In PHP, querying whether a value belongs to an array is a very basic operation. This article will introduce three different methods to determine whether a value belongs to an array.
First method: use the in_array() function
PHP provides an in_array() function, which can be used to determine whether a value belongs to an array. This function has two parameters: the first parameter is the value to be queried, and the second parameter is the array to be queried. The function will return true if the first argument belongs to the second argument, false otherwise.
The following is an example:
<?php $fruits = array('apple', 'banana', 'orange'); if (in_array('apple', $fruits)) { echo 'apple belongs to fruits array'; } else { echo 'apple does not belong to fruits array'; } ?>
The above code will output: apple belongs to fruits array
Second method: use array_search() function
PHP also provides another function array_search(), which can be used to query whether a value belongs to an array, and this function can also return the position of the value in the array. If the query is successful, this function will return the key name of the value in the array, otherwise it will return false.
The following is an example:
<?php $fruits = array('apple', 'banana', 'orange'); $search = array_search('apple', $fruits); if ($search !== false) { echo 'apple belongs to fruits array, and its key is ' . $search; } else { echo 'apple does not belong to fruits array'; } ?>
The above code will output: apple belongs to fruits array, and its key is 0
Please note that if false is found in the array , 0 or '', will also return false, so we need to use the identity operator (===) to distinguish between returning false and returning 0.
Third method: Use isset() function
isset() function is used to determine whether a variable exists and whether the variable has a value set. Because in an array, whether a certain key has been set to a certain value is often the result of whether we want the query. Therefore, we can also use the isset() function to determine whether a value belongs to an array. The isset() function will return true if the variable has been set to a certain value, otherwise it will return false.
The following is an example:
<?php $fruits = array('apple', 'banana', 'orange'); if (isset($fruits[array_search('apple', $fruits)])) { echo 'apple belongs to fruits array'; } else { echo 'apple does not belong to fruits array'; } ?>
The above code will output: apple belongs to fruits array
Please note that since the array_search() function is used in this example, We have judged the results to avoid certain unforeseen errors.
The above is the detailed content of How to check whether a certain value belongs to an array in php. For more information, please follow other related articles on the PHP Chinese website!
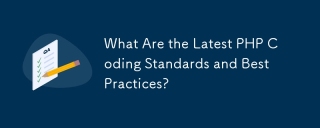
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
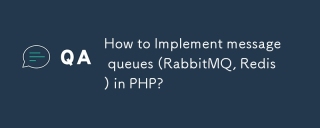
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
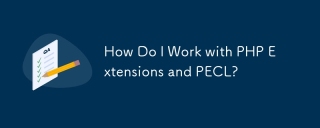
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
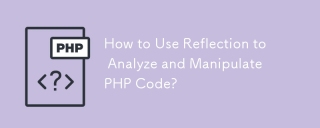
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
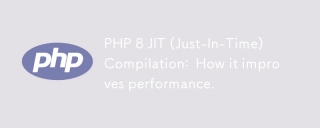
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
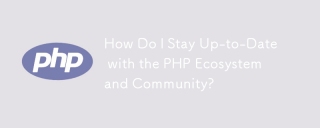
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
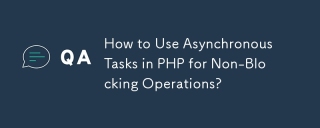
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
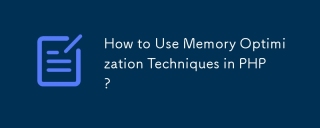
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
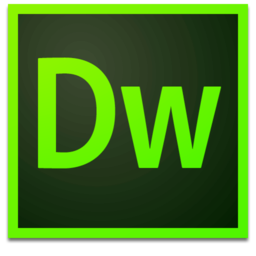
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
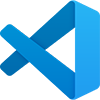
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
