Laravel is a popular PHP framework that provides many useful functions and tools, one of the important features is filling data. Filling data refers to filling the data in the database table with some predefined values for testing and development. This article will introduce how to use Laravel's fill data function.
1. Preparation
Before using Laravel's data filling function, you need to create a database table and an Eloquent model. The following is a simple example:
php artisan make:model User -m
The above command will create a User
model and a database migration file xxxx_xx_xx_xxxxxx_create_users_table.php# in the
app directory. ##. We need to define the table structure in the migration file:
public function up() { Schema::create('users', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); }Then run the database migration:
php artisan migrateThis will create a data table named
users.
db:seed command for filling data. We can create a Seeder class in the
database/seeds directory, inherit the
Illuminate\Database\Seeder class, and implement the
run method.
UserSeeder.php:
use Illuminate\Database\Seeder; use App\User; class UserSeeder extends Seeder { public function run() { factory(User::class, 50)->create(); } }The above code uses Laravel’s factory pattern to generate 50
User models, and store them into the database.
run method, we can use Laravel's query builder and Eloquent model to operate the database. In this example, we use the
factory function to create 50
User model instances and save them to the database.
database/seeds/DatabaseSeeder.php file. This class is a main seeder, which is used to call other seeder classes.
use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { public function run() { $this->call(UserSeeder::class); } }After calling the
UserSeeder class, we can execute the
db:seed command through the command line to fill in the data:
php artisan db:seedThis command will be found automatically All defined
Seeder classes and run their
run methods.
User model instances. Laravel's factory pattern can help us easily generate the data required for testing and development.
make and
create methods. The
make method is used to create a temporary model instance that is not saved to the database, and the
create method is used to create a model instance that is saved to the database.
Factory class to define, register and use factories. The use of the
Factory class is very simple. We only need to create a factory file in the
database/factories directory and define a model and its attributes through the
define method. Here is a simple example
UserFactory.php:
use Faker\Generator as Faker; use App\User; $factory->define(User::class, function (Faker $faker) { return [ 'name' => $faker->name, 'email' => $faker->unique()->safeEmail, 'password' => bcrypt('password'), ]; });In this example, we use the
Faker class to generate random model data and simulate real users data. This factory defines three attributes:
name,
email, and
password.
use App\User; $user = factory(User::class)->create();This will create a new
User model instance and save it to the database . We can also use the
make method to create a model instance that is not saved to the database:
use App\User; $user = factory(User::class)->make();This model instance will contain randomly generated attribute values, which we can use in testing and development . 5. SummaryLaravel provides a convenient data filling function. We can use Seeder to generate test data and use factory mode to generate model instances. With this feature, we can easily populate database tables for easy testing and development.
The above is the detailed content of How to use Laravel's fill data function. For more information, please follow other related articles on the PHP Chinese website!

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
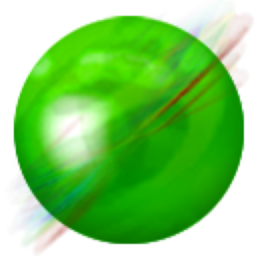
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
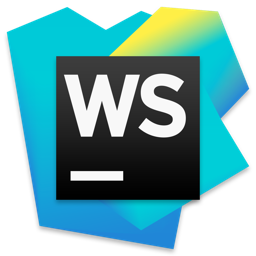
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor