Go language is a popular programming language, especially in the field of network programming. In network programming, setting PMTU (Path Maximum Transmission Unit) is very important, and the Go language also provides some APIs to set PMTU. This article will introduce how to set PMTU in Go language.
What is PMTU?
PMTU (Path Maximum Transmission Unit) refers to the maximum load that a data packet can carry during network transmission. When transmitting data, the size of the packets cannot be too large, otherwise they may be corrupted or lost. Therefore, the size of the data packet needs to be limited before transmission, which is what PMTU does. PMTU can vary based on network topology and device configuration, so it needs to be adjusted dynamically when transmitting data.
PMTU settings in Go language
When using Go language for network programming, we need to understand how to set PMTU to ensure the transmission of data packets in the network. The Go language provides some APIs to set PMTU. Next, we will introduce two methods of setting PMTU: using the net package and using raw socket.
Use net package
Using net package can easily adjust PMTU. The following is a sample program:
package main import ( "net" "fmt" ) func main() { conn, err := net.Dial("udp", "127.0.0.1:5555") if err != nil { fmt.Println(err) return } defer conn.Close() conn.SetWriteBuffer(65535) conn.SetReadBuffer(65535) err = conn.Write([]byte("Hello World!")) if err != nil { fmt.Println(err) return } buf := make([]byte, 4096) n, err := conn.Read(buf) if err != nil { fmt.Println(err) return } fmt.Println(string(buf[0:n])) }
In the routine, the size of the write and read buffers is set to 65535 bytes to ensure that data can be transmitted. Of course, this value can be adjusted according to actual conditions.
Using raw socket
Using raw socket to set PMTU is relatively complicated. First, you need to create an original socket, and then set the size of its data packet. The following is a sample program:
package main import ( "fmt" "syscall" "unsafe" ) const ( IPPROTO_IP = 0 IP_MTU_DISCOVER = 10 IP_PMTUDISC_DO = 2 IP_MTU = 14 ) func main() { fd, err := syscall.Socket(syscall.AF_INET, syscall.SOCK_RAW, syscall.IPPROTO_RAW) if err != nil { fmt.Printf("Error: %v\n", err) return } defer syscall.Close(fd) pmtu, err := syscall.GetsockoptInt(fd, IPPROTO_IP, IP_MTU) if err != nil { fmt.Printf("Error: %v\n", err) return } fmt.Printf("PMTU=%d\n", pmtu) flag := IP_PMTUDISC_DO _, _, e := syscall.Syscall6(syscall.SYS_SETSOCKOPT, uintptr(fd), uintptr(IPPROTO_IP), uintptr(IP_MTU_DISCOVER), uintptr(unsafe.Pointer(&flag)), unsafe.Sizeof(flag), 0) if e != 0 { fmt.Printf("Error: %v\n", e) return } pmtu, err = syscall.GetsockoptInt(fd, IPPROTO_IP, IP_MTU) if err != nil { fmt.Printf("Error: %v\n", err) return } fmt.Printf("PMTU=%d\n", pmtu) }
In the routine, since you want to use the original socket, you first need to obtain privileges to obtain sufficient permissions. When setting PMTU, you need to call the systemcall.SetsockoptInt() API to change the socket settings. This process is relatively complicated.
Summary
Whether you use the net package or raw socket, setting PMTU in Go language is very simple. We need to choose the appropriate method to set up according to the actual situation to ensure that data can be transmitted stably in the network. At the same time, we need to pay attention to adjusting the size of the data packet and maintaining a reasonable PMTU value.
The above is the detailed content of How to set pmtu in golang. For more information, please follow other related articles on the PHP Chinese website!
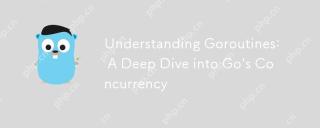
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
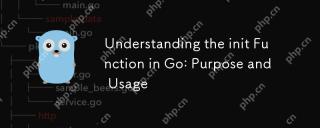
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
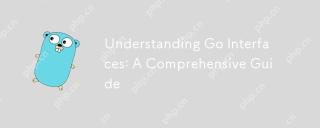
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
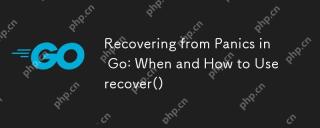
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
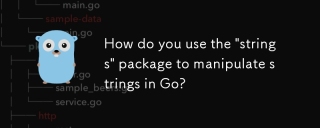
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
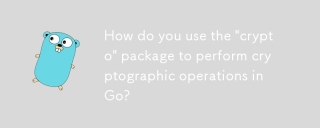
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
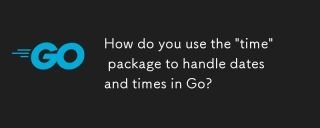
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
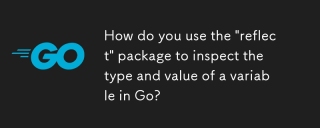
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
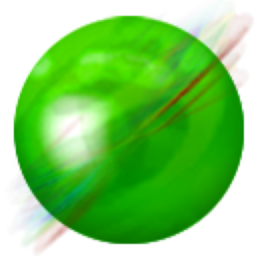
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
