In PHP, a two-dimensional array is a very common data structure, usually used to store multiple collections of related data. When processing large amounts of data, using two-dimensional arrays can effectively reduce the amount of code and improve program execution efficiency.
This article will introduce how to create, traverse and operate two-dimensional arrays in PHP, as well as avoid some common pitfalls and mistakes.
1. Create a two-dimensional array
In PHP, the way to create a two-dimensional array is similar to creating a one-dimensional array. You only need to nest one or more arrays in the array. Create multidimensional arrays. The following is a simple example:
$students = array( array("name" => "Tom", "age" => 20, "score" => 80), array("name" => "Mary", "age" => 22, "score" => 90), array("name" => "John", "age" => 21, "score" => 85) );
In this example, $students is a two-dimensional array containing 3 sub-arrays, each sub-array contains three associated index keys: name, age and score.
In addition to using the array() function to create a two-dimensional array, you can also use the [][] symbol abbreviation, for example:
$students = [ ["name" => "Tom", "age" => 20, "score" => 80], ["name" => "Mary", "age" => 22, "score" => 90], ["name" => "John", "age" => 21, "score" => 85] ];
No matter which method is used to create a two-dimensional array, It is necessary to pay attention to keeping the structure of each sub-array consistent to avoid data inconsistency caused by incorrect index keys.
2. Traversing a two-dimensional array
Traversing a two-dimensional array usually requires the use of nested loops. The first-level loop is used to obtain each sub-array, and the second-level loop is used to traverse each sub-array. key-value pairs. The following is an example:
foreach ($students as $student) { foreach ($student as $key => $value) { echo "$key: $value<br>"; } }
In this example, a foreach() loop is used to traverse each subarray in turn, and then traverse each key-value pair of the subarray.
If you only need to get specific key-value pairs in a two-dimensional array, you can use the array_column() function, for example:
$names = array_column($students, "name"); print_r($names);
In this example, use the array_column() function to get the $students array The value of all names in , the result is a one-dimensional array, which is convenient to use.
3. Operating two-dimensional arrays
Operating two-dimensional arrays is roughly the same as operating one-dimensional arrays. You need to pay attention to the use of double index keys when traversing and accessing two-dimensional arrays. The following are some common examples of two-dimensional array operations:
- Add a new subarray to a two-dimensional array:
$students[] = array("name" => "Lucy", "age" => 19, "score" => 95);
- Modify an element in a two-dimensional array Subarray:
$students[0]["score"] = 85;
- Delete a subarray in a two-dimensional array:
unset($students[2]);
- Find the index position of a subarray in a two-dimensional array :
$index = array_search(array("name" => "Mary", "age" => 22, "score" => 90), $students);
In this example, use the array_search() function to find the value in the $students array array("name" => "Mary", "age" => 22, "score " => 90), the result is 1.
4. Common Errors and Precautions
When dealing with two-dimensional arrays, common errors and precautions include:
- When double using foreach() to traverse , you need to pay attention not to repeat the names of the outer loop variables and the inner loop variables.
- In order to facilitate the search, addition, modification and deletion of sub-arrays in a two-dimensional array, the associated key of the sub-array needs to be unique in the array.
- The index key of a two-dimensional array can be a number or a string, but consistency must be ensured, otherwise access errors may easily occur.
- When using functions such as array_search() to find subarrays in a two-dimensional array, you need to ensure that the order and type of the key-value pairs of the subarrays are consistent, otherwise you may get wrong results.
5. Summary
PHP processing two-dimensional arrays is a very common and important task in development. Proficient in the creation, traversal and operation of two-dimensional arrays can help developers be more Process large amounts of data efficiently. Pay attention to avoid common mistakes and precautions to improve the robustness and maintainability of your code.
The above is the detailed content of How php handles two-dimensional arrays. For more information, please follow other related articles on the PHP Chinese website!
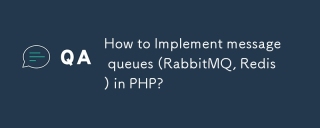
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
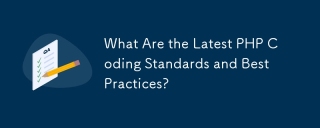
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
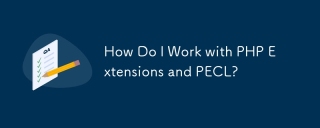
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
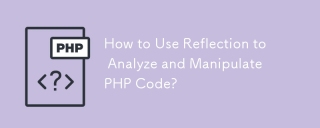
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
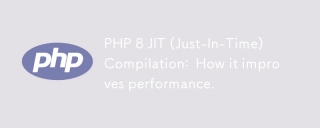
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
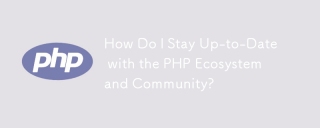
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
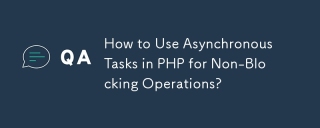
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
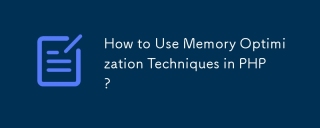
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
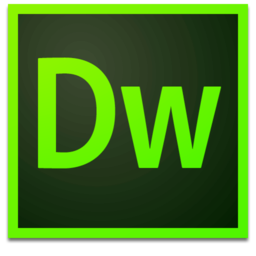
Dreamweaver Mac version
Visual web development tools
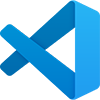
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
