JSON (JavaScript Object Notation) is a lightweight data exchange format. It uses human-readable text to transfer and store data objects. Unlike XML, JSON is easier to parse and process, so it is often used when transferring and exchanging data between web applications and servers.
In PHP, you can use the built-in json_decode function to convert a JSON string into a PHP object. For example, the following example parses a JSON string into a PHP object:
<?php $json_string = '{"name":"John", "age":30, "city":"New York"}'; $obj = json_decode($json_string); echo $obj->name; //输出 John echo $obj->age; //输出 30 echo $obj->city; //输出 New York ?>
This function accepts two parameters: the JSON string to be parsed and a Boolean variable indicating to convert the parsed JSON object into a PHP object ( Default is false) or associative array (true).
However, if the JSON data contains an array of objects or an array of objects, it will need to be processed using a recursive function. Here is an example where the JSON data contains nested object arrays and object arrays:
{ "employees": [ { "name": "John Doe", "email": "john@example.com", "phones": [ { "type": "home", "number": "555-555-1234" }, { "type": "work", "number": "555-555-5678" } ] }, { "name": "Jane Smith", "email": "jane@example.com", "phones": [ { "type": "home", "number": "555-555-4321" }, { "type": "work", "number": "555-555-8765" } ] } ] }
To parse such data, you can write a recursive function that iterates through the entire JSON object and converts it to a PHP object or array. Here is an example function that handles JSON object arrays, object arrays, and standard JSON objects:
$value) { if (is_object($value)) { $result[$key] = json_to_array($value); } else if (is_array($value)) { $result[$key] = []; foreach ($value as $item) { $result[$key][] = json_to_array($item); } } else { $result[$key] = $value; } } return $result; } $json_string = '{ "employees": [ { "name": "John Doe", "email": "john@example.com", "phones": [ { "type": "home", "number": "555-555-1234" }, { "type": "work", "number": "555-555-5678" } ] }, { "name": "Jane Smith", "email": "jane@example.com", "phones": [ { "type": "home", "number": "555-555-4321" }, { "type": "work", "number": "555-555-8765" } ] } ] }'; $obj = json_decode($json_string); $array = json_to_array($obj); print_r($array); ?>
This function will return a PHP array containing the JSON data for all nested object arrays and objects.
The above is the detailed content of How PHP parses JSON data. For more information, please follow other related articles on the PHP Chinese website!
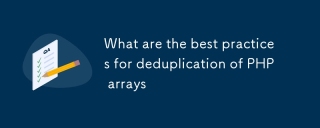
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
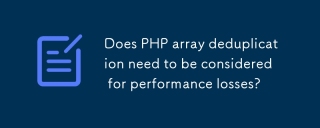
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
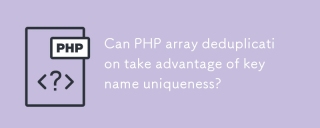
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
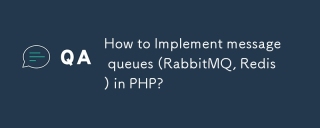
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
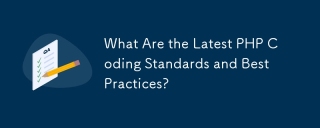
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
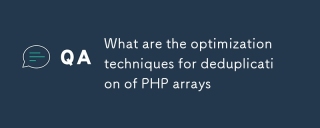
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
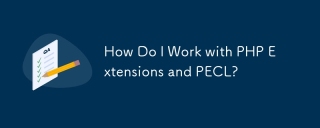
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
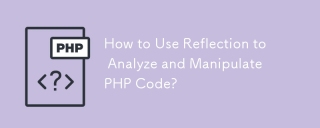
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
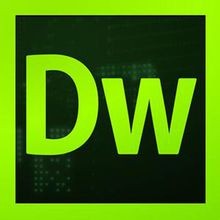
Dreamweaver CS6
Visual web development tools
