When writing PHP applications, sometimes you need to change the field names of existing database tables. This situation may occur in the following scenarios:
- The database table design needs to be adjusted, or a better name is needed.
- To connect with other applications or systems, field names are required to be consistent.
This article will introduce how to change the field names of database tables in PHP.
Step 1: Connect to the database
Before you start changing the database table field names, you need to connect to the database first. You can connect to a MySQL database using PHP's built-in function mysqli_connect() or PDO. If you use other types of databases, you need to use the corresponding connection function.
The following is a sample code for using mysqli_connect() to connect to a MySQL database:
// 连接数据库 $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; $conn = mysqli_connect($servername, $username, $password, $dbname); // 检查连接是否成功 if (!$conn) { die("连接失败:" . mysqli_connect_error()); }
After connecting to the database, you can use the mysqli_query() function to execute SQL statements.
Step 2: Change the field name
To change the field name in the database table, you need to use the ALTER TABLE statement. In the ALTER TABLE statement, you need to specify the table name, the field name to be changed, and the new field name.
The following is a sample code to change the name of a table field:
// 更改字段名称 $sql = "ALTER TABLE myTable RENAME COLUMN oldColumnName TO newColumnName"; if (mysqli_query($conn, $sql)) { echo "字段名称更改成功"; } else { echo "错误:" . mysqli_error($conn); }
In this example, the field name of a table named myTable is changed. The changed column name is oldColumnName and the new column name is newColumnName.
Step 3: Close the database connection
After completing the database operation, the database connection must be closed to avoid occupying resources. The connection can be closed using the mysqli_close() function.
The following is a sample code to close the connection:
// 关闭连接 mysqli_close($conn);
Summary:
In PHP, changing the name of a database table field requires executing an ALTER TABLE statement. Use the ALTER TABLE statement to specify the table name to change, the field names to change, and the new field names. After connecting to the database and changing field names, the database connection must be closed.
Before changing the field name, please back up the database to avoid data loss. Additionally, you should ensure that existing applications or systems are not affected by the name change.
When writing code, please pay attention to handling exceptions and errors correctly. Possible errors should be caught and handled when using the mysqli_query() function.
The above are the basic steps and sample code for changing database table field names in PHP. Hopefully this information will help readers become more proficient when changing database table field names in PHP applications.
The above is the detailed content of How to change field name in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
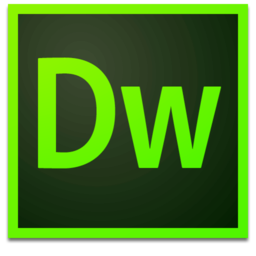
Dreamweaver Mac version
Visual web development tools
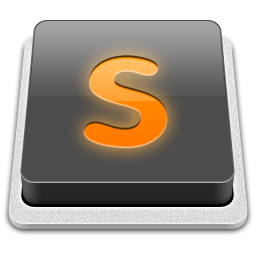
SublimeText3 Mac version
God-level code editing software (SublimeText3)
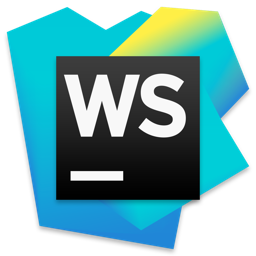
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
