I learned bit operation a long time ago, but I haven’t used it for a long time, and I feel like I have almost forgotten it. I recently looked at a few codes for bit arithmetic and found that I couldn’t understand them all, haha. It’s time to come back and catch up on the basics.
Operator |
Meaning |
& |
bitwise and |
| |
bitwise or |
~ |
Bitwise negation |
^ |
Bitwise XOR |
|
Shift left |
>> |
Shift right with sign |
##>> ;> | Unsigned right shift |
These are very basic knowledge, but if you don’t use it for too long, you will inevitably forget it. You can use it more while coding!
Talk is cheap, show me the code.
Note: It is really difficult to see the application when discussing these alone. If there is anything unclear You can check out other people’s summaries.
Let’s take a look at the application of bit operations with a code:
public final void writeInt(int v) throws IOException {
out.write((v >>> 24) & 0xFF);
out.write((v >>> 16) & 0xFF);
out.write((v >>> 8) & 0xFF);
out.write((v >>> 0) & 0xFF);
incCount(4);
}
This code is a method in the DataOutputStream
class, used to convert an int type integer Write to the stream. The naming of this method is very interesting. It is completely different from the public abstract void write(int b) throws IOException
in OutputStream
. The parameters of this method seem to indicate that it can write an integer to the stream, but the function of the method is not guessed, but depends on the description of the method.
public abstract void write(int b) throws IOException
Introduction in API:
Writes the specified byte to this output stream. The general contract for write is that one byte is written to the output stream. The byte to be written is the eight low-order bits of the argument b. The 24 high-order bits of b are ignored.
it It is to write a specific byte into the stream. We know that an int type variable occupies 32 bits and a byte occupies 8 bits, so an int type integer less than 256 (2^8) and the last 8 bits of the byte type integer are identical.
So this method is to write the lowest 8 bits of an int variable and ignore the remaining 24 bits. Be extra careful when using this method!
The byte to be written is the eight low-order bits of the argument b. The 24 high-order bits of b are ignored.
So, writing an int type variable completely into the stream is not a very simple problem. Let's go back to the code above: It is written four times in a row, each time writing one byte of data. In this way, an int type variable is turned into 4 bytes and written into the stream.
out.write((v >>> 24) & 0xFF); This method is to write the lower 8-digit number above, and this specific implementation is corresponding Provided by subclasses.
Let’s take a look at the diagram: A simple AND operation: It can be seen that the result of the operation retains the lower 8 bits, which is (v>>>24) & 0xFF The result of the operation.
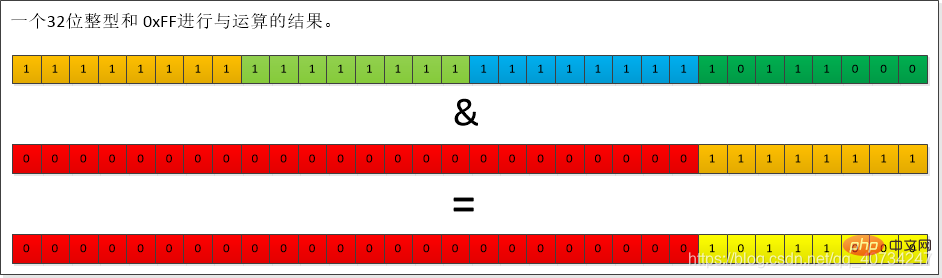
So how to get the high 8-bit value? This requires the use of shift operations:
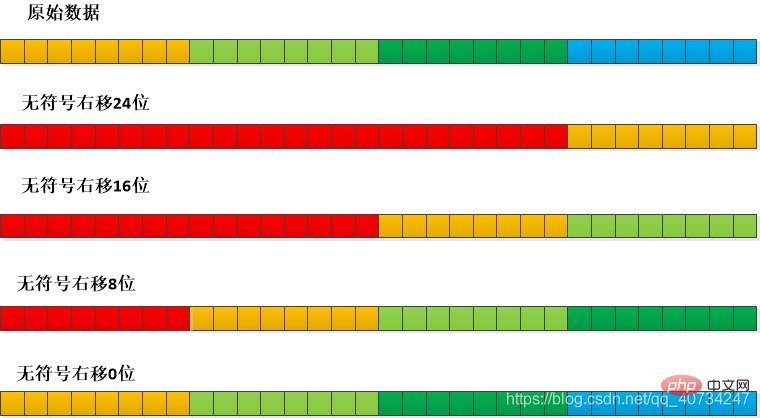
By performing the shift operation, you can obtain each 8-bit data, and then perform the bitwise AND & operation, You can completely write an integer into the stream.
