In computer science, a prime number refers to a positive integer that is only divisible by 1 and itself. Prime numbers can be used in fields such as encryption, mathematical derivation, and algorithm optimization. In practical applications, the algorithm for finding prime numbers is also one of the very important knowledge points. Today we will discuss how to use scripts in PHP to find prime numbers.
- Screening method
The screening method is a classic algorithm for finding prime numbers. Its core idea is to continuously filter out numbers that are not prime numbers, and what is left in the end is a prime number. The specific steps are as follows:
- Initialize a prime array $prime = array(), and put numbers from 2 to n (n is the required range) into it.
- For the number 2~sqrt(n) (sqrt(n) represents the square root of n), determine whether it is a prime number in turn. If so, remove its multiples from the prime number array.
- After the loop ends, the remaining numbers in the prime array are all the prime numbers.
The implementation code is as follows:
function sieve($n) { $prime = array(); for($i = 2; $i <ol start="2"><li>Fermat’s Little Theorem</li></ol><p>Fermat’s Little Theorem is an important number theory theorem that can be used Determine whether a number is prime. Fermat's Little Theorem is expressed as follows: If p is a prime number and a is any integer, then a^(p-1)≡1(mod p). </p><p>The specific steps are as follows: </p><ol> <li> Randomly select a number a and determine whether a and n are mutually prime. If they are not mutually prime, return false directly. </li> <li>Calculate the value of a^(n-1) mod n. If it is not equal to 1, return false. </li> <li>After many tests, if the above two conditions are met, then n is likely to be a prime number. </li> </ol><p>The implementation code is as follows: </p><pre class="brush:php;toolbar:false">function is_prime($n) { if($n 0) { if($exp % 2 == 1) { $result = ($result * $base) % $modulus; } $exp = $exp >> 1; $base = ($base * $base) % $modulus; } return $result; }
The above are two methods of finding prime numbers using scripts in PHP. It should be noted that the screening method is often more efficient than Fermat's Little Theorem when solving a large range of prime numbers.
The above is the detailed content of Use script to find prime numbers in php. For more information, please follow other related articles on the PHP Chinese website!
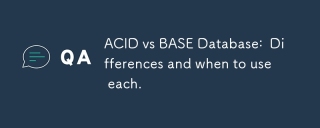
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
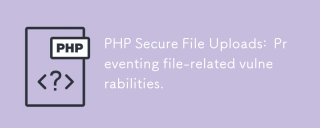
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
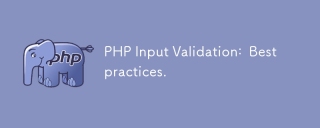
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
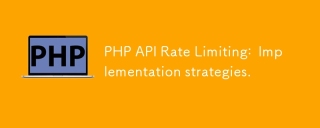
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
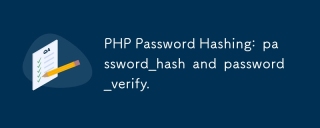
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
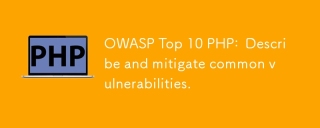
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
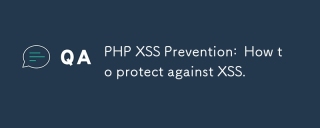
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
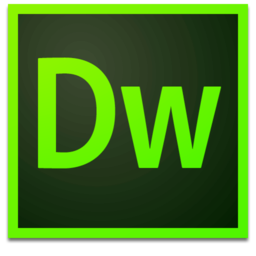
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.