In PHP, sometimes you need to traverse multiple arrays at the same time. For example, when merging two arrays, you need to use multiple array traversal operations. This article will explain how to iterate through multiple arrays in PHP.
Method 1: Using foreach loop nesting
Using foreach loop nesting is a common method of traversing multiple arrays. This method requires placing multiple arrays that need to be traversed into one array, and then using a nested foreach loop to traverse the elements in each array. The code is as follows:
$fruit = array('apple', 'banana', 'orange'); $color = array('red', 'yellow', 'orange'); $combined = array($fruit, $color); foreach ($combined as $array) { foreach ($array as $item) { echo $item . ' '; } } //输出结果为:apple banana orange red yellow orange
In the above code, we put the $fruit and $color arrays that need to be traversed in the $combined array, and then use a foreach loop to traverse each array in the $combined array, and then use The foreach loop iterates through the elements in each array.
Method 2: Use multiple array functions
In addition to using foreach loop nesting, you can also use multiple array functions to traverse multiple arrays. For example, array_merge() function, array_replace() function, etc. The code is as follows:
$fruit1 = array('apple', 'banana', 'orange'); $fruit2 = array('pear', 'watermelon', 'pineapple'); $color = array('red', 'yellow', 'orange'); $merged = array_merge($fruit1, $fruit2, $color); foreach ($merged as $item) { echo $item . ' '; } //输出结果为:apple banana orange pear watermelon pineapple red yellow orange
In the above code, we use the array_merge() function to merge the $fruit1, $fruit2 and $color arrays into a new array $merged, and then use a foreach loop to traverse the $merged array of each element.
Method 3: Use the array_map() function
The array_map() function can merge elements at the same position in multiple arrays into a new array, and then use a foreach loop to traverse the new array. The code is as follows:
$fruit = array('apple', 'banana', 'orange'); $color = array('red', 'yellow', 'orange'); $merged = array_map(function ($f, $c) { return $f . ' ' . $c; }, $fruit, $color); foreach ($merged as $item) { echo $item . ' '; } //输出结果为:apple red banana yellow orange orange
In the above code, we use the array_map() function to merge the elements at the same position in the $fruit and $color arrays into a new array $merged, and then use a foreach loop to traverse the $merged array every element in .
Summary
The above are three ways to traverse multiple arrays in PHP. Which method to use depends on the specific needs. If you need to directly traverse the elements in multiple arrays, it is best to use the first method, which is to use a nested foreach loop. If you need to merge multiple arrays into a new array, it is more convenient to use the second method. If you need to merge elements at the same position in multiple arrays into a new array, the third method is more suitable.
The above is the detailed content of How to traverse multiple arrays in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
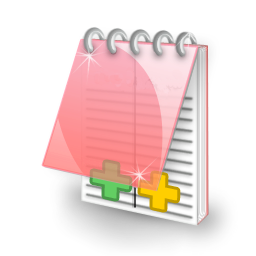
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
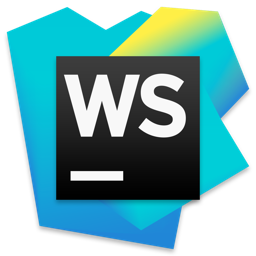
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
