With the continuous development of Internet technology, multimedia content has become an indispensable part of the Internet. In today's Internet world, video content and image content have become the most important display methods in websites and applications, which makes the processing of images and videos a problem that developers must face. In this regard, PHP, as a very popular web development language, has a wide range of applications in processing videos and pictures. This article will introduce how to implement video and picture replacement in PHP.
1. PHP video processing
To process videos in PHP, you need to use the FFmpeg library. FFmpeg is an open source cross-platform multimedia processing library that can implement functions such as audio and video encoding and decoding, format conversion, and processing. Using FFmpeg, we can easily process videos, such as file format conversion, video screenshots, video transcoding, etc.
- Installing FFmpeg
Before you start using FFmpeg, you need to install it. In Linux, installation can be done through the terminal. Open a terminal window and use the following command:
sudo apt-get install ffmpeg
- Video screenshot
Generally, we need to extract a certain frame from the video, which is usually used as A thumbnail or preview image of the video. To do this in PHP, you need to execute the following command:
<?php $video_path = 'video.mp4'; // 视频文件路径 $output_path = 'output.jpg'; // 输出截图文件路径 $time = 10; // 截图的时间点(秒) $cmd = "ffmpeg -ss " . $time . " -i " . $video_path . " -y -vframes 1 -q:v 2 " . $output_path; $output = shell_exec($cmd); echo "截图完成!"; ?>
Explain the above code:
-
$video_path
: Indicates the path where the video file is located; -
$output_path
: Indicates the output path of the screenshot file; -
$time
: Indicates which time point the screenshot is taken from the video; -
$cmd
: Indicates the conversion command to be executed; -
shell_exec()
: Indicates using the shell to execute the command.
- Video transcoding
Video transcoding is the process of converting a video file from one codec format to another codec format. Often, the video's codec format may not support playback on some devices, so it needs to be transcoded in PHP. To transcode video in PHP, you can execute the following code:
<?php $video_path = 'video.mp4'; // 要转换的视频路径 $output_path = 'converted-video.mp4'; // 转换后视频的输出路径 $cmd = "ffmpeg -i " . $video_path . " -c:a copy -vf scale=640:480 -c:v libx264 " . $output_path; $output = shell_exec($cmd); echo "视频转码完成!"; ?>
The conversion command corresponding to the above code:
ffmpeg -i video.mp4 -c:a copy -vf scale=640:480 -c:v libx264 converted-video.mp4
Among them, -c:a copy
represents audio Copy directly without recoding. -vf scale=640:480
means changing the resolution of the video to 640x480. -c:v libx264
means using h264 encoding.
2. PHP processing pictures
To process pictures in PHP, you can use the GD extension. The GD extension is a library for processing images in PHP that can generate, edit and output various types of images. Using the GD library, we can easily achieve image cropping, scaling, watermarking, etc.
