In golang, sometimes it is necessary to handle newlines (\n) for better access and manipulation of text. But sometimes it is necessary to remove line breaks from text in order to perform certain calculations or statistical functions.
This article will introduce how to remove newlines in golang. We will demonstrate through several different methods and compare the similarities and differences between them to be able to understand better.
1. Strings.Replace function
The strings.Replace function can replace certain characters in the character sequence with other characters or delete characters. Here we can use this function to remove newlines from text.
The following is an example of using the strings.Replace function to remove newlines:
package main import ( "fmt" "strings" ) func main() { text := "hello\nworld\n" newText := strings.Replace(text, "\n", "", -1) fmt.Println("原文本:", text) fmt.Println("新文本:", newText) }
Output:
原文本: hello world 新文本: helloworld
2. Strings.Trim function
strings. The Trim function can remove specified characters at the beginning and end of a string. Here, we can use the newline character as the specified character and use this function to remove the newline character from the text.
The following is an example of using the strings.Trim function to remove newlines:
package main import ( "fmt" "strings" ) func main() { text := "hello\nworld\n" newText := strings.Trim(text, "\n") fmt.Println("原文本:", text) fmt.Println("新文本:", newText) }
Output:
原文本: hello world 新文本: helloworld
3. Strings.Join and strings.Split functions
strings.Join function can join the string array into a string using the specified delimiter. The strings.Split function can split a string into a string array using the specified delimiter.
Here, we can use the strings.Split function to split the text and the strings.Join function to join all the lines in the text into one string. This method is somewhat similar to using the strings.Replace function, but concatenating all the lines into a single string is more concise.
The following is an example of using the strings.Join and strings.Split functions to remove newlines:
package main import ( "fmt" "strings" ) func main() { text := "hello\nworld\n" lineArray := strings.Split(text, "\n") newText := strings.Join(lineArray, "") fmt.Println("原文本:", text) fmt.Println("新文本:", newText) }
Output:
原文本: hello world 新文本: helloworld
4. bufio.Scanner and bytes.Buffer
A more low-level and flexible method is to use bufio.Scanner and bytes.Buffer. bufio.Scanner is used to read data from an input source (such as a file or string) and split it into words. Bytes.Buffer is used to dynamically cache byte arrays.
Here we can put the text into bytes.Buffer and then use bufio.Scanner to read data from it. When reading data, we can add all characters to the new bytes.Buffer but skip newlines. This method is more flexible than the previous method because we can perform more complex judgments and processing of characters.
Here is an example of using bufio.Scanner and bytes.Buffer to remove newlines:
package main import ( "bufio" "bytes" "fmt" ) func main() { text := "hello\nworld\n" buf := bytes.NewBufferString(text) scanner := bufio.NewScanner(buf) newBuf := bytes.Buffer{} for scanner.Scan() { newBuf.WriteString(scanner.Text()) } if scanner.Err() != nil { fmt.Println("读取数据时出现错误。") } fmt.Println("原文本:", text) fmt.Println("新文本:", newBuf.String()) }
Output:
原文本: hello world 新文本: helloworld
In this article, we demonstrate that in golang Different ways to remove newlines in . These methods include using the strings.Replace, strings.Trim, strings.Join and strings.Split functions, as well as more low-level methods using bufio.Scanner and bytes.Buffer. Each of these methods has its own advantages and disadvantages, and you can choose the appropriate method for processing according to your needs.
The above is the detailed content of How to remove newlines in golang. For more information, please follow other related articles on the PHP Chinese website!
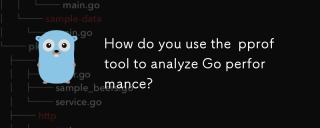
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
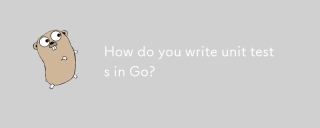
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
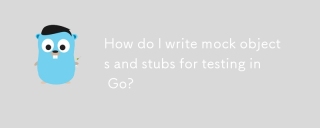
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
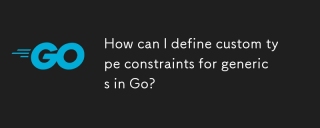
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
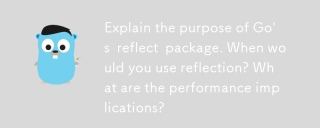
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
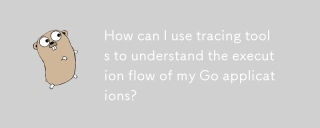
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
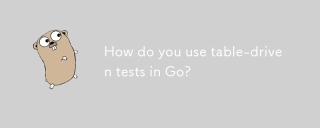
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
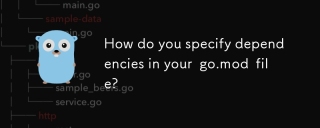
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
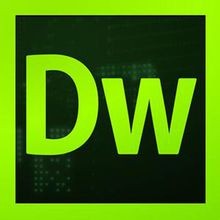
Dreamweaver CS6
Visual web development tools
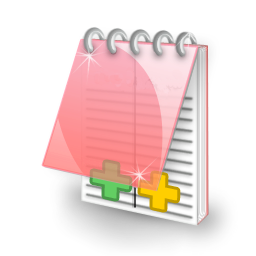
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
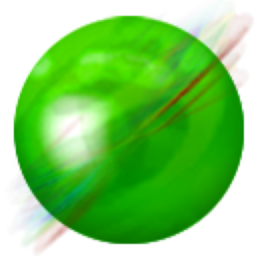
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
