PHP is a popular open source programming language that is widely used in web development. In PHP, variables are a very important data type that can store data and be accessed and modified by the program. In this article, we will explain how to query results from PHP variables.
1. Declare variables
In PHP, you can declare variables through the assignment operator "=". The variable name needs to start with the "$" symbol, for example:
$name = "Tom"; //String type
$age = 20; //Integer type
$score = 85.5; //Floating point type
$flag = true; //Boolean type
These variables can store different types of data, string type stores any characters, integer type Stores any integer, the floating point type stores decimals, and the Boolean type stores "true" or "false".
2. Query variable results
PHP provides various statements to query variable results. The following are some commonly used query result statements:
2.1.echo statement
Use the echo statement to output the value of a variable to a browser or Web page. For example, the following code will output "Tom":
$name = "Tom";
echo $name;
The echo statement can also output multiple variables, for example:
$name = "Tom";
$age = 20;
echo "Name: " . $name . ", Age: " . $age;
The above code will output " Name: Tom, Age: 20".
2.2.print statement
The print statement is similar to the echo statement and can output the value of the variable to the browser or Web page. For example, the following code will output "Tom":
$name = "Tom";
print $name;
The print statement can also output multiple variables, for example:
$name = "Tom";
$age = 20;
print "Name: " . $name . ", Age: " . $age;
The above code will output " Name: Tom, Age: 20".
2.3.var_dump function
The var_dump function can output the type and value of the variable. For example, the following code will output the type and value of the variable $name:
$name = "Tom";
var_dump($name);
The above code will output "string(3 ) "Tom"", indicating that the type of variable $name is string and the value is "Tom".
2.4.gettype function
The gettype function can query the type of a variable. For example, the following code will output the type of variable $name:
$name = "Tom";
echo gettype($name);
The above code will output "string".
3. Query array results
The array in PHP is a commonly used data type, which can store multiple variables, called "elements". The following are some commonly used statements for querying array results:
3.1.print_r function
The print_r function can output all elements of the array and their values. For example, the following code will output all elements of the array $grades and their values:
$grades = array("Tom" => 90, "Jack" => 85, "Mary" => 95);
print_r($grades);
The above code will output "Array ([Tom] => 90 [Jack] => 85 [Mary] => 95 )".
3.2.var_dump function
The var_dump function can also output all elements of the array and their types and values. For example, the following code will output all elements of the array $grades and their types and values:
$grades = array("Tom" => 90, "Jack" => 85, "Mary" = > 95);
var_dump($grades);
The above code will output "array(3) { ["Tom"]=> int(90) ["Jack"]=> int(85) ["Mary"]=> int(95) }", indicating that the array $grades has 3 elements, their types are integers, and their values are 90, 85, and 95 respectively.
4. Query object results
The object in PHP is a complex data type that encapsulates properties and methods. The following are some commonly used statements for querying object results:
4.1.print_r function
The print_r function can output all attributes of the object and their values. For example, the following code will output all properties of the object $person and their values:
class Person {
public $name; public $age; public function sayHello() { echo "Hello, my name is " . $this->name; }
}
$person = new Person();
$person->name = "Tom";
$person->age = 20;
print_r($person);
The above code will output "Person Object ([name] => Tom [age] => 20 )".
4.2.var_dump function
The var_dump function can also output all properties of the object and their types and values. For example, the following code will output all properties of the object $person and their types and values:
class Person {
public $name; public $age; public function sayHello() { echo "Hello, my name is " . $this->name; }
}
$person = new Person();
$person->name = "Tom";
$person->age = 20;
var_dump($person);
The above code will output "object(Person) #1 (2) { ["name"]=> string(3) "Tom" ["age"]=> int(20) }", indicating that the object $person has 2 attributes, and their types are characters String and integer, the values are "Tom" and 20 respectively.
Summary
In PHP, variables are a very important data type that can store various types of data. By querying the results of variables, arrays, and objects, you can better understand their values and types, making it easier to develop and debug programs. The statements introduced above are only some common methods, and the specific application needs to be used flexibly according to different situations.
The above is the detailed content of How to query results from PHP variables. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
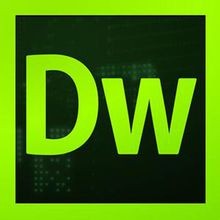
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
