In many scenarios, we need to verify whether the time and date are in the correct format, and whether the time conforms to the convention.
1. Verify the date in yyyy-MM-dd HH:mm:dd format
String date = "2020-01-25 12:36:45"; System.out.println("date "+isLegalDate(date.length(),date,"yyyy-MM-dd HH:mm:ss"));
2. Verify the date in yyyy-MM-dd format
String yearMonthday = "2020-01-01"; System.out.println("yearMonthday: "+isLegalDate(yearMonthday.length(),yearMonthday,"yyyy-MM-dd"));
3. Verify the date in yyyy-MM format
String yearMonth = "2020-02"; System.out.println("yearMonth: "+isLegalDate(yearMonth.length(),yearMonth,"yyyy-MM"));
4. Verify the date in yyyy format
String year = "2020"; System.out.println("year: "+isLegalDate(year.length(),year,"yyyy"));
5. Verify the date in HH:mm:ss format
String hms = "12:36:89"; System.out.println("hms: "+isLegalDate(hms.length(),hms,"HH:mm:ss"));
6. The following is a complete method class that can be run directly to verify whether the date format is correct
package com.shucha.deveiface.biz.test; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; /** * @author tqf * @Description 时间格式校验 * @Version 1.0 * @since 2020-09-15 16:49 */ public class IsLegalDate { public static void main(String[] args) { //1、验证 yyyy-MM-dd HH:mm:dd 格式的日期 String date = "2020-01-25 12:36:45"; System.out.println("date "+isLegalDate(date.length(),date,"yyyy-MM-dd HH:mm:ss")); //2、验证 yyyy-MM-dd 格式的日期 String yearMonthday = "2020-01-01"; System.out.println("yearMonthday: "+isLegalDate(yearMonthday.length(),yearMonthday,"yyyy-MM-dd")); //3、验证 yyyy-MM 格式的日期 String yearMonth = "2020-02"; System.out.println("yearMonth: "+isLegalDate(yearMonth.length(),yearMonth,"yyyy-MM")); //4、验证 yyyy 格式的日期 String year = "2020"; System.out.println("year: "+isLegalDate(year.length(),year,"yyyy")); //5、验证 HH:mm:ss 格式的日期 String hms = "12:36:89"; System.out.println("hms: "+isLegalDate(hms.length(),hms,"HH:mm:ss")); } /** * 根据时间 和时间格式 校验是否正确 * @param length 校验的长度 * @param sDate 校验的日期 * @param format 校验的格式 * @return */ public static boolean isLegalDate(int length, String sDate,String format) { int legalLen = length; if ((sDate == null) || (sDate.length() != legalLen)) { return false; } DateFormat formatter = new SimpleDateFormat(format); try { Date date = formatter.parse(sDate); return sDate.equals(formatter.format(date)); } catch (Exception e) { return false; } } }
The following is a screenshot after time verification
The above is the detailed content of How to verify whether the time format is correct in Java. For more information, please follow other related articles on the PHP Chinese website!
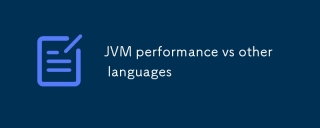
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
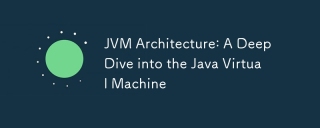
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
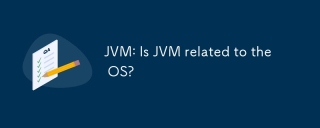
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
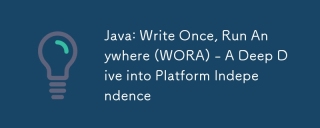
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
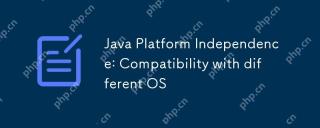
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
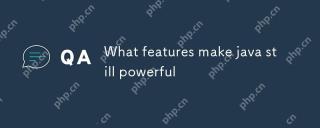
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
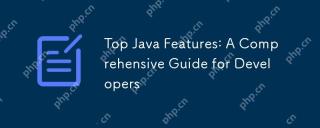
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
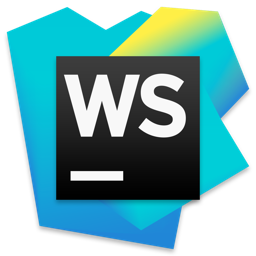
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
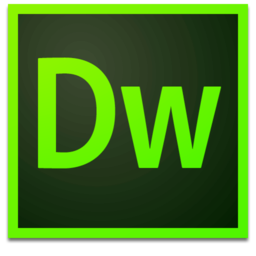
Dreamweaver Mac version
Visual web development tools
