In PHP, array is a very common data structure that allows us to store data in an ordered collection. Usually, we use arrays to store some relevant data, such as attributes of a product or information about a person, etc.
Sometimes, we need to delete a column of data in an array, either because we no longer need that particular column, or because we need to rearrange the data. Whatever the reason, PHP provides some simple methods to help us deal with arrays easily.
This article will introduce some methods that allow you to easily delete a column of data in an array.
- Use the unset() function
The unset() function is a powerful function in PHP that can help us delete any non-array values, array keys and array elements .
We can use the unset() function to delete a column of data in the array, as shown below:
// 定义一个数组 $myArray = array( array("name" => "John", "age" => 37, "sex" => "male"), array("name" => "Lisa", "age" => 25, "sex" => "female"), array("name" => "David", "age" => 42, "sex" => "male") ); // 删除sex列 foreach($myArray as &$value) { unset($value["sex"]); } // 输出结果 print_r($myArray);
In the above example, we use the unset() function to delete a column of data in the array. sex" column. We use a foreach loop to iterate through each element in the array and use the unset() function to delete the corresponding column.
- Using the array_filter() function
The array_filter() function is another practical function in PHP that can help us filter arrays.
We can use the array_filter() function in conjunction with the array_map() function to delete a column of data in the array, as shown below:
// 定义一个数组 $myArray = array( array("name" => "John", "age" => 37, "sex" => "male"), array("name" => "Lisa", "age" => 25, "sex" => "female"), array("name" => "David", "age" => 42, "sex" => "male") ); // 删除sex列 $myArray = array_map(function($value) { unset($value["sex"]); return $value; }, $myArray); // 输出结果 print_r($myArray);
In the above example, we use the array_map() function to iterate over each element in the array and use an anonymous function to delete the corresponding column. We store the results in a new array and replace the original array with the returned results.
- Using the array_reduce() function
The array_reduce() function is another practical function in PHP that can help us operate on arrays recursively.
We can use the array_reduce() function in combination with a custom function to delete a column of data in the array, as shown below:
// 定义一个自定义函数 function removeColumn($carry, $item) { unset($item["sex"]); $carry[] = $item; return $carry; } // 定义一个数组 $myArray = array( array("name" => "John", "age" => 37, "sex" => "male"), array("name" => "Lisa", "age" => 25, "sex" => "female"), array("name" => "David", "age" => 42, "sex" => "male") ); // 删除sex列 $myArray = array_reduce($myArray, "removeColumn"); // 输出结果 print_r($myArray);
In the above example, we defined a custom Function removeColumn(), used to delete the "sex" column. We use the array_reduce() function to iterate through each element in the array, and the removeColumn() function to delete the corresponding column. We store the results in a new array and replace the original array with the returned results.
Summary
The above are three common ways to delete a column of data from a PHP array. Each method has its advantages, disadvantages and applicable scenarios. In actual development, please choose the appropriate method according to your own needs and data structure.
The above is the detailed content of How to remove a column from php array. For more information, please follow other related articles on the PHP Chinese website!
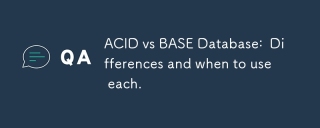
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
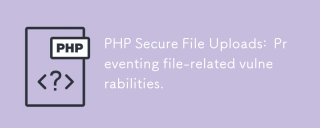
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
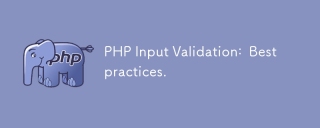
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
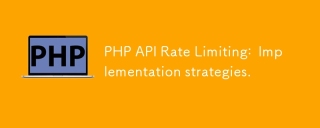
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
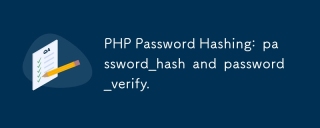
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
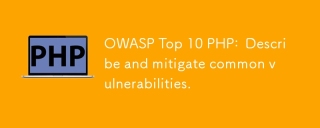
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
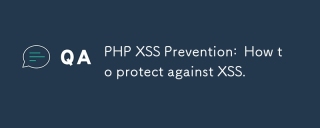
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
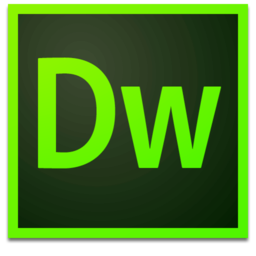
Dreamweaver Mac version
Visual web development tools
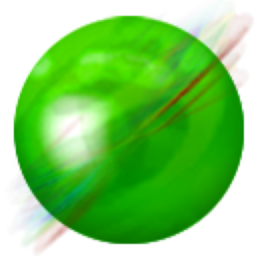
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment