In PHP, you can split a string or numeric variable into an array through some simple methods. This process is very practical as it allows us to split strings and numbers into multiple elements to better manipulate them. This article will introduce you to some methods for splitting a string or numeric variable into an array, provided you are familiar with PHP first.
1. Use the str_split function to split a string
The str_split() function can convert a string into an array. Here is an example of using this function:
<?php $str = "This is an example string."; $chars = str_split($str); print_r($chars); ?>
Output result:
Array ( [0] => T [1] => h [2] => i [3] => s [4] => [5] => i [6] => s [7] => [8] => a [9] => n [10] => [11] => e [12] => x [13] => a [14] => m [15] => p [16] => l [17] => e [18] => [19] => s [20] => t [21] => r [22] => i [23] => n [24] => g [25] => . )
In the above example, we passed a string variable $str
to str_split()
function. This function returns an array $chars
containing each character in the string.
2. Use the explode function to split the string
explode() function can also convert a string into an array. This function uses the delimiter specified in the first parameter to split the string. Here is an example using the explode function:
<?php $str = "one,two,three,four"; $parts = explode(",", $str); print_r($parts); ?>
Output:
Array ( [0] => one [1] => two [2] => three [3] => four )
In the above example, we passed a string containing comma delimiters to the explode() function. This function returns a new array containing the strings before and after each delimiter $parts
.
3. Use the range function to generate a number array
The range() function generates an array containing numbers within the specified range. The following is an example of using the range() function:
<?php $numbers = range(1,5); print_r($numbers); ?>
Output result:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
In the above example, we passed two numeric parameters to the range() function, which returns a A new array $numbers
containing the numbers 1
through 5
.
4. Use the sscanf function to parse strings
The sscanf() function can parse a formatted string into multiple values in order to convert it into an array. The following is an example of using the sscanf() function:
<?php $str = 'first:1 second:2 third:3'; $vars = sscanf($str, '%s:%d %s:%d %s:%d'); print_r($vars); ?>
Output result:
Array ( [0] => first [1] => 1 [2] => second [3] => 2 [4] => third [5] => 3 )
In the above example, we define a format string $str
, Each value consists of a string and a number, and by using the sscanf() function, we parse each value of $str
into a new array $vars
.
5. Use the array_map function
array_map() function can apply a function to each element of the array and return a new array containing the result. The following is an example of using the array_map() function:
<?php $numbers = array(1,2,3,4,5); $squares = array_map(function($n) { return $n * $n; }, $numbers); print_r($squares); ?>
Output result:
Array ( [0] => 1 [1] => 4 [2] => 9 [3] => 16 [4] => 25 )
In the above example, we use an anonymous function to calculate the square of the array $numbers
value. By using the array_map() function, we can apply the anonymous function to each element of the $numbers
array and return a new array containing the result $squares
.
Summary
The above are five ways to split variables into arrays using PHP. These methods allow us to more easily manipulate numeric and string variables and convert them into arrays of multiple elements. Whether you're working with text strings or numeric patterns, you can use these methods to solve your problem.
The above is the detailed content of How to split variables into arrays in php. For more information, please follow other related articles on the PHP Chinese website!
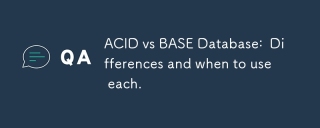
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
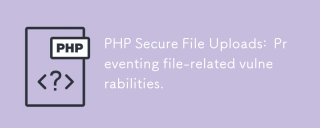
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
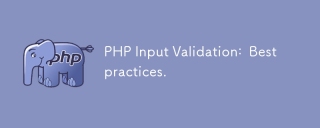
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
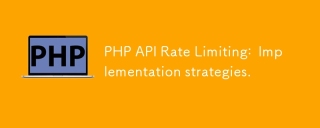
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
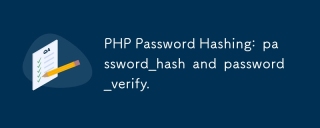
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
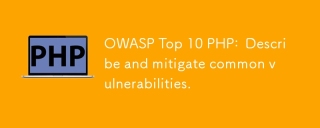
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
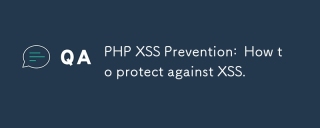
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
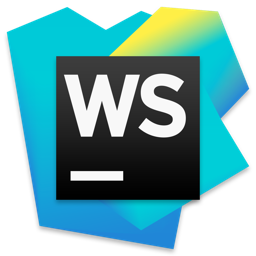
WebStorm Mac version
Useful JavaScript development tools
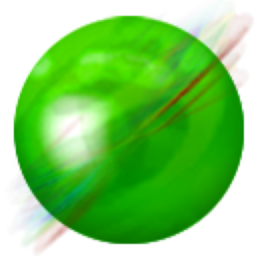
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment