


Steps and methods to implement automated collection tasks using Python and pywinauto
Automated collection tasks based on pywinauto
Implementation technology
This program uses a Python automation library----pywinauto, because the official version has not been updated for a long time, so the python version The highest can only be around Python 3.7, I use Python 3.7.1. I used it to simulate entering words, copying example sentences, getting example sentences, clearing the clipboard, and then repeating this operation. Overall, the implementation is relatively crude. Moreover, in order Simple, I manually switch to the example sentence page, so I don’t have to use a program to switch to the example sentence page.
Code
requirements.txt
pyperclip==1.8.2 pywin32==304 pywinauto==0.6.8
Code
import os import random import time import re from typing import Dict, List from pywinauto.application import Application from pywinauto import mouse from pywinauto import keyboard import pyperclip import json # 程序处理中的各种路径 dir_path = r"C:/Users/Dick/Desktop/work/DragonEnglish/tools" input_path = os.path.join(dir_path, r"input.txt") output_path = os.path.join(dir_path, r"output.json") error_path = os.path.join(dir_path, r"error.txt") # 顺序错误的单词 error_words = [] # 有道词典的进程id processId = 13840 def line_process(content: str) -> str: """ 去除所有空行, 再去除前面四行无关内容 """ lines = content.split("\r\n") # 因为例句开头是 数字. 开头的, 所以先以这个为特点来进行过滤掉多复制的开头 count = 0 for i in range(len(lines)): if re.match(r"\d+\.", lines[i]): count = i break lines = lines[count:] filter_lines = [] for line in lines: if line.strip() != "": # 过滤空行 if not line.startswith("youdao") and not \ (line.startswith("《") and line.endswith("》")): # 过滤来源 filter_lines.append(line) if len(filter_lines) % 3 != 0: raise Exception("抓取数据错误") content = "\n".join(filter_lines) + "\n" # 补上一个 \n, 不然正则会漏掉一个结果 return content def to_list(line: str) -> List[Dict[str, str]]: """ 直接生成列表字典对象 [{ "no": 1, "original": "", "translate" }] """ sentences = [] # 正则表达式 REGEXP = r'(?P<no>\d+?)\.\n(?P<original>.+?)\n(?P<translate>.+?)\n' # 编译 pattern = re.compile(REGEXP) # 匹配 rs = pattern.finditer(line) # 组装结果 for r in rs: print(r.groupdict()) sentences.append(r.groupdict()) return sentences if __name__ == "__main__": # 连接网易有道词典 app = Application(backend="uia").connect(process=processId) # 获取需要的窗口 win = app.window(class_name="RICHEDIT50W") # 输入词汇列表 input_words = [] # 输出词汇对象列表 output_words = [] # 打开输入文件,初始化输入词汇列表 with open(input_path, "r", encoding="utf-8") as input_file: input_words = input_file.read().split("\n") for word in input_words: print("正在抓取单词: %s" % word) # 清空剪切板,这步很重要,防止重复复制 pyperclip.copy("") # 将输入数据复制到剪切板 pyperclip.copy(word) # 定位到输入框(采用坐标定位,定位到大致位置即可) mouse.click(coords=(2400, 80)) # 模拟按键操作:全选 删除 粘贴 回车(触发查询) keyboard.send_keys("^a{DELETE}^v{ENTER}") # 清空剪切板,这步很重要,防止重复复制 pyperclip.copy("") # 鼠标左键点击,这个操作只是为了把鼠标移动到这里 mouse.click(button="left", coords=(2200, 330)) # 模拟键盘 CTRL+A CTRL+C,直接全选所有的例句(这里会多选一部分内容,待会再处理) keyboard.send_keys("^a^c") # 暂停一会儿,不做操作的太快 time.sleep(random.random() * 2 + 1) # pywinauto 复制的内容是在系统的剪切板里面的,所以需要其它库读取 content = pyperclip.paste() # 对内容进行简单的预处理后,加入output_words try: lines = line_process(content) except BaseException as exp: print(exp) # 如果抓取出现问题,说明被网易抓了现行,直接退出即可。 break sentences = to_list(lines) if not sentences: print("获取例句为空, 可能是数据格式错误.") break output_words.append({ "word": word, "example": sentences, }) # 模拟暂停一个较长的随机时间,没有必要追求速度,平稳运行即可。 time.sleep(random.random() * 3 + 3) # 清空剪切板,这步很重要,防止重复复制 pyperclip.copy("") # 抓取完毕一个文件的内容后,然后一次性写入即可。 # 之前的写法是一个单词写入一次,会造成太多的IO次数,浪费性能! with open(output_path, "a+", encoding="utf-8") as output_file: output_file.write(json.dumps( output_words, ensure_ascii=False, indent=4)) # 错误单词记录 with open(error_path, "w", encoding="utf-8") as err_file: err_file.writelines("\n".join(error_words))
Demonstration If you want to start this code, it is quite complicated. I will just list the steps required here, hoping to help. Interested students.
Modify dir_path and prepare an input.txt file below.
Get the ID of the Youdao Dictionary process.
Get the coordinates of the word input box and get the coordinates of the copy and paste location.
-
Adjust the Youdao dictionary interface to the example sentence.
To start the project, you need an input.txt file. Here is the file I tested.
##sophisticatedI obtained the process pid through the task manager, you can also access it through other methods. Or the simplest is to use Inspect and Spy, I am lazy here , how to save trouble directly.centralization
phenomenon
internationalization
radioactive
Console output
The above is the detailed content of Steps and methods to implement automated collection tasks using Python and pywinauto. For more information, please follow other related articles on the PHP Chinese website!
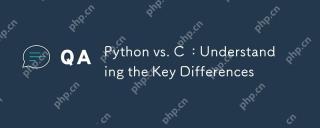
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
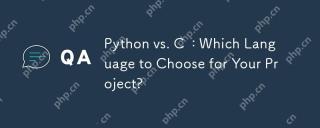
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
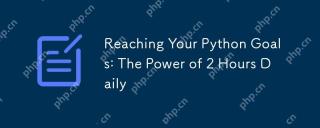
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
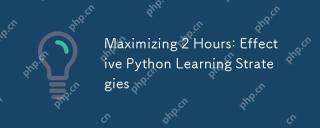
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
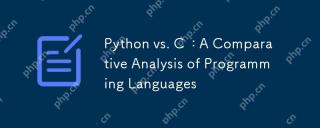
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
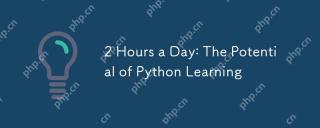
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
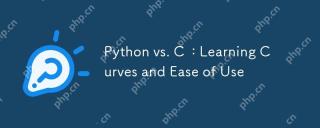
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
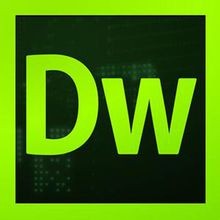
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool