Golang is a popular programming language. Its built-in integer division operator directly truncates the result to the integer part, which may be difficult for beginners. So how to get decimal after integer division in Golang? This article will discuss Golang integer division and decimal operations and provide practical solutions.
Golang integer division
In Golang, the integer division operator is "/", for example, the result of 5/2 operation is 2. This integer truncation behavior is determined by Golang's type system, because the divisor and dividend are both integer types, so the result must also be of integer type.
If you want to get the decimal part, you can convert one of the operands to a floating point type, such as 5.0/2, in which case the result is 2.5.
Another solution is to use "float64" type conversion, such as 5/float64(2), which can also get the result of 2.5.
Golang decimal operation
In Golang, the default type of decimal operation is the "float64" type. Therefore, for addition, subtraction, multiplication and division of two decimals, you can directly use the " ", "-", "*" and "/" operators. For example:
var a, b, c, d float64 a = 5.0 b = 2.5 c = a + b // 结果为7.5 d = a * b // 结果为12.5
It should be noted that since floating point operations have precision issues, you need to pay attention to precision issues when performing decimal operations. For example, the following operation results:
var a, b, c float64 a = 0.1 b = 0.2 c = a + b // 结果为0.30000000000000004
When doing complex decimal operations, you need to use Golang's built-in "math" package, which contains some commonly used decimal calculation functions, such as:
import "math" var a float64 a = math.Sin(1.0) // 求sin(1),结果为0.8414709848078965
Customized decimal division function
In the actual process of writing code, we sometimes need to encapsulate some functions to facilitate the use of ourselves or others. Therefore, we can write a function to implement the decimal part calculation of integer division. For example:
func Divide(a, b int) float64 { return float64(a) / float64(b) }
The above function takes "a" and "b" of integer type as input and converts them to floating point type for division operation. The function then returns a result of type "float64".
Through the above measures, integer division in Golang can also achieve decimal results.
Conclusion
In Golang, using the integer division operator directly will cause the result to be directly truncated to an integer, which may increase programming difficulty. However, this problem can be solved by converting one of the operands to a floating point type, or using a type conversion function. In addition, when performing decimal operations, you need to pay attention to the accuracy of floating point numbers. Writing a custom decimal division function is also a good solution to make the code cleaner and easier to understand.
The above content covers the basic knowledge and practical solutions of integer division and decimal operations in Golang. Hope this article is helpful to you.
The above is the detailed content of golang integer division decimal. For more information, please follow other related articles on the PHP Chinese website!
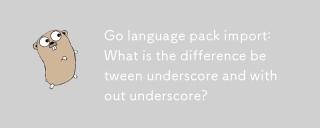
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
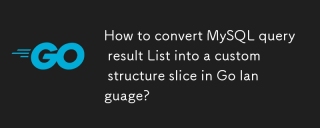
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
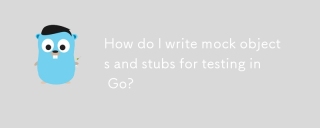
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
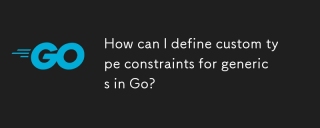
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
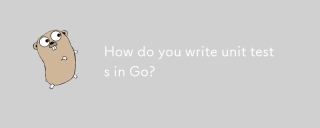
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
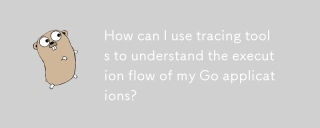
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
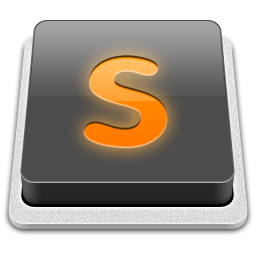
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
