Quick Sort
Quick sort is a relatively efficient sorting algorithm. It adopts the idea of "divide and conquer" to achieve sorting through multiple comparisons and exchanges. In one sorting pass, the data to be sorted is divided into Two independent parts, sort the two parts so that all the data in one part is smaller than the other part, and then continue to recursively sort the two parts, and finally achieve all data in order.
public static void quickSort(int[] arry,int left,int right){ //运行判断,如果左边索引大于右边是不合法的,直接return结束此方法 if(left>right){ return; } //定义变量保存基准数(第一次进入方法最左边的数字下标为0) int base = arry[left]; //定义变量i,指向最左边 int i = left; //定义j ,指向最右边(第一次进入方法最右边数字下标为数组的长度减1) int j = right; //当i和j不相遇的时候,再循环中进行检索 while(i!=j){ //先由j从右往左检索比基准数小的,如果检索到比基准数小的就停下。 //如果检索到比基准数大的或者相等的就停下 while(arry[j]>=base && i<j){ j--; //j从右往左检索 } while(arry[i]<=base && i<j){ i++; //i从左往右检索 } //代码走到这里i停下,j也停下,然后交换i和j位置的元素 int tem = arry[i]; arry[i] = arry[j]; arry[j] = tem; } //如果上面while条件不成立就会跳出这个循环,往下执行 //如果这个条件不成立就说明 i和j相遇了 //如果i和j相遇了,就交换基准数这个元素和相遇位置的元素 //把相遇元素的值赋给基准数这个位置的元素 arry[left] = arry[i]; //把基准数赋给相遇位置的元素 arry[i] = base; //基准数在这里递归就为了左边的数比它小,右边的数比它大 //排序基准数的左边 quickSort(arry,left,i-1); //排右边 quickSort(arry,j+1,right); } public static void main(String[] args) { int[] arry = {11,81,71,61,10,42,33,24,99}; System.out.println("arry排序前:"+Arrays.toString(arry)); quickSort(arry,0, arry.length-1); System.out.println("arry排序后:"+Arrays.toString(arry)); }
Bubble sort
Bubble sort idea: Given an array, sort the array in ascending (descending) order.
Compare adjacent elements in the array from front to back. If the previous element is larger than the next element, swap . The largest element after one trip Right at the end of the array.
Follow the above process until all elements in the array are arranged.
public static void main(String[] args) { int[] arr = {18,13,50,15,4,17,18}; System.out.println("arr的排序前:\n18 13 50 15 4 17 18 "); int temp = 0 ; for(int i = 0 ;i< arr.length -1; i++){ for(int j = 0; j<arr.length-1-i; j++){ if(arr[j]>arr[j+1]){ //条件成立 交换位置 temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } } } System.out.println("arr排序后:"); for(int i = 0; i<arr.length; i++){ System.out.print(arr[i]+"\t"); } }
The above is the detailed content of How to sort arrays from small to large in JAVA. For more information, please follow other related articles on the PHP Chinese website!
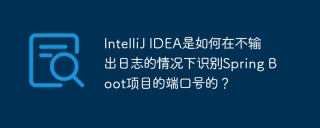
Start Spring using IntelliJIDEAUltimate version...
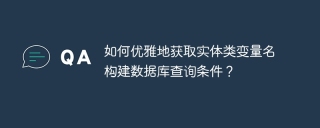
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
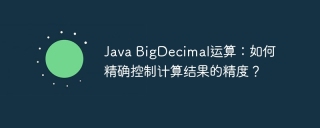
Java...
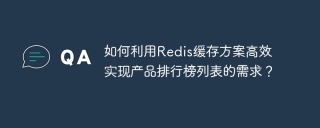
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
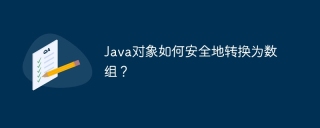
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
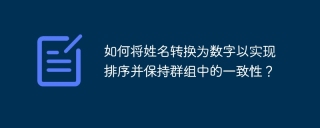
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
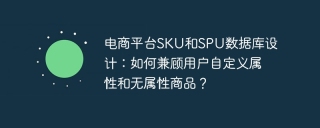
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
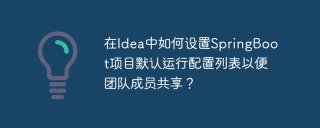
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
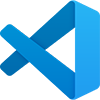
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software