In PHP, objects and arrays are two different data types. However, in some cases, we need to convert the object into an array for other operations. In this article, we will explore how to convert objects into arrays in PHP.
1. Original method in PHP
PHP provides a function get_object_vars() for returning the properties of the object. This function will return an associative array, the keys of the array are the property names of the object, and the values of the array are the values of the properties. The following is a sample code:
class myClass { public $name = 'Jenny'; private $age = 25; } $obj = new myClass(); $array = get_object_vars($obj); print_r($array);
Output:
Array ( [name] => Jenny )
With this method, we can obtain the public properties of an object, but cannot obtain them if they are private properties. So if we want to get all the properties of an object, we need to change the class definition to the following form:
class myClass { public $name = 'Jenny'; private $age = 25; public function toArray() { return get_object_vars($this); } } $obj = new myClass(); $array = $obj->toArray(); print_r($array);
Output:
Array ( [name] => Jenny [age] => 25 )
With this method, we can get all the properties of an object Properties, including private properties.
2. Use serialization and deserialization methods
Another way to convert an object into an array is to use PHP's serialization and deserialization functions. The serialize function serializes an object into a string, while the unserialize function converts a string back into an object. In this way we can convert the object into an array.
The following is a sample code:
class myClass { public $name = 'Jenny'; private $age = 25; } $obj = new myClass(); $serialize = serialize($obj); $array = unserialize($serialize); print_r($array);
Output:
myClass Object ( [name] => Jenny [age:myClass:private] => 25 )
We can see that this method converts the object into an array containing object information. If we only need the property values of the object, further processing of the array is required. The following is a modified sample code:
class myClass { public $name = 'Jenny'; private $age = 25; public function toArray() { $serialized = serialize($this); $array = unserialize($serialized); return get_object_vars($array); } } $obj = new myClass(); $array = $obj->toArray(); print_r($array);
Output:
Array ( [name] => Jenny [age] => 25 )
With this method, we can quickly convert the object into an array and obtain the object's property values.
3. Use the json_encode and json_decode methods
Another way to convert an object into an array is to use the json_encode and json_decode methods in PHP. json_encode converts objects into JSON format, while json_decode converts JSON-formatted strings back into arrays.
The following is a sample code:
class myClass { public $name = 'Jenny'; private $age = 25; } $obj = new myClass(); $json = json_encode($obj); $array = json_decode($json, true); print_r($array);
Output:
Array ( [name] => Jenny [age] => 25 )
We can see that this method quickly converts the object into an array and obtains the object's attribute value.
In summary, we have introduced several methods to convert objects in PHP into arrays. Each of these methods has its own advantages and disadvantages, and choosing the appropriate method depends on the specific application scenario. No matter which method you use, you need to have a deep understanding of the difference between PHP objects and arrays in order to implement your code logic correctly.
The above is the detailed content of How to convert object into array in php. For more information, please follow other related articles on the PHP Chinese website!
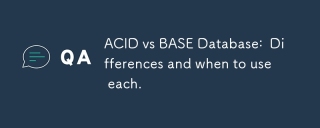
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
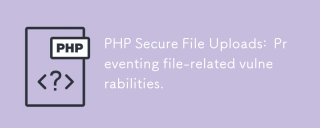
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
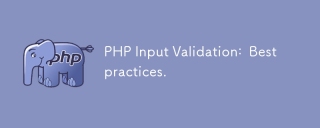
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
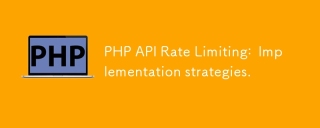
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
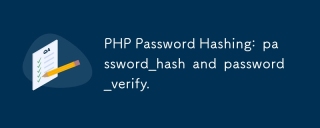
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
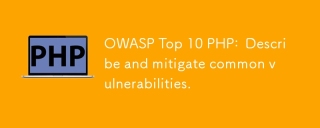
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
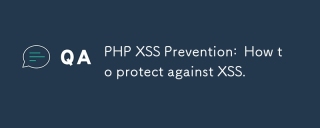
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
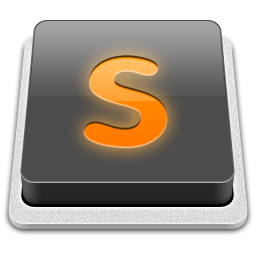
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor