1. Proxy mode
When we need to call a method of a certain class (specific implementation class), we do not directly create an object of that class, but get the proxy class object of the class and use it through the proxy Object, calling the function of the specific implementation class. Both the concrete implementation class and the proxy class implement the same interface, and the proxy class holds the object of the implementation class. This creates a layer of isolation between the calling end and the specific implementation end to avoid direct interaction.
There are many similar examples of the agency model in reality. For example, when we buy or rent a house, we have to go through an intermediary. This intermediary is equivalent to an agent.
2. Static proxy implementation
1) Define interface:
public interface IHouse { void sallHouse(); int sallHouse2(); }
2) Specific implementation class:
public class Andy implements IHouse { @Override public void sallHouse() { System.out.println("andy sall house.."); } @Override public int sallHouse2() { return 100; } }
3) Proxy class:
public class HouseProxy implements IHouse { Andy andy; public HouseProxy(Andy andy) { this.andy = andy; } @Override public void sallHouse() { andy.sallHouse(); } @Override public int sallHouse2() { return andy.sallHouse2(); } }
4) Client call:
//1.创建被代理对象 Andy andy = new Andy(); //2.创建代理对象,代理对象持有被代理对象的引用 HouseProxy proxy = new HouseProxy(andy); //3.客户端通过代理对象调用。 proxy.sallHouse();
3. Dynamic proxy implementation
//1.被代理对象 final Andy andy = new Andy(); //2.创建动态代理,Java在运行时动态生成的。 ClassLoader classLoader = andy.getClass().getClassLoader(); Class[] interfaces = andy.getClass().getInterfaces(); IHouse iHouse = (IHouse) Proxy.newProxyInstance(classLoader, interfaces, new InvocationHandler() { @Override public Object invoke(Object o, Method method, Object[] objects) throws Throwable { //通过反射调用被代理对象的方法 return method.invoke(andy, objects); } }); //3.客户端通过代理对象调用被代理方法。 iHouse.sallHouse();
Dynamic proxy analysis:
1) IHouse iHouse = (IHouse) Proxy.newProxyInstance();
Create a dynamic proxy object,
has three parameters:
1.ClassLoader class loader
2.Class class of the proxy interface ,
3.InvocationHandler interface implementation class
2) After getting the iHouse dynamic proxy, call the interface method iHouse.sallHouse();
As soon as this method is called, The invoke method in the InvocationHandler class will be executed.
@Override
public Object invoke(Object o, Method method, Object[] objects) throws Throwable {
//Invoke the proxy object through reflection Method
return method.invoke(andy, objects);
}
The invoke method has three parameters:
method, which is the proxy class The called method name (sallHouse)
objects is the parameter passed when the proxy class calls the method.
Object obj = method.invoke(andy, objects);
Invoke the andy object through the reflection mechanism, the corresponding method in the specific implementer.
His return value can be received when the proxy object calls the interface. Whatever type it is, what type is returned. This is what Retrofit does
The above is the detailed content of What is Java dynamic proxy and how to implement it. For more information, please follow other related articles on the PHP Chinese website!
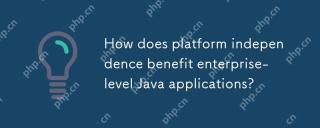
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
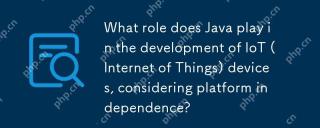
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
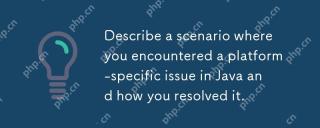
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
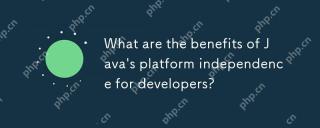
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
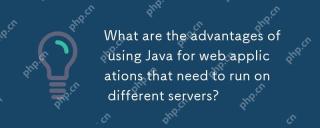
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
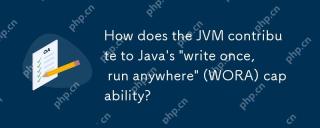
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
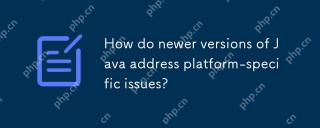
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
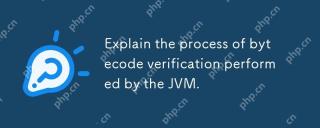
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
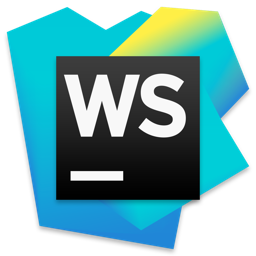
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
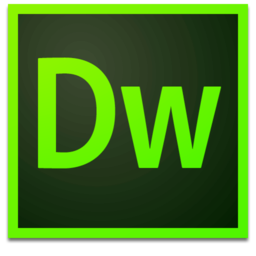
Dreamweaver Mac version
Visual web development tools
