In PHP programming, it is a very common requirement to convert a number string into an array, such as reading a column of numbers in a csv file, storing it as an array for subsequent operations, or reading a column of numbers from a database. , also needs to be converted into an array for processing. This article introduces several methods to convert digital strings into arrays.
Method 1: Use str_split function
str_split function can split the string into an array, for example:
$str = "123456"; $arr = str_split($str); print_r($arr);
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 )
This kind The method is very simple, but it should be noted that if the number string contains a negative sign or a decimal point, this method will not handle it correctly.
Method 2: Use the preg_split function
The preg_split function can split the string into an array according to the specified delimiter, for example:
$str = "1,2,3,4,5,6"; $arr = preg_split('/,/', $str); print_r($arr);
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 )
This method is more flexible than str_split, and different delimiters can be specified according to the actual situation.
Method 3: Use the explode function
The explode function can also split the string into an array according to the specified delimiter, for example:
$str = "1,2,3,4,5,6"; $arr = explode(',', $str); print_r($arr);
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 )
Compared with the preg_split function, the explode function is more concise, but its performance is slightly worse than preg_split.
Method 4: Use the str_split and array_map functions
This method combines the str_split and array_map functions to convert the digital string into an array, for example:
$str = "123456"; $arr = array_map('intval', str_split($str)); print_r($arr);
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 )
This method uses the array_map function to convert a string array into an integer array, while maintaining the splitting capability of the str_split function.
Method 5: Use regular expressions
If you need to parse complex numeric strings, such as "1,2,3 | 4,5,6 | 7,8,9", Regular expressions can be used for parsing, for example:
$str = "1,2,3 | 4,5,6 | 7,8,9"; $arr = preg_split('/[\s|,]+/', $str, -1, PREG_SPLIT_NO_EMPTY); print_r($arr);
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 [6] => 7 [7] => 8 [8] => 9 )
This method uses [\s|,] in regular expressions to match any spaces, vertical bars and comma, and use the PREG_SPLIT_NO_EMPTY flag to ensure that no empty elements are split.
Summary
The above methods can convert digital strings into arrays, each with its own advantages and disadvantages. It is necessary to choose the most suitable method according to the actual situation.
The above is the detailed content of How to convert numeric string into array in php. For more information, please follow other related articles on the PHP Chinese website!
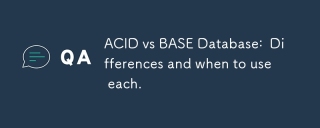
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
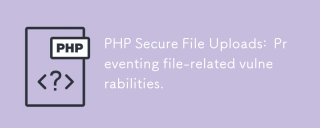
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
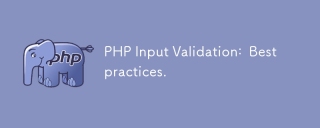
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
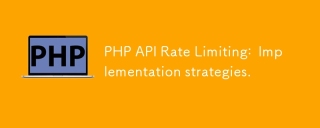
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
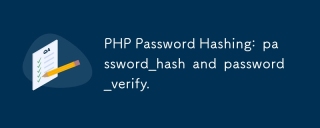
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
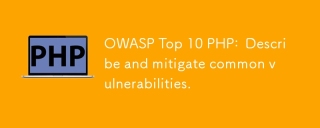
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
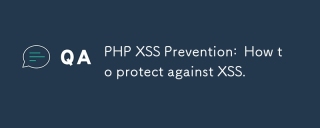
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
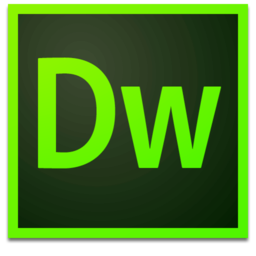
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.