- Install the GD extension
Before you start using the GD extension, you need to install it into PHP. If you are using a Linux system, you can install it through the following command:
sudo apt-get install php-gd
- Picture cropping
We often need to crop pictures, you can use the following code:
<?php $src_path = 'image.jpg'; // 原始图片路径 $output_path = 'cropped-image.jpg'; // 输出裁剪后的图片路径 // 创建图片资源 $src_img = imagecreatefromjpeg($src_path); // 获取裁剪后的图片 $cropped_img = imagecrop($src_img, ['x' => 10, 'y' => 10, 'width' => 100, 'height' => 100]); // 保存裁剪后的图片 imagejpeg($cropped_img, $output_path); // 释放内存 imagedestroy($src_img); imagedestroy($cropped_img); echo "图片裁剪完成!"; ?>
- Picture scaling
Using the GD library, you can also easily achieve image scaling. You can use the following code:
<?php $src_path = 'image.jpg'; // 原始图片路径 $output_path = 'resized-image.jpg'; // 输出缩放后的图片路径 $size = 0.5; // 图片缩放比例 // 创建图片资源 $src_img = imagecreatefromjpeg($src_path); // 获取缩放后的宽高 $dst_w = imagesx($src_img) * $size; $dst_h = imagesy($src_img) * $size; // 创建缩放后的图片资源 $dst_img = imagecreatetruecolor($dst_w, $dst_h); // 执行缩放 imagecopyresampled($dst_img, $src_img, 0, 0, 0, 0, $dst_w, $dst_h, imagesx($src_img), imagesy($src_img)); // 保存缩放后的图片 imagejpeg($dst_img, $output_path); // 释放内存 imagedestroy($src_img); imagedestroy($dst_img); echo "图片缩放完成!"; ?>
- Adding watermarks to pictures
We often need to add watermarks to pictures to protect the copyright information of the picture or as an identification of the picture. Using the GD library, you can also easily add watermarks to images. You can use the following code to add watermarks to pictures:
<?php $src_path = 'image.jpg'; // 原始图片路径 $output_path = 'watermarked-image.jpg'; // 输出加水印后的图片路径 $watermark_path = 'watermark.png'; // 水印图片路径 // 创建图片资源 $src_img = imagecreatefromjpeg($src_path); $watermark_img = imagecreatefrompng($watermark_path); // 获取水印图片的宽高 $w_w = imagesx($watermark_img); $w_h = imagesy($watermark_img); // 获取原始图片的宽高 $s_w = imagesx($src_img); $s_h = imagesy($src_img); // 计算水印图片在原始图片上的位置 $x = $s_w - $w_w - 10; $y = $s_h - $w_h - 10; // 合成图片 imagecopy($src_img, $watermark_img, $x, $y, 0, 0, $w_w, $w_h); // 保存加水印后的图片 imagejpeg($src_img, $output_path); // 释放内存 imagedestroy($src_img); imagedestroy($watermark_img); echo "图片加水印完成!"; ?>
Summary
This article introduces how to process videos and pictures in PHP. By using the FFmpeg library and GD extension, we can easily implement the processing of multimedia content. Of course, in actual applications, there may be more complex and specific requirements, which need to be adjusted and optimized according to the actual situation. I hope this article can provide you with some help and reference so that you can better apply PHP in development.
The above is the detailed content of How to implement video image replacement in PHP. For more information, please follow other related articles on the PHP Chinese website!
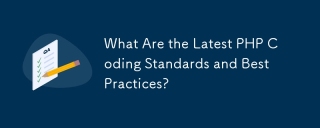
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
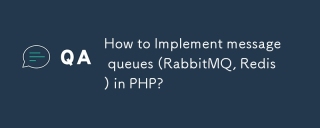
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
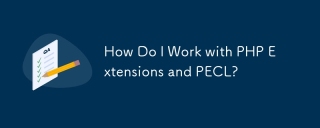
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
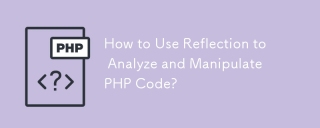
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
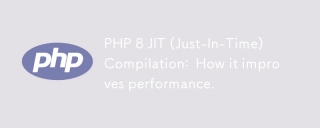
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
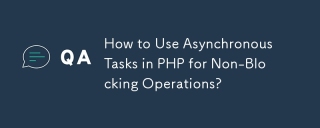
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
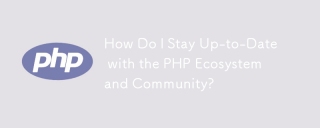
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
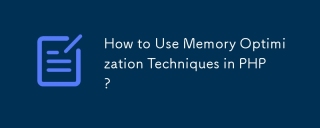
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
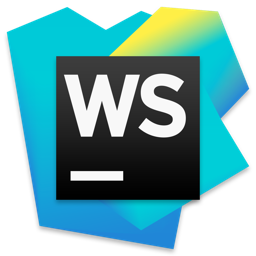
WebStorm Mac version
Useful JavaScript development tools
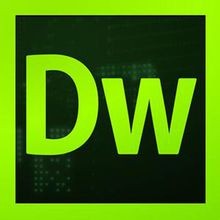
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
