order to meet this demand, new development languages and frameworks are constantly emerging. As one of the mainstream languages for Web development, PHP's concepts of classes and objects are becoming more and more important.
Classes and objects in PHP are an important part of programmers developing web applications. The introduction of classes and objects in PHP makes the organization and maintenance of code easier, and can improve the reusability and scalability of programs.
Basic concepts of classes and objects
In PHP, a class is a blueprint composed of properties and methods, used to define specific behaviors and properties of a type of object. An object is the concrete existence of a class after it is instantiated, and can access all properties and methods of the class.
In PHP, the basic syntax of a class is as follows:
class MyClass{ //定义属性 public $my_attribute_1; private $my_attribute_2; protected $my_attribute_3; //定义方法 public function my_method_1(){ //方法体 } private function my_method_2(){ //方法体 } protected function my_method_3(){ //方法体 } }
The above code defines a class named MyClass, which contains three properties and three methods. Properties can be marked as public, private, or protected, and have different visibility when used in a class and its subclasses. Methods can also be marked as public, private or protected, with access restrictions similar to properties.
Using objects
In PHP, you can create an object through the new operator. For example:
$my_object = new MyClass();
The above code creates an object of MyClass class and assigns it to the variable $my_object. The properties and methods of the MyClass class can be accessed through this variable, for example:
//访问属性 $my_object->my_attribute_1; //调用方法 $my_object->my_method_1();
Inheritance and polymorphism
In PHP, inheritance is the process in which one class derives another class. Derived classes inherit the properties and methods of the base class and can add, modify or delete them. For example:
class MyDerivedClass extends MyClass{ //添加属性 public $my_derived_attribute; //修改方法 public function my_method_1(){ //覆盖父类方法 } //添加方法 public function my_derived_method(){ //新方法 } }
The above code defines a MyDerivedClass class, which inherits from the MyClass class. Added an attribute to the MyDerivedClass class, overridden the my_method_1() method in the MyClass class, and added a new method my_derived_method(). These changes only affect the MyDerivedClass class, not the MyClass class.
Polymorphism means that objects of different classes can respond differently to the same method. In PHP, the same method can be implemented by multiple classes, for example:
class ClassA{ public function my_method(){ //方法体 } } class ClassB{ public function my_method(){ //方法体 } } $object_a = new ClassA(); $object_b = new ClassB(); //调用ClassA和ClassB中的my_method()方法 $object_a->my_method(); $object_b->my_method();
The above code creates objects of two classes, ClassA and ClassB, both of which implement a method named my_method(). Through these objects, their respective my_method() methods can be called, achieving a polymorphic effect.
Summary
Classes and objects in PHP are an indispensable and important part of developing web applications. Through classes and objects, code can be better organized and maintained, and the reusability and extensibility of the program can be improved. Inheritance and polymorphism of classes and objects make it easier for programmers to reuse and extend code. Therefore, mastering classes and objects in PHP is one of the basic skills that every web developer must have.
The above is the detailed content of Classes and Objects in PHP. For more information, please follow other related articles on the PHP Chinese website!
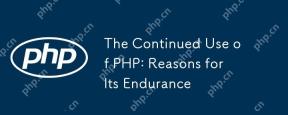
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
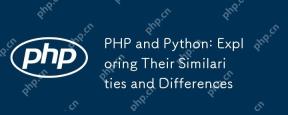
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
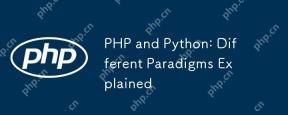
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
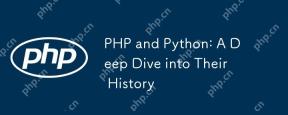
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
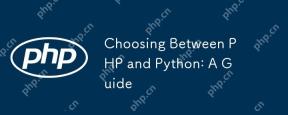
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
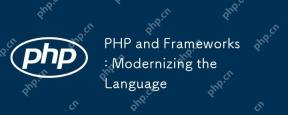
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
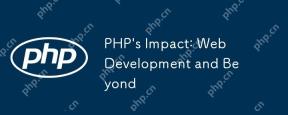
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
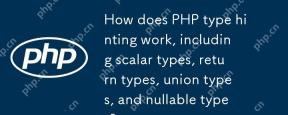
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
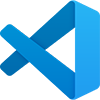
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
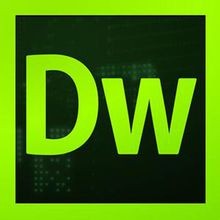
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.