JavaScript is a popular and powerful programming language that is often used for the development of websites, applications, and games. When making a website, graphic elements are an integral part. Many websites require the use of graphic elements in various shapes, such as lines, rectangles, circles, and semicircles. In this article, we will discuss how to make a half-circle graphic using JavaScript.
1. Understand the method of realizing a semicircle
Semicircular graphics are usually composed of three elements: circle, cutting line and cutting area. If you want to make a circle with half of it missing, you need to delete a semicircle from the entire circle and add a line for the cutout. Then, the remaining semicircle needs to be filled with the appropriate color.
2. Making a circle with half missing
In JavaScript, making a circle with half missing requires the following steps:
1. Create an HTML Canvas element.
2. Create a drawing context object.
3. Use the beginPath() method to start creating the path.
4. Use the arc() method to create a circular path.
5. Use the lineTo() method to add a cutting line path.
6. Use the closePath() method to end the path.
7. Use fill() or stroke() method to fill or stroke.
8. Set styles and add graphics to the page.
The following is a JavaScript code example to implement a circle with half of it missing:
let canvas = document.createElement('canvas'); let ctx = canvas.getContext('2d'); canvas.width = 200; canvas.height = 200; ctx.beginPath(); ctx.moveTo(canvas.width / 2, canvas.height / 2); ctx.arc( canvas.width / 2, canvas.height / 2, 100, (Math.PI / 2), -(Math.PI / 2), true ); ctx.lineTo(canvas.width / 2, canvas.height / 2); ctx.closePath(); ctx.fillStyle = 'red'; ctx.fill(); document.body.appendChild(canvas);
In this code, we first create a canvas element and use the getContext() method to obtain the context object. Then, we set the width and height of the canvas and start creating the path using the beginPath() method. Next, use the arc() method to create a circular path starting at 90 degrees (i.e. 1/2π) and ending at -90 degrees (i.e. -1/2π), indicating that we cut the top half. Finally, we use the lineTo() method to create a line connecting the circle and use the fillStyle property to fill the remaining circle portion with red.
3. Use SVG to realize a circle missing half of it
When using JavaScript to make a semicircle, there is another way to use SVG to achieve it. SVG (Scalable Vector Graphics) is an XML format used to describe vector-based graphics. Using SVG, you can easily create and edit various types of graphics, including semicircles and circles with half of them missing.
Here is a code example using SVG to create a missing half of a circle:
<svg> <path></path> </svg>
In this code, we first create a 200x200 pixel SVG element. Next, we create a path using its path element. The d attribute of a path describes the shape of the path. "M 100,100" means creating a path starting from coordinates (100,100). "a 100,100 0 1 1 -2,0 z" means using an elliptical arc path to draw a circle with half of it missing.
4. Summary
Semicircles are a common graphic element when making websites and applications, and JavaScript and SVG can easily implement circles with half of them missing. With the advancement of technology, web developers have many powerful tools to achieve various complex graphics and animation effects. However, for beginners, it is still very important to understand the basics and techniques. As you learn to make graphic elements, remember to stay focused on every step of the code and always strive for perfection.
The above is the detailed content of How to create missing semicircle graphics in javascript. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

Reactisafrontendlibrary,focusedonbuildinguserinterfaces.ItmanagesUIstateandupdatesefficientlyusingavirtualDOM,andinteractswithbackendservicesviaAPIsfordatahandling,butdoesnotprocessorstoredataitself.

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
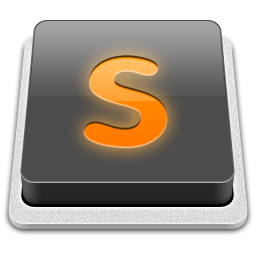
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor