


PHP is a very popular programming language that can handle database operations very well. In actual projects, we sometimes need to query the data in the database before 11 o'clock that day. So how to achieve it?
1. Get the current date and 11 o'clock timestamp
Use the PHP built-in function time()
to get the current timestamp. We can calculate the timestamp of 11 o'clock that day.
$current_time = time(); // 当前时间戳 $eleven_time = strtotime(date('Y-m-d 11:00:00')); // 今天11点的时间戳
2. Connect to the database
In PHP, you can use the PDO (PHP Data Objects)
or mysqli
function to connect to the database. Here we take PDO as an example to connect.
$dsn = 'mysql:host=localhost;dbname=test'; $username = 'root'; $password = '123456'; $options = array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION); try { $pdo = new PDO($dsn, $username, $password, $options); } catch(PDOException $e) { echo '连接失败:' . $e->getMessage(); exit; }
3. Query the data before 11 o'clock on the day
With the above two steps, we can start querying the database.
$sql = "SELECT * FROM `table` WHERE `create_time` prepare($sql); $stmt->bindParam(':eleven_time', $eleven_time); $stmt->execute(); $data = $stmt->fetchAll(PDO::FETCH_ASSOC);
We pass the 11 o'clock timestamp as a parameter to the SQL statement, and use the operator to query all data in the table that was created before 11 o'clock that day.
4. Complete code
The complete code is as follows:
$current_time = time(); // 当前时间戳 $eleven_time = strtotime(date('Y-m-d 11:00:00')); // 今天11点的时间戳 $dsn = 'mysql:host=localhost;dbname=test'; $username = 'root'; $password = '123456'; $options = array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION); try { $pdo = new PDO($dsn, $username, $password, $options); } catch(PDOException $e) { echo '连接失败:' . $e->getMessage(); exit; } $sql = "SELECT * FROM `table` WHERE `create_time` prepare($sql); $stmt->bindParam(':eleven_time', $eleven_time); $stmt->execute(); $data = $stmt->fetchAll(PDO::FETCH_ASSOC); print_r($data);
5. Summary
Through the above code, we can very conveniently query the database for 11 days of the day data before the point. PDO is used here to connect to the database, which is not only safe and reliable, but also more convenient to write code. Of course, if you are used to using mysqli functions, you can also try it.
It is worth noting that in actual projects, we should prevent SQL injection. Both PDO and mysqli provide measures such as prepared statements to prevent SQL injection. We should pay attention to this when writing code.
The above is the detailed content of How to query the data in the table before 11 o'clock on the same day in php. For more information, please follow other related articles on the PHP Chinese website!
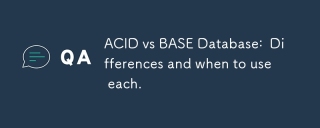
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
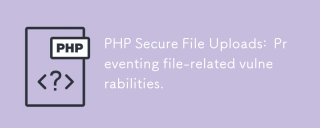
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
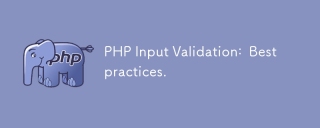
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
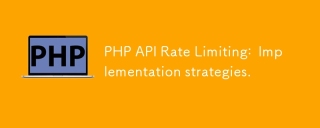
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
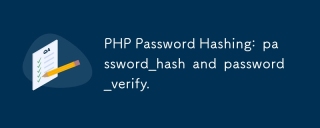
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
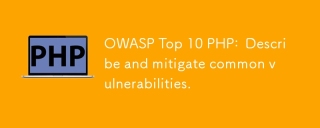
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
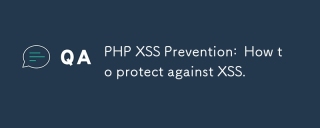
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
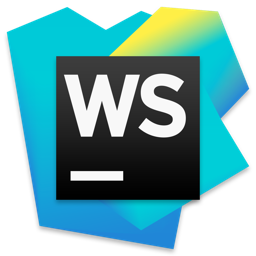
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
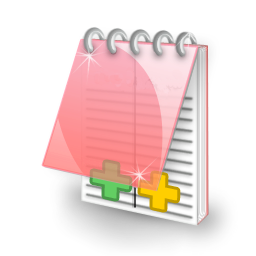
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software