Code Demonstration
Code
package dragon;
/**
* 分析这一个方法,目前水平有限,先从最简单的做起!
* */
// public final void writeInt(int v) throws IOException {
// out.write((v >>> 24) & 0xFF);
// out.write((v >>> 16) & 0xFF);
// out.write((v >>> 8) & 0xFF);
// out.write((v >>> 0) & 0xFF);
// incCount(4);
// }
//上面这段代码是将一个32位整型,写入输出流。
//并且是将32位整型分为4个部分,每次写入8位。
//这是Java的特性。
public class DataOutputStreamAnalysis {
public static void main(String[] args) {
DataOutputStreamAnalysis analysis = new DataOutputStreamAnalysis();
analysis.analysis(65535);
}
public void analysis(int number) {
int number1, number2, number3, number4; //后面的数字表示是一个32位整型的第几个8位。
number1 = (number >>> 24) & 0xFF;
number2 = (number >>> 16) & 0xFF;
number3 = (number >>> 8) & 0xFF;
number4 = (number >>> 0) & 0xFF;
System.out.println(this.format(Integer.toBinaryString(number))+" 原始数据");
System.out.println(this.format(Integer.toBinaryString(number1))+" 原始数据第一个8位");
System.out.println(this.format(Integer.toBinaryString(number2))+" 原始数据第二个8位");
System.out.println(this.format(Integer.toBinaryString(number3))+" 原始数据第三个8位");
System.out.println(this.format(Integer.toBinaryString(number4))+" 原始数据第四个8位");
}
/**
* 输入一个二进制字符串,将其格式化,因为整型是
* 占32位的,但是转换成的二进制字符串,并没有32位*/
public String format(String bstr) {
int len = bstr.length();
StringBuilder sb = new StringBuilder(35);
for (int i = 0; i < 32-len; i++) {
sb.append("0");
}
sb.append(bstr);
sb.insert(8, " ");
sb.insert(17, " ");
sb.insert(26, " "); //前面插入一个字符后,所有字符的索引都变了!
return sb.toString();
}
}
Result
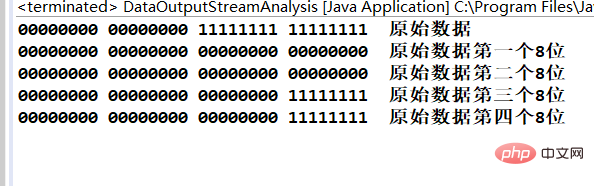
Explanation: Negative numbers are not considered here The situation is the same, except that the expression of negative numbers is a little more troublesome. As long as you understand positive numbers, negative numbers are not a problem.
Application of bit operations
1. Determine whether the int type variable x is an odd or even number
Perform a bitwise AND operation on the variables x and 1 , if the result is 0, then the variable x is an even number, otherwise it is an odd number.
if (x & 1 ==0)
System.out.println("x是偶数");
if (x & 1 == 1)
System.out.println("x是奇数");
Explanation: This is still easy to understand, because the final shift of even numbers must be 0. (Binary representation)
2. Take the k-th bit of the int type variable The binary value of the bit.
Expression: x >> k & 1 (It is recommended to add parentheses to make it clearer.)
3. Change the int type The k-th position 1 of variable Expression:
x = x | (1 4. Clear the k-th bit of the int type variable to 0 Shift 1 to the left by k bits and then invert the result, and then add the result to the variable for logical operation. Then the k-th bit of variable x is cleared to 0, and the other bits remain unchanged. Expression bits:
x = x & ~(1 ##5. Calculate the average of two integers Expression bits:(x & y) ((x ^ y) >> 1)
6. For an integer x greater than 1 , determine whether x is a power of 2
if (x & (x-1) == 0)
System.out.println("x是2的次幂");
7. Multiply a number by the nth power of 2
Expression: x = x
For example: expand x by 2 times: x = x
The reason why bitwise operations are recommended:
The speed of bit operations is faster than arithmetic operations, because bit operations require fewer instructions and require less time to execute. They will appear very fast, but the bit operations can only be seen when a large number of executions are performed. Advantages of operations. After all, today's computers are getting faster and faster.
The above is the detailed content of Java bit operation sample code analysis. For more information, please follow other related articles on the PHP Chinese website!