


When writing PHP programs, we often need to deal with arrays. Arrays in PHP can be single-dimensional or multi-dimensional, but in some cases, we need to convert multi-dimensional arrays into single-dimensional arrays. This article will show you how to convert a multi-dimensional array into a single-dimensional array using PHP.
1. Understanding multi-dimensional arrays and one-dimensional arrays
In PHP, a multi-dimensional array refers to an array that contains other arrays. That is to say, each element in the multi-dimensional array is an array. For example, the following is a two-dimensional array:
$fruit = array( 'apple' => array('type' => 'fruit', 'taste' => 'sweet'), 'banana' => array('type' => 'fruit', 'taste' => 'sweet'), 'carrot' => array('type' => 'vegetable', 'taste' => 'bitter') );
If you want to convert this two-dimensional array to a one-dimensional array, you can use array_merge_recursive() or a foreach loop to achieve this.
2. Use array_merge_recursive() function
array_merge_recursive() function can be used to merge multi-dimensional arrays. This function merges multiple arrays into a single array, retaining all values for the same key. For example, if you have two arrays with the same key "apple" and one contains "taste" and "color" information and the other contains "type" and "attribute" information, array_merge_recursive() will retain the All values, i.e.:
$fruit = array( 'apple' => array('taste' => 'sweet', 'color' => 'red'), 'banana' => array('taste' => 'sweet', 'color' => 'yellow') ); $vegetable = array( 'apple' => array('type' => 'fruit', 'attribute' => 'delicious'), 'carrot' => array('type' => 'vegetable', 'attribute' => 'healthy') ); $merged = array_merge_recursive($fruit, $vegetable);
The result will be a multi-dimensional array $merged, as shown below:
$array = Array ( [apple] => Array ( [taste] => Array ( [0] => sweet [1] => Array ( [0] => fruit ) ) [color] => red [attribute] => delicious ) [banana] => Array ( [taste] => sweet [color] => yellow ) [carrot] => Array ( [type] => vegetable [attribute] => healthy ) )
Since the array merged using the array_merge_recursive() function is a multi-dimensional array, you still need Use a foreach loop to convert it to a one-dimensional array.
3. Use the foreach loop
Using the foreach loop to convert a multi-dimensional array into a one-dimensional array is more flexible than using the array_merge_recursive() function. The basic idea of using a foreach loop to convert a multidimensional array to a one-dimensional array is to copy each value in the multidimensional array to a new one-dimensional array. For example, for the $fruit array above, you can convert it to a one-dimensional array using the following code:
$flat = array(); foreach($fruit as $key => $value) { if(is_array($value)) { $flat = array_merge($flat, array_flatten($value)); } else { $flat[$key] = $value; } }
This code uses recursion and the array_merge() function to flatten a multi-dimensional array. The array_flatten() function is defined as follows:
function array_flatten($array) { $result = array(); foreach($array as $key => $value) { if (is_array($value)) { $result = array_merge($result, array_flatten($value)); } else { $result[$key] = $value; } } return $result; }
This function calls itself recursively to convert a multi-dimensional array into a one-dimensional array.
4. Summary
Converting a multi-dimensional array to a one-dimensional array is a basic task in PHP development. In this article, we covered two methods: using the array_merge_recursive() function and using a foreach loop. Regardless of which method you choose, converting a multidimensional array to a one-dimensional array is a useful skill.
The above is the detailed content of How to convert multi-dimensional array into one-dimensional array in php. For more information, please follow other related articles on the PHP Chinese website!
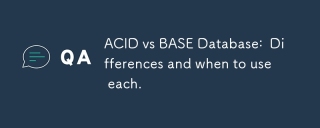
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
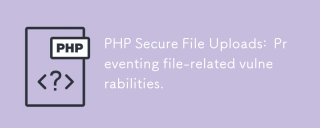
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
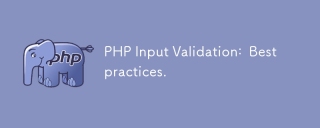
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
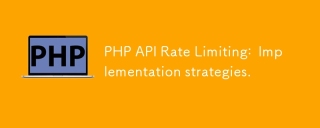
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
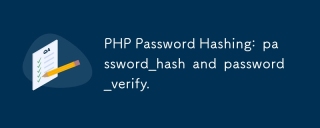
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
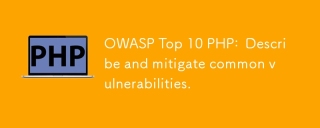
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
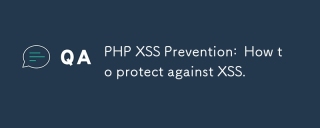
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
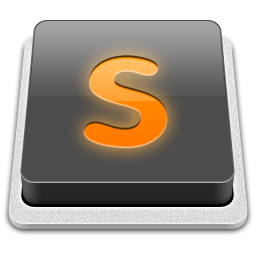
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
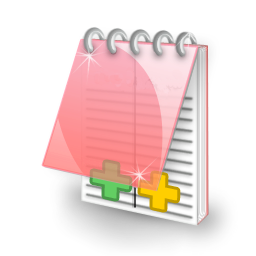
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.