PHP is a highly extensible programming language, and arrays are one of the most important data structures in PHP programming. In PHP, it is often necessary to convert array values to integer types for various calculations. This article will explain how to convert an array value to an integer type using functions in PHP.
- The intval() function in PHP
The intval() function in PHP can convert any value to an integer type. The syntax is as follows:
intval($value, $base);
Among them, $value represents the value to be converted, and $base represents the base to be used. If $base is not assigned a value, intval() will use decimal base for conversion.
When converting an array value to an integer type, we can use the intval() function. The following is a sample code:
$arr = array('1', '2', '3'); foreach($arr as $value){ $intval = intval($value); echo $intval . '<br>'; }
In the above code, we convert the values in the array $arr to integer types respectively, and use the echo statement to print each converted value. The output result is as follows:
1 2 3
As you can see, the intval() function successfully converted the array value into an integer type and output the correct result.
- array_map() function in PHP
In addition to using the foreach loop and intval() function, we can also use the array_map() function in PHP for conversion. The array_map() function is a very useful function that applies a callback function to each element in an array.
The following is a sample code that uses the array_map() function to convert an array value to an integer type:
$arr = array('1', '2', '3'); $int_arr = array_map('intval', $arr); print_r($int_arr);
In the above code, we use the array_map() function to convert each value in the array $arr The intval() function is applied to the elements to convert them to integer types. Then we use the print_r() function to output the entire array. The output result is as follows:
Array ( [0] => 1 [1] => 2 [2] => 3 )
As you can see, the array value can also be successfully converted to an integer type using the array_map() function.
Summary
In PHP, converting an array value to an integer type is very easy. We can use intval() function or array_map() function to achieve this purpose. Either way, each value in the array can be easily converted to an integer type for various calculations and operations.
The above is the detailed content of How to convert array value to integer in php. For more information, please follow other related articles on the PHP Chinese website!
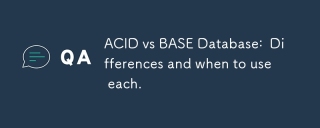
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
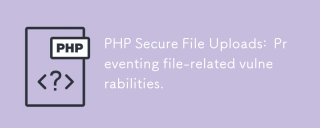
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
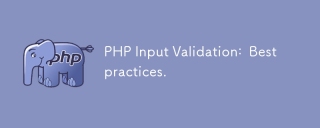
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
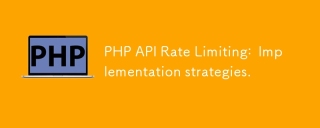
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
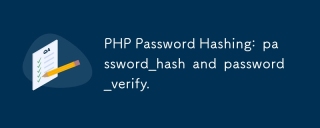
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
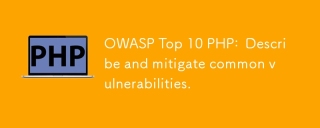
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
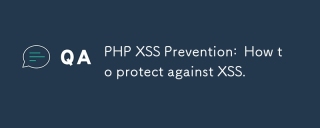
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
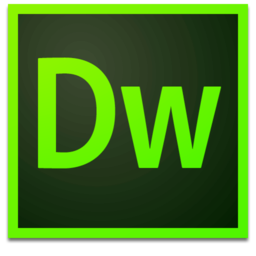
Dreamweaver Mac version
Visual web development tools
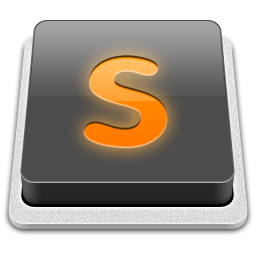
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
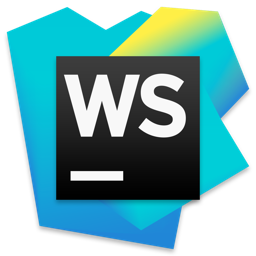
WebStorm Mac version
Useful JavaScript development tools