In golang, interface and structure are two common data types. An interface is an abstract type that defines a set of methods without implementation, while a structure is a concrete type that is used to organize and store data. In actual development, we may need to convert the interface into a structure. This article will introduce two methods for converting interfaces into structures in golang.
1. Use reflection to convert the interface into a structure
In golang, you can use reflection to complete the operation of converting the interface into a structure. Reflection is a powerful mechanism that can dynamically read and modify information such as the value, type, and attributes of a variable at runtime. In reflection, commonly used ones include reflect.Value and reflect.Type.
The specific implementation steps are as follows:
- Create a structure type to store the data in the interface. For example:
type Person struct { Name string Age int }
- Create an interface type to convert data into a structure. For example:
type PersonInterface interface { GetName() string GetAge() int }
- Implement a function to convert the interface type to the structure type. For example:
func ConvertInterfaceToStruct(p PersonInterface) (Person, error) { var person Person value := reflect.ValueOf(p) if value.Kind() == reflect.Ptr && !value.IsNil() { value = value.Elem() if value.Kind() == reflect.Struct { person.Name = value.FieldByName("Name").String() person.Age = int(value.FieldByName("Age").Int()) return person, nil } } return person, fmt.Errorf("invalid type:%v", reflect.TypeOf(p)) }
In this function, first convert the interface type to a reflection value (reflect.Value), and then determine whether the value is a pointer type and non-null. Next, the pointer type is converted to a structure type, and then the Name and Age field values are obtained through reflection, and finally the Person structure type is returned.
- Test in the main function. For example:
func main() { p := &Person{Name: "Tom", Age: 20} fmt.Println(p) if ps, err := ConvertInterfaceToStruct(p); err != nil { log.Fatal(err) } else { fmt.Println(ps) } }
In this test code, create a Person structure pointer, use the implemented ConvertInterfaceToStruct function to convert the pointer type to the Person structure type, and output the result.
2. Use json to implement the interface and convert it into a structure
In golang, json is a common data format. You can serialize an object into a json string through json.Marshal(). You can also deserialize a json string into an object through json.Unmarshal().
The specific implementation steps are as follows:
- Create a structure type to store the data in the interface. For example:
type Person struct { Name string `json:"name,omitempty"` Age int `json:"age,omitempty"` }
In this structure, the json field name is specified to match its field name in the interface in order to achieve conversion.
- Create an interface type for converting data into a structure. For example:
type PersonInterface interface { GetName() string GetAge() int }
- Implement a function to convert the interface type to a structure type. For example:
func ConvertInterfaceToStruct(p PersonInterface) (Person, error) { jsonStr, err := json.Marshal(p) if err != nil { return Person{}, err } var person Person err = json.Unmarshal(jsonStr, &person) if err != nil { return Person{}, err } return person, nil }
In this function, first use json.Marshal() to convert the interface type into a json string, then use json.Unmarshal() to convert it into a Person structure type, and Return the structure.
- Test in the main function. For example:
func main() { p := &Person{Name: "Tom", Age: 20} fmt.Println(p) if ps, err := ConvertInterfaceToStruct(p); err != nil { log.Fatal(err) } else { fmt.Println(ps) } }
In this test code, create a Person structure pointer, use the implemented ConvertInterfaceToStruct function to convert the pointer type to the Person structure type, and output the result.
Summary:
Both of the above two methods can convert the interface type into a structure type, using different methods for implementation. The implementation of the reflection method is relatively low-level and requires type judgment. And field reflection reading, etc., while the json method is relatively simple and clear, you only need to use the json serialization and deserialization methods in golang. The choice of different methods depends on factors such as actual needs and performance.
The above is the detailed content of golang interface to struct. For more information, please follow other related articles on the PHP Chinese website!
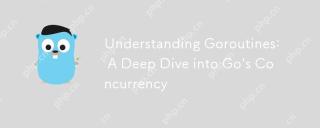
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
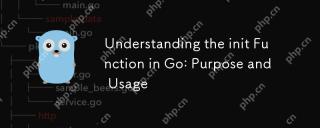
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
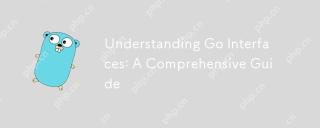
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
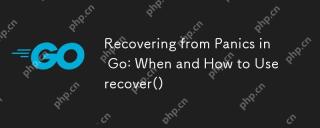
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
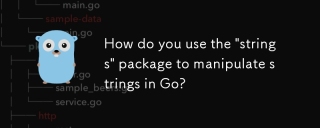
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
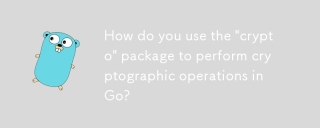
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
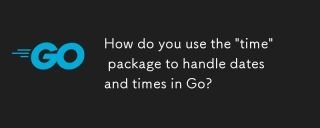
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
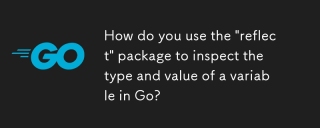
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
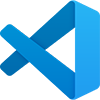
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
