PHP is a powerful and widely used programming language that is often used to develop web applications. In PHP, array is an important data type used to store and process a group of related data.
In PHP, we often need to check if an array is empty, sometimes we need to know if it has no elements at all, and sometimes we just need to know if it has elements but all the elements are null or false. In this article, we will discuss how to tell if a PHP array is empty and explore which method to use in different situations.
Let’s take a look at a simple PHP array first:
$fruits = ["apple", "banana", "orange"];
This is an array containing three elements, it is not an empty array. We can use the following simple method to determine whether an array is empty:
if (empty($fruits)) { echo "数组为空"; } else { echo "数组不为空"; }
Here we use PHP's built-in empty() function to determine whether $fruits is empty. If $fruits is an empty array, that is, there are no elements, then the empty() function will return true and print "the array is empty", otherwise it will return false and print "the array is not empty". In this example, $fruits is not an empty array, so "array is not empty" will be printed.
Please note that the empty() function is ideal for checking whether a defined variable has been assigned a value of null, zero, false, an empty string, or an empty array. It can easily determine whether a variable is "null".
Next, let’s look at another example, assuming we have an empty array:
$empty_array = [];
Now we want to use the empty() function to check whether $empty_array is empty:
if (empty($empty_array)) { echo "数组为空"; } else { echo "数组不为空"; }
Since $empty_array is an empty array, the empty() function returns true and "array is empty" will be printed. This is because the empty() function considers an empty array to be a "null value" situation.
However, please note that in some cases, we need to determine whether an array has no elements at all, that is, it has neither keys nor values. The above method may treat an array containing null, false, zero or empty string as non-empty, for example:
$falsey_array = [null, false, "", 0]; if (empty($falsey_array)) { echo "数组为空"; } else { echo "数组不为空"; }
Here $falsey_array contains null, false, empty string and the number 0, but empty( ) function treats it as a non-empty array and prints "array is not empty".
To determine whether an array has no elements, we can use the count() function, which will count the number of elements in the array. If the count() function returns 0, the array is empty. For example:
if (count($empty_array) == 0) { echo "数组为空"; } else { echo "数组不为空"; }
Since $empty_array does not contain any elements, the count() function returns 0 and prints "array is empty".
Similarly, if we want to check an array that has neither keys nor values, we can use the following method:
function is_empty_array($array) { if (count($array) == 0 && array_keys($array) === []) { return true; } return false; } if (is_empty_array($empty_array)) { echo "数组为空"; } else { echo "数组不为空"; }
Here we define a function called is_empty_array(), which The function accepts an array as argument and uses the count() function to check if there is an element and the array_keys() function to check if the array has any keys. If the count() function returns 0, we also need to check that the array_keys() function returns an empty array. This will ensure that only arrays with neither keys nor values are treated as "really" empty arrays.
To sum up, judging whether a PHP array is empty depends on the defined empty array. If you just need to check whether the array has a value, the empty() function is preferred because it can recognize null, false, 0, empty string and empty array.
If we need to determine an empty array without keys and values, use the count() function to count the number of elements in the array, and use the array_keys() function to check whether it is empty. In some cases, it is necessary to define the is_empty_array() function, which can check whether the array is really empty.
In actual work, we need to choose different ways to determine whether the PHP array is empty according to the actual situation. This will help ensure the correctness and reliability of the code, and improve the quality and efficiency of the code.
The above is the detailed content of How to determine if an array is empty in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
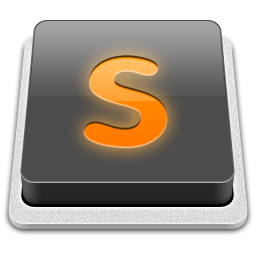
SublimeText3 Mac version
God-level code editing software (SublimeText3)
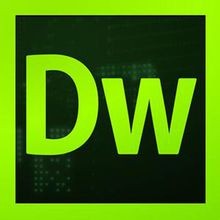
Dreamweaver CS6
Visual web development tools
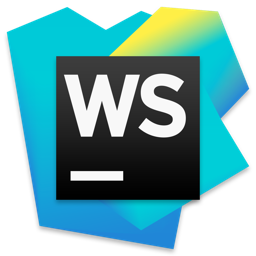
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
