FTP Introduction
FTP is the File Transfer Protocol (File Transfer Protocol), which is a standard protocol for file transfer on the network. FTP client can upload files from local to server or download from server to local.
ftplib module
Python provides a standard library ftplib for implementing FTP client functions in Python. Using ftplib, we can connect to the FTP server and perform various FTP operations, such as uploading and downloading files, etc.
Code explanation
The following is a sample code for uploading files through FTP using Python:
from ftplib import FTP import argparse def ftpconnect(host, username, password): ftp = FTP() ftp.connect(host, 21) ftp.login(username, password) return ftp #从本地上传文件到ftp def uploadfile(ftp, remotepath, localpath): bufsize = 1024 fp = open(localpath, 'rb') ftp.storbinary('STOR ' + remotepath, fp, bufsize) ftp.set_debuglevel(0) fp.close() if __name__ == "__main__": parser = argparse.ArgumentParser() parser.add_argument('--ip', type=str, default = None) parser.add_argument('--user', type=str, default = None) parser.add_argument('--password', type=str, default = None) parser.add_argument('--localFileName', type=str, default = None) parser.add_argument('--fileName', type=str, default = None) args = parser.parse_args() ftp = ftpconnect(args.ip,args.user ,args.password) uploadfile(ftp, "/home/"+args.fileName, args.localFileName) ftp.quit()
First, we import the ftplib module and argparse module.
Next, a ftpconnect function is defined to connect to the FTP server. This function requires 3 parameters: host (FTP server IP address or domain name), username (username), password (password). The function returns an FTP object.
Next, an uploadfile function is defined, which is used to upload files from the local to the FTP server. This function requires 3 parameters: ftp (FTP object), remotepath (path uploaded to FTP server) and localpath (local file path). The function opens a local file, uploads the file using the storbinary method of the FTP object, and finally closes the local file and FTP connection.
Finally, use the argparse module to parse command line parameters. The command line parameters include the IP address of the FTP server, user name, password, local file path, and path to upload to the FTP server. In the main function, first call the ftpconnect function to connect to the FTP server, then call the uploadfile function to upload the file, and finally close the FTP connection.
Run results
We can save the above code as ftp_upload.py and execute the following command in the command line:
python ftp_upload.py --ip 192.168.1.100 --user ftpuser --password ftppass --localFileName localfile.txt --fileName remotefile.txt
where 192.168.1.100 is the FTP server IP address, ftpuser is the FTP username, ftppass is the FTP password, and localfile.txt is the local file.
The above is the detailed content of How to use FTP to upload files in Python. For more information, please follow other related articles on the PHP Chinese website!
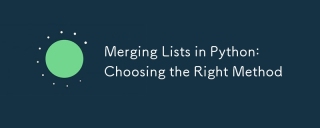
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
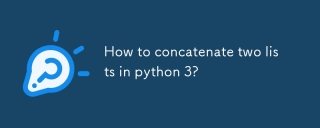
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
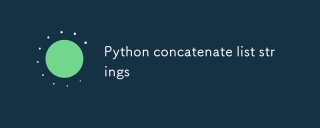
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
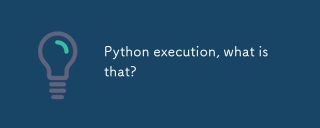
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
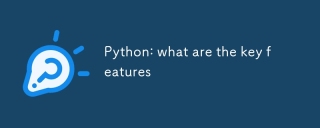
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
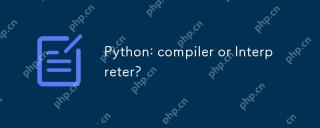
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
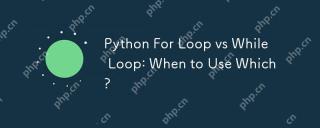
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
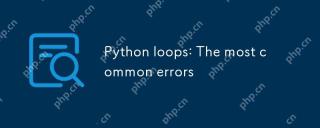
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
