PHP is a scripting language widely used in web development and provides a wealth of built-in functions and features. Among them, the functions of processing strings and arrays are very important. In this article, we will focus on how PHP splits a string array.
First, let us understand the definition and examples of string arrays in PHP:
$stringArray = array("apple,banana,cherry,date,elderberry");
There are 5 elements in this string array (each element is separated by commas), representing 5 different fruits. Next we will introduce two ways to split this string array.
1. Use the explode() function
The explode() function is one of the basic functions for processing strings in PHP. It is used to split a string into multiple substrings and return An array of these substrings.
In this example, we can use the explode() function to split each element into a separate string:
$stringArray = array("apple,banana,cherry,date,elderberry"); $newArray = array(); foreach ($stringArray as $fruitString) { $fruits = explode(",", $fruitString); foreach ($fruits as $fruit) { $newArray[] = $fruit; } } print_r($newArray);
In the above code, we use a foreach loop to iterate through each element (i.e. every watermelonString). In each loop, we use the explode() function to split the element into multiple substrings by commas, and again use the foreach loop to iterate through each substring and add it to the new array $newArray.
Result output:
Array ( [0] => apple [1] => banana [2] => cherry [3] => date [4] => elderberry )
2. Using regular expressions
In PHP, you can also use regular expressions to process strings. Regular expressions provide a more flexible method of pattern matching.
In this case, we can use the preg_split() function to match commas and split the fruits in each element into separate strings:
$stringArray = array("apple,banana,cherry,date,elderberry"); $newArray = array(); foreach ($stringArray as $fruitString) { $fruits = preg_split("/\s*,\s*/", $fruitString); foreach ($fruits as $fruit) { $newArray[] = $fruit; } } print_r($newArray);
In the above code, we Use the preg_split() function to split the fruits in each element into multiple substrings by commas, and use the foreach loop again to traverse each substring and add it to the new array $newArray.
Result output:
Array ( [0] => apple [1] => banana [2] => cherry [3] => date [4] => elderberry )
The advantage of using regular expressions is that it can use a more flexible pattern matching method, so in some cases, regular expressions may be better than explode( ) function is more suitable.
In this article, we introduced how to split a string array in PHP. Regardless of which method is used, our goal is to separate each element and add it to a new array. In actual development, which method you choose depends on your needs and personal preferences.
The above is the detailed content of How to split string array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
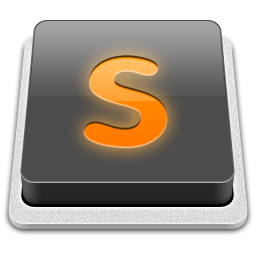
SublimeText3 Mac version
God-level code editing software (SublimeText3)
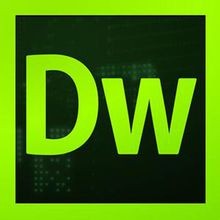
Dreamweaver CS6
Visual web development tools
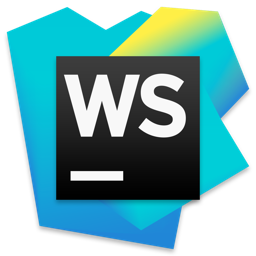
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
