In PHP, there are many ways to determine whether the key element in the array exists. This article will introduce three commonly used methods.
Method 1: Use isset() function
isset() function is used to check whether a variable has been defined and is not NULL. We can use the isset() function to determine whether the key in the array exists. An example is as follows:
// 定义一个数组 $arr = array( 'name' => '张三', 'age' => 25, 'sex' => '男' ); // 判断数组中的 key 是否存在 if (isset($arr['name'])) { echo '姓名存在'; } else { echo '姓名不存在'; }
Method 2: Use array_key_exists() function
array_key_exists() function is used to check whether the specified key name exists in the array. We can use the array_key_exists() function to determine whether the key in the array exists. Examples are as follows:
// 定义一个数组 $arr = array( 'name' => '张三', 'age' => 25, 'sex' => '男' ); // 判断数组中的 key 是否存在 if (array_key_exists('name', $arr)) { echo '姓名存在'; } else { echo '姓名不存在'; }
Method 3: Use the in_array() function
The in_array() function is used to find whether a value exists in an array. We can use the in_array() function to determine whether the key in the array exists. Examples are as follows:
// 定义一个数组 $arr = array( 'name' => '张三', 'age' => 25, 'sex' => '男' ); // 判断数组中的 key 是否存在 if (in_array('name', array_keys($arr))) { echo '姓名存在'; } else { echo '姓名不存在'; }
The above three methods can be used to determine whether the key in the array exists, among which the isset() function is the most commonly used method. If you are judging the value in the array instead of the key, you can use the in_array() function.
The above is the detailed content of How to determine whether array key element exists in php. For more information, please follow other related articles on the PHP Chinese website!
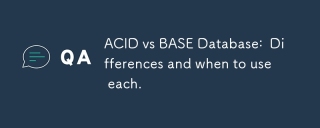
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
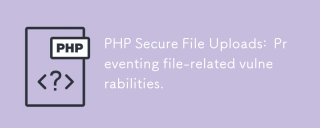
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
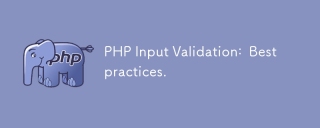
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
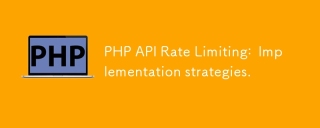
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
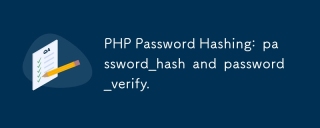
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
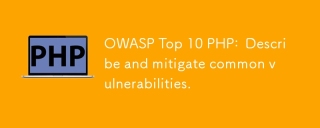
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
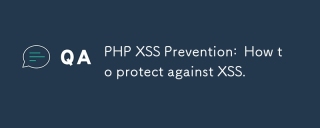
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
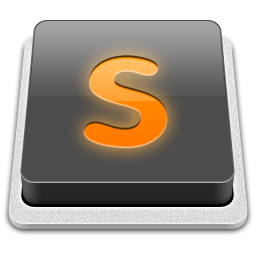
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.