Thread group in Java (ThreadGroup class)
The ThreadGroup class is used in Java to represent a thread group, which represents a collection of threads and can manage a batch of threads and thread groups. Threads can be assigned to a certain thread group. There can be thread objects in the thread group, there can also be thread groups, and there can also be threads in the group. This organizational structure is somewhat similar to the form of a tree, as shown in the figure.
All threads created by the user belong to the specified thread group. If no thread group is explicitly specified, the thread belongs to the default thread group (ie, main thread group). By default, the child thread and the parent thread are in the same thread group.
In addition, the thread group to which a thread belongs can only be specified when the thread is created. The thread group to which it belongs cannot be changed while the thread is running. That is to say, the thread group to which a thread belongs cannot be changed once it is specified.
2. Why use thread groups
1.Safety
Threads in the same thread group can modify each other's data. But if they are in different thread groups, then the data cannot be modified "cross-thread groups", and data security can be guaranteed to a certain extent.
2. Batch management
Threads or thread group objects can be managed in batches to effectively organize or control threads or thread group objects.
3. Thread group usage example
1. Thread association thread group: first-level association
The so-called first-level association means that the parent object has child objects, but no grandchild objects are created. For example, create a thread group, and then assign the created threads to the group to effectively manage these threads. The code example is as follows:
public class ThreadGroupTest { public static void main(String[] args) { ThreadGroup rootThreadGroup = new ThreadGroup("root线程组"); Thread thread0 = new Thread(rootThreadGroup, new MRunnable(), "线程A"); Thread thread1 = new Thread(rootThreadGroup, new MRunnable(), "线程B"); thread0.start(); thread1.start(); } } class MRunnable implements Runnable { @Override public void run() { while (!Thread.currentThread().isInterrupted()) { System.out.println("线程名: " + Thread.currentThread().getName() + ", 所在线程组: " + Thread.currentThread().getThreadGroup().getName()) ; try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } } 复制代码
The execution results are as follows:
线程名: 线程A, 所在线程组: root线程组 线程名: 线程B, 所在线程组: root线程组 复制代码
2. Thread association thread group: multi-level association
The so-called multi-level association means that the parent object has child objects, and creating a grandchild object in the child object will have the effect of descendants. For example, use the second construction method in the figure below to attribute the sub-thread group to a certain thread group, and then attribute the created thread to the sub-thread group. This will have the effect of a thread tree.
The code example is as follows:
public class ThreadGroupTest { public static void main(String[] args) { ThreadGroup rootThreadGroup = new ThreadGroup("root线程组"); Thread thread0 = new Thread(rootThreadGroup, new MRunnable(), "线程A"); Thread thread1 = new Thread(rootThreadGroup, new MRunnable(), "线程B"); thread0.start(); thread1.start(); ThreadGroup threadGroup1 = new ThreadGroup(rootThreadGroup, "子线程组"); Thread thread2 = new Thread(threadGroup1, new MRunnable(), "线程C"); Thread thread3 = new Thread(threadGroup1, new MRunnable(), "线程D"); thread2.start(); thread3.start(); } } class MRunnable implements Runnable { @Override public void run() { while (!Thread.currentThread().isInterrupted()) { System.out.println("线程名: " + Thread.currentThread().getName() + ", 所在线程组: " + Thread.currentThread().getThreadGroup().getName() + ", 父线程组: " + Thread.currentThread().getThreadGroup().getParent().getName()); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } } 复制代码
The execution results are as follows:
线程名: 线程A, 所在线程组: root线程组, 父线程组: main 线程名: 线程B, 所在线程组: root线程组, 父线程组: main 线程名: 线程C, 所在线程组: 子线程组, 父线程组: root线程组 线程名: 线程D, 所在线程组: 子线程组, 父线程组: root线程组 复制代码
3. Batch management of threads in the group
Naturally, using a thread group requires batch management of threads. For example, you can batch interrupt threads in the group. The code example is as follows:
public class ThreadGroupTest { public static void main(String[] args) { ThreadGroup rootThreadGroup = new ThreadGroup("root线程组"); Thread thread0 = new Thread(rootThreadGroup, new MRunnable(), "线程A"); Thread thread1 = new Thread(rootThreadGroup, new MRunnable(), "线程B"); thread0.start(); thread1.start(); ThreadGroup threadGroup1 = new ThreadGroup(rootThreadGroup, "子线程组"); Thread thread2 = new Thread(threadGroup1, new MRunnable(), "线程C"); Thread thread3 = new Thread(threadGroup1, new MRunnable(), "线程D"); thread2.start(); thread3.start(); rootThreadGroup.interrupt(); System.out.println("批量中断组内线程"); } } class MRunnable implements Runnable { @Override public void run() { while (!Thread.currentThread().isInterrupted()) { System.out.println("线程名: " + Thread.currentThread().getName() + ", 所在线程组: " + Thread.currentThread().getThreadGroup().getName() + ", 父线程组: " + Thread.currentThread().getThreadGroup().getParent().getName()); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); break; } } System.out.println(Thread.currentThread().getName() + "执行结束"); } } 复制代码
The execution result is as follows:
线程名: 线程A, 所在线程组: root线程组, 父线程组: main 线程名: 线程B, 所在线程组: root线程组, 父线程组: main 线程名: 线程C, 所在线程组: 子线程组, 父线程组: root线程组 线程名: 线程D, 所在线程组: 子线程组, 父线程组: root线程组 批量中断组内线程 线程A执行结束 线程B执行结束 线程C执行结束 线程D执行结束 复制代码
The above is the detailed content of Examples and methods of using thread groups in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
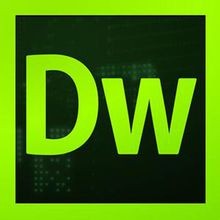
Dreamweaver CS6
Visual web development tools
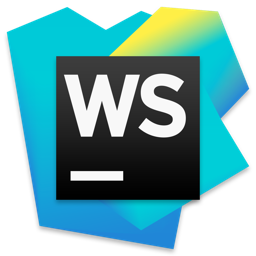
WebStorm Mac version
Useful JavaScript development tools
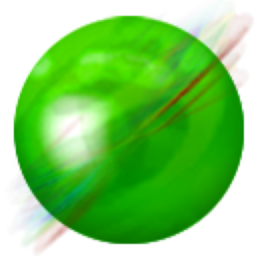
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor