In PHP, objects and arrays are very important data types, and they have their own characteristics and uses. During the development process, we often need to convert objects into arrays to facilitate data transmission and processing. This article will introduce in detail the conversion methods between PHP objects and arrays and their precautions.
1. The basic concept of PHP objects
In PHP, an object is the basis of object-oriented programming. It is an entity instantiated from a class and can be manipulated through methods and properties. it. The following is the basic syntax for creating an object:
class MyClass { public $name; public function sayHello() { echo "Hello, my name is " . $this->name; } } $obj = new MyClass(); $obj->name = "PHP"; $obj->sayHello();
In the above code, we first define a MyClass class, which has a public property $name and a public method sayHello, and then we create a MyClass object $obj, and assign its attribute $name to "PHP", and finally call the sayHello method of $obj to output the result.
2. Method of converting PHP object to array
Converting object to array is a relatively common operation. PHP provides two methods: forced type conversion and serialization (serialize).
- Forced type conversion
We can convert objects to arrays through (array) or get_object_vars(). Their principle is to convert the attributes in the object into the corresponding Array of key-value pairs.
class MyClass { public $name = "PHP"; private $age = 20; } $obj = new MyClass(); $arr = (array) $obj; var_dump($arr); //输出:array(2) { ["name"]=> string(3) "PHP" ["age"]=> int(20) } $obj = new MyClass(); $arr = get_object_vars($obj); var_dump($arr); //输出:array(1) { ["name"]=> string(3) "PHP" }
In the above code, we first define a MyClass class, which has a public attribute $name and a private attribute $age. Then we convert this object through (array) and get_object_vars() respectively. are arrays, and finally output their results.
It should be noted that when using forced type conversion, the value of the private attribute cannot be obtained, and only the public attribute can be converted successfully. If we need to convert private properties into arrays, we need to use ReflectionClass.
- Serialization
In PHP, serialization (serialize) refers to the process of converting an object or array into a string, while deserialization (unserialize) is Convert the string into the original object or array. We can implement serialization and deserialization through the serialize() and unserialize() functions.
class MyClass { public $name = "PHP"; private $age = 20; } $obj = new MyClass(); $str = serialize($obj); //序列化对象 $arr = unserialize($str); //反序列化数组 var_dump($arr); //输出:object(MyClass)#2 (2) { ["name"]=> string(3) "PHP" ["age":"MyClass":private]=> int(20) }
In the above code, we first define a MyClass class, which has a public property $name and a private property $age, then we serialize the $obj object into a string $str, and then Then deserialize to get the array $arr and output the result.
It should be noted that when using serialization, the value of the private attribute can also be obtained, but when deserializing, you need to ensure that the class definition of the original object exists, otherwise unserialize(data): undefined class will appear Error with constant 'xxxx'.
3. Method of converting PHP array to object
In addition to converting objects to arrays, it is often necessary to convert arrays to objects. In PHP, we can achieve this through cast or json_decode() function.
- Forced type conversion
To convert an array into an object, you only need to assign the array to the object. The attribute name of the object is the key name of the array, and the attribute value is the key value of the array.
class MyClass {} $arr = array('name' =>'PHP', 'age' =>20); $obj = (object) $arr; var_dump($obj); //输出:object(stdClass)#1 (2) { ["name"]=> string(3) "PHP" ["age"]=> int(20) }
In the above code, we first create an empty MyClass class, then define an array $arr, which contains two key-value pairs of 'name' and 'age', and then pass it through ( object) is cast to object $obj and outputs the result.
- json_decode()
We can also use the json_decode() function to convert the array into an object, and the implementation method is also very simple. Convert the array into a JSON string using the json_encode() function, and then use the json_decode() function to convert the JSON string into an object.
class MyClass {} $arr = array('name' =>'PHP', 'age' =>20); $json = json_encode($arr); $obj = json_decode($json); var_dump($obj); //输出:object(stdClass)#1 (2) { ["name"]=> string(3) "PHP" ["age"]=> int(20) }
In the above code, we first create an empty MyClass class, then define an array $arr, which contains two key-value pairs of 'name' and 'age', and then pass it through json_encode () function into a JSON string $json, then use the json_decode() function to convert the JSON string into an object $obj, and output the result. It should be noted that json_decode() returns a PHP object by default instead of an array.
4. Some points that need attention
Although the conversion method between objects and arrays is very simple, there are still some things that need to be paid attention to during use.
- Private properties cannot be obtained through forced type conversion
When the object is converted into an array, the value of the private property cannot be obtained. You need to use ReflectionClass to obtain it. Otherwise, the error "Private property cannot be accessed in..." will appear.
- Serialization is a resource-consuming process
Although serialization is convenient and simple, when processing large amounts of data, serialization will occupy a lot of CPU and memory, so you need to pay attention to its consumption.
- json_decode() may return false
When an error occurs in parsing the JSON string, the json_decode() function may return false. If you need to use its results, you need to pay attention. Add error handling.
In short, conversion between objects and arrays is very commonly used in PHP, and can be achieved through forced type conversion and serialization. When application development requires frequent use of objects and arrays, mastering these skills will greatly improve work efficiency.
The above is the detailed content of How to convert php object to array. For more information, please follow other related articles on the PHP Chinese website!
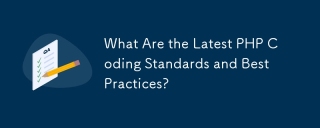
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
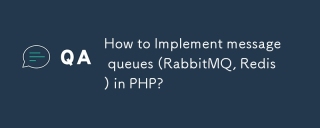
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
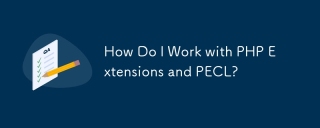
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
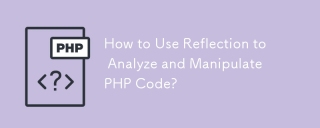
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
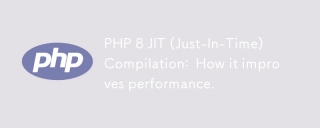
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
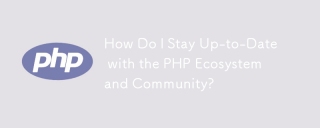
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
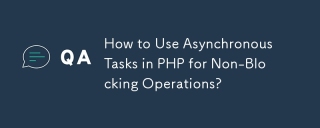
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
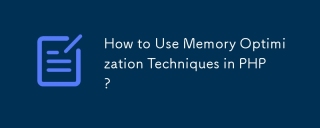
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
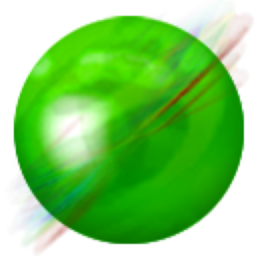
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
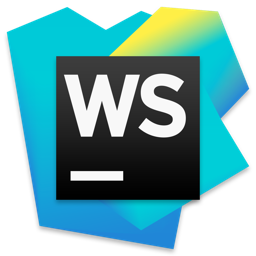
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
