


What are the principles and implementation methods of Java reflection mechanism?
1. Through reflection, we can build an instance, get the value of the member variable, get the method and call it.
You can also get annotations defined on member variables, methods, and method parameters.
Next, let’s look at the code implementation, and then talk about the principle.
1) Construct a parameterless instance: call the parameterless constructor through reflection
//1.通过全类名加载字节码对象 Class clazz = Class.forName("com.example.lib.Person"); //2.通过类的字节码拿到定义的构造函数 Constructor constructor = clazz.getConstructor(); //3.通过构造方法创建对象 Object obj = constructor.newInstance();
2) Construct a parameterized instance:
//1.通过全类名加载字节码对象 Class clazz = Class.forName("com.example.lib.Person"); //2.通过类的字节码拿到定义的构造函数 Constructor constructor = clazz.getConstructor(int.class,String.class); //3.通过构造方法创建对象 Object obj = constructor.newInstance(20,"xiaohua");
3) Obtain the value of the member variable through reflection .
//4.通过属性名获取属性 Field field = clazz.getDeclaredField("age"); field.setAccessible(true); //5.调用get方法拿到对象obj属性age的值 Integer age = (Integer) field.get(obj);
4) Call the object's method through reflection.
//4.通过方法名和参数类型,拿到方法 method = clazz.getMethod("setAge", int.class); //5.调用方法 obj是哪个对象身上的方法。 method.invoke(obj, 21); method = clazz.getMethod("getAge"); method.invoke(obj);
5). Obtain the value of static variables through reflection.
//1.通过全类名加载字节码对象 Class clazz = Class.forName("com.example.lib.Person"); //2.获取静态属性ID Field field = clazz.getField("ID"); field.setAccessible(true); //3.拿到静态属性ID的值。 // 因为静态变量存在方法区,在对象创建之前,就已经加装到了内存 //所以,没有对象,也可以获取变量的值,这里传null也是可以的。 int id = (int) field.get(null);
2. Obtain the value of the defined annotation through reflection
1) Obtain the annotation and value of the member variable.
@Target({ElementType.FIELD}) @Retention(RetentionPolicy.RUNTIME) public @interface BindView { int value(); }
public class MainActivity { @BindView(10000) TextView textView; }
//10通过反射拿到定义在属性上的注解 Class clazz = MainActivity.class; Field textView = clazz.getDeclaredField("textView"); BindView bindView = textView.getAnnotation(BindView.class); int txtId = bindView.value();
3) Obtain the annotations and values defined on the method and method parameters through reflection
@Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface Post { String value() default ""; }
public interface NetWorkInterface { @Post("http://www.baidu.com") Call getPerson(@Queue("name") String name, @Queue("200") int price); }
//11通过反射拿到方法上定义的注解 clazz = NetWorkInterface.class; Method method = clazz.getMethod("getPerson", String.class, int.class); //获取Post注解 Post post = method.getAnnotation(Post.class); //获取值 String url = post.value();
//12通过反射拿到参数上的注解 //为是个二维数组,因为方法参数会有多个,一个参数有可能定义多个注解 Annotation[][] annotations = method.getParameterAnnotations(); for (Annotation[] ans : annotations) { for (Annotation an : ans) { if (an instanceof Queue) { Queue queue = (Queue) an; String value = queue.value(); } } }
4) Obtain the parameter and return value types of the method.
//13.拿到方法参数的类型。 Type[] types = method.getGenericParameterTypes(); for (Type type : types) { System.out.println(type.toString()); } //14.获取方法返回值类型 Type type = method.getGenericReturnType();
3 Summary: Through reflection, you can get the values of member variables on the object, call methods, and obtain annotations defined on member variables, methods, and method parameters. Retrofit uses annotation plus reflection technology, and dynamic proxy (this technology will be shared later)
4. Through reflection, you can do the above things. What is the principle of reflection?
1) The source code we write is a .java file, which becomes a .class file after being compiled by javac, that is, a bytecode file.
2) When the program is executed, the JVM will class load the bytecode file into the memory. Strictly speaking, it is loaded into the method area and converted into a
java.lang.Class object. Everything is an object. Class is called a class object, which describes the data structure of a class in the metadata space, including the attributes, methods, constructors, annotations, interfaces and other information defined in the class.
The first step in all reflection is to get the class object Class object. Once you get the Class object, you also get everything defined in the class.
Class clazz = Class.forName("com.example.lib.Person");
This line of code loads the Person class into memory through the class loader , and get the corresponding Class object.
The above is the detailed content of What are the principles and implementation methods of Java reflection mechanism?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
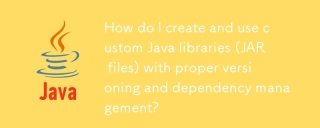
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
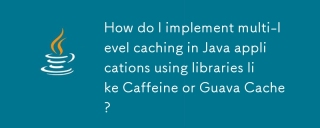
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
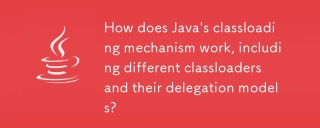
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
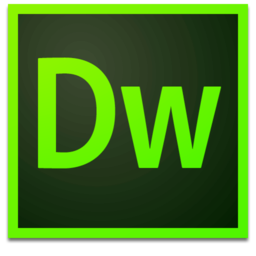
Dreamweaver Mac version
Visual web development tools
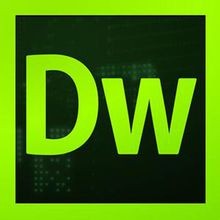
Dreamweaver CS6
Visual web development tools