Python collection (array)
There are four collection data types in the Python programming language:
List (List) is an ordered and changeable gather. Duplicate members are allowed.
Tuple is an ordered and unchangeable collection. Duplicate members are allowed.
Set is an unordered and unindexed collection. There are no duplicate members.
A dictionary is an unordered, mutable and indexed collection. There are no duplicate members.
When choosing a collection type, it is useful to know the properties of that type.
Choosing the right type for a specific data set can mean preserving meaning, and it can mean improving efficiency or security.
List
A list is an ordered and changeable collection. In Python, lists are written using square brackets.
Instance
Create List:
thislist = ["apple", "banana", "cherry"] print(thislist)
Run Instance
Access Project
You can access a list item by referencing the index number:
Example
Print the second item of the list:
thislist = ["apple", "banana", "cherry"] print(thislist[1])
Run the example
Negative index
Negative index means starting from the end, -1 means the last item, -2 means the second to last item, and so on .
Example
Print the last item in the list:
thislist = ["apple", "banana", "cherry"] print(thislist[-1])
Run the example
Instance
Return the third, fourth, and fifth items:thislist = ["apple", "banana", "cherry", "orange", "kiwi", "melon", "mango"] print(thislist[2:5])Run instance
Example
This example Will return items from index -4 (included) to index -1 (excluded):thislist = ["apple", "banana", "cherry", "orange", "kiwi", "melon", "mango"] print(thislist[-4:-1])Run instance
Instance
Change the second item:thislist = ["apple", "banana", "cherry"] thislist[1] = "mango" print(thislist)Run Example
Example
One by one Print all items in the list:thislist = ["apple", "banana", "cherry"] for x in thislist: print(x)Run Example
Example
Check if "apple" exists in the list:thislist = ["apple", "banana", "cherry"] if "apple" in thislist: print("Yes, 'apple' is in the fruits list")Running instance
Example
Print the number of items in the list:thislist = ["apple", "banana", "cherry"] print(len(thislist))Run the example
Example
Use the append() method to append an item:thislist = ["apple", "banana", "cherry"] thislist.append("orange") print(thislist)Run the instance
Instance
Insert item as second position:thislist = ["apple", "banana", "cherry"] thislist.insert(1, "orange") print(thislist)Run instance
##Delete items
There are several ways to delete items from the list:
Example The remove() method deletes the specified item: thislist = ["apple", "banana", "cherry"]
thislist.remove("banana")
print(thislist)
Run the example
thislist = ["apple", "banana", "cherry"] thislist.pop() print(thislist)Run instance
实例
del 关键字删除指定的索引:
thislist = ["apple", "banana", "cherry"] del thislist[0] print(thislist)
运行实例
实例
del 关键字也能完整地删除列表:
thislist = ["apple", "banana", "cherry"] del thislist
运行实例
实例
clear() 方法清空列表:
thislist = ["apple", "banana", "cherry"] thislist.clear() print(thislist)
运行实例
复制列表
您只能通过键入 list2 = list1 来复制列表,因为:list2 将只是对 list1 的引用,list1 中所做的更改也将自动在 list2 中进行。
有一些方法可以进行复制,一种方法是使用内置的 List 方法 copy()。
实例
使用 copy() 方法来复制列表:
thislist = ["apple", "banana", "cherry"] mylist = thislist.copy() print(mylist)
运行实例
制作副本的另一种方法是使用内建的方法 list()。
实例
使用 list() 方法复制列表:
thislist = ["apple", "banana", "cherry"] mylist = list(thislist) print(mylist)
运行实例
合并两个列表
在 Python 中,有几种方法可以连接或串联两个或多个列表。
最简单的方法之一是使用 + 运算符。
实例
合并两个列表:
list1 = ["a", "b" , "c"] list2 = [1, 2, 3] list3 = list1 + list2 print(list3)
运行实例
连接两个列表的另一种方法是将 list2 中的所有项一个接一个地追加到 list1 中:
实例
把 list2 追加到 list1 中:
list1 = ["a", "b" , "c"] list2 = [1, 2, 3] for x in list2: list1.append(x) print(list1)
运行实例
或者,您可以使用 extend() 方法,其目的是将一个列表中的元素添加到另一列表中:
实例
使用 extend() 方法将 list2 添加到 list1 的末尾:
list1 = ["a", "b" , "c"] list2 = [1, 2, 3] list1.extend(list2) print(list1)
运行实例
list() 构造函数
也可以使用 list() 构造函数创建一个新列表。
实例
使用 list() 构造函数创建列表:
thislist = list(("apple", "banana", "cherry")) # 请注意双括号 print(thislist)
运行实例
列表方法
Python 有一组可以在列表上使用的内建方法。
The above is the detailed content of What are the application methods of Python lists?. For more information, please follow other related articles on the PHP Chinese website!
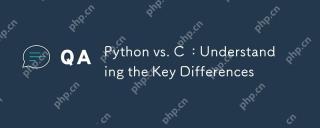
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
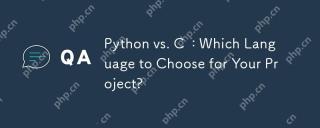
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
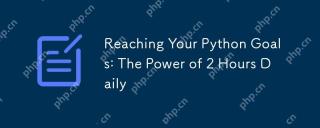
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
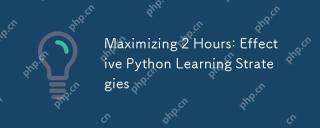
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
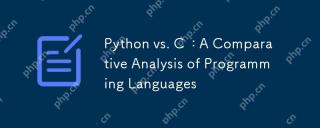
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
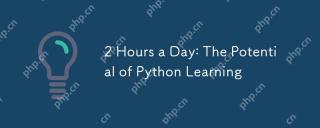
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
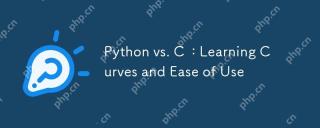
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.