Use stack
package net.javaguides.corejava.string; import java.util.Stack; /** * * @author Ramesh Fadatare * */ public class ReverseStringUsingStack { // Function to reverse a string in Java using a stack and character array public static String reverse(String str) { // base case: if string is null or empty if (str == null || str.equals("")) return str; // create an empty stack of characters Stack < Character > stack = new Stack < Character > (); // push every character of the given string into the stack char[] ch = str.toCharArray(); for (int i = 0; i < str.length(); i++) stack.push(ch[i]); // start from index 0 int k = 0; // pop characters from the stack until it is empty while (!stack.isEmpty()) { // assign each popped character back to the character array ch[k++] = stack.pop(); } // convert the character array into string and return it return String.copyValueOf(ch); } public static void main(String[] args) { String str = "javaguides"; str = reverse(str); // string is immutable System.out.println("Reverse of the given string is : " + str); } }
Output:
Reverse of the given string is : sediugavaj
What are the basic data types of java
The basic data types of Java are divided into: 1. Integer type, used to represent the data type of integers. 2. Floating point type, a data type used to represent decimals. 3. Character type. The keyword of character type is "char". 4. Boolean type is the basic data type that represents logical values.
The above is the detailed content of How to use stack to reverse string in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
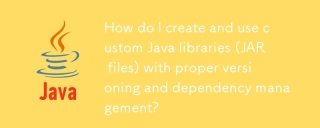
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
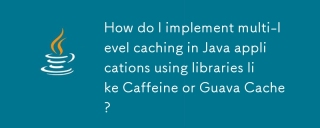
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
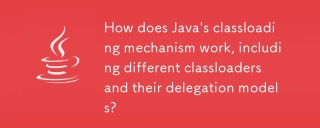
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
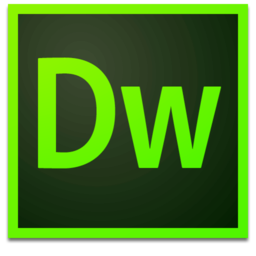
Dreamweaver Mac version
Visual web development tools
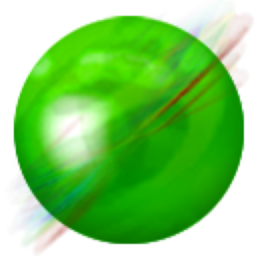
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment