In PHP, we can use the in_array() function to determine whether a given value exists in an array.
Function prototype: in_array($value, $array);
This function receives two parameters. $value represents the value to be found, and $array represents the array to be found.
If the $value value exists in the $array array, the in_array() function will return true; otherwise, it will return false.
For example:
//定义一个数组 $fruits = array('apple', 'banana', 'orange'); //判断 'apple' 是否在数组中 if(in_array('apple', $fruits)){ echo "'apple' 在数组中"; }else{ echo "'apple' 不在数组中"; }
Running the above code will output "'apple' in the array".
In addition to using the in_array() function, we can also use the array_search() function to determine whether a given value exists in the array.
Function prototype: array_search($value, $array);
This function also receives two parameters. $value represents the value to be found, and $array represents the array to be found.
If the $value value exists in the $array array, the array_search() function will return the key name of the value in the array; otherwise, it will return false.
For example:
//定义一个数组 $fruits = array('apple', 'banana', 'orange'); //判断 'banana' 是否在数组中 $key = array_search('banana', $fruits); if($key !== false){ echo "'banana' 在数组中,键名为:" . $key; }else{ echo "'banana' 不在数组中"; }
Running the above code will output "'banana' in the array, key name: 1".
It should be noted that the above two functions use weak type comparison (that is, using the == operator instead of the === operator) when determining whether the value is in the array. If you want to use strict type comparison, you can convert both $value and the elements in the array to the appropriate type before comparison.
For example:
//定义一个数组 $nums = array(1, 2, 3); //判断 '2' 是否在数组中 if(in_array('2', $nums, true)){ //第三个参数指定为 true,表示使用严格类型比较 echo "'2' 在数组中"; }else{ echo "'2' 不在数组中"; }
Running the above code will output "'2' is not in the array".
In addition to the above two functions, there are many other array processing functions in PHP. Proficiency in these functions allows us to deal with array-related issues more conveniently.
The above is the detailed content of PHP determines whether the value is in the array. For more information, please follow other related articles on the PHP Chinese website!
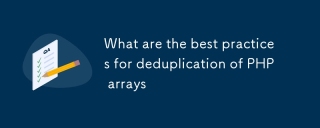
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
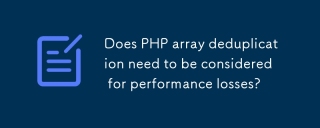
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
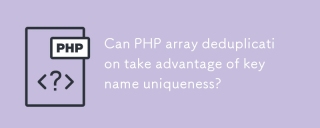
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
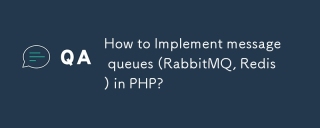
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
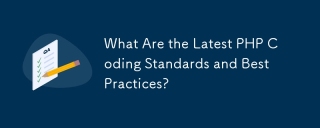
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
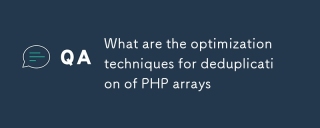
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
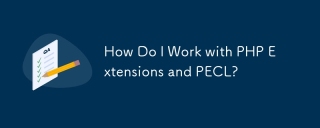
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
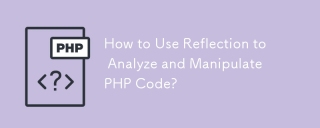
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
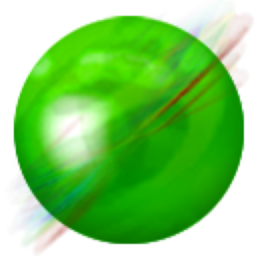
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
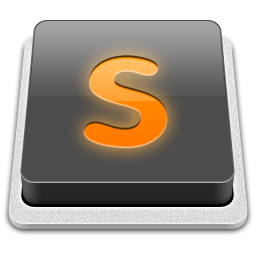
SublimeText3 Mac version
God-level code editing software (SublimeText3)
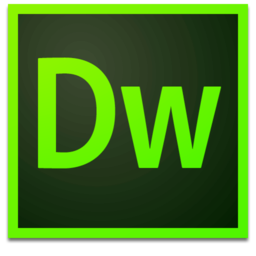
Dreamweaver Mac version
Visual web development tools
