1. What is it?
For example, if there is an 11 * 11 backgammon board, and we want to use a program to simulate it, it must be a two-dimensional array. Then use 1 to represent black stones and 2 to represent white stones. If there is only one black stone and one white stone on the chessboard, then there is only one 1 and one 2 in this two-dimensional array, and the others are meaningless 0s that do not represent any chess pieces, as follows:
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 ……
When most of the elements in an array are 0, or have the same value, you can use a sparse array to save the array. Why do this? Because it saves space.
2. How to use?
Record how many rows and columns the original array has and how many different values it has
Put the rows and columns of elements with different values and values are recorded in a small-scale array, this small-scale array is called a sparse array
3. Case:
The current situation is as follows The original array of 6 * 7:
0 0 0 22 0 0 15 0 11 0 0 0 17 0 0 0 0 -6 0 0 0 0 0 0 0 0 39 0 91 0 0 0 0 0 0 0 0 28 0 0 0 0
First, the first row and the first column of the sparse array are to record how many rows the element array has. The first row and the second column are to record how many columns the original array is. The first row and the first column are The three columns record how many different values the original array has (except 0). So one row of the sparse array should be:
行 列 值 6 7 8
Starting from the second row of the sparse array, each row records the row, column, and value size of the non-0 value in the original array. For example, if the second line is to record the row, column, and value of 22 in the original array, then the second line of the sparse array is:
行 列 值 0 3 22
Then use this method to record 15, 11, 17, -6, 39, 91 , 28 related information, so the sparse array finally converted from the original array is:
行 列 值 6 7 8 0 3 22 0 6 15 1 1 11 1 5 17 2 3 -6 3 5 39 4 0 91 5 2 28
This turns a 6 * 7 array into a 9 * 3 array, achieving the compression effect .
4. Ideas for converting original arrays and sparse arrays:
Convert original arrays to sparse arrays:
Traverse two dimensions The array gets the number of valid arrays count;
You can create a sparse array based on count
int[count 1][3]
;Save the valid array into a sparse array
Convert sparse array to original Array:
Read the first row of the sparse array. Based on the first row of the array, you can know how many rows and columns the original array has, and then create the original array;
Read the array of several rows after the sparse array and assign it to the original array
##5. Code practice:
public class SparseArray { public static void main(String[] args){ // 创建一个 11 * 11的原始数组 int[][] arr1 = new int[11][11]; arr1[1][2] = 1; arr1[2][3] = 2; // 原始数组转稀疏数组 // 1. 遍历,得到非0数据的个数以及所在的行列 int count = 0; Map<string> map = new HashMap(); for (int i = 0; i The above code realizes the mutual conversion between original array and sparse array. Flexible use of sparse array can save running memory and improve program performance. <p></p></string>
The above is the detailed content of How to convert original array to sparse array in java. For more information, please follow other related articles on the PHP Chinese website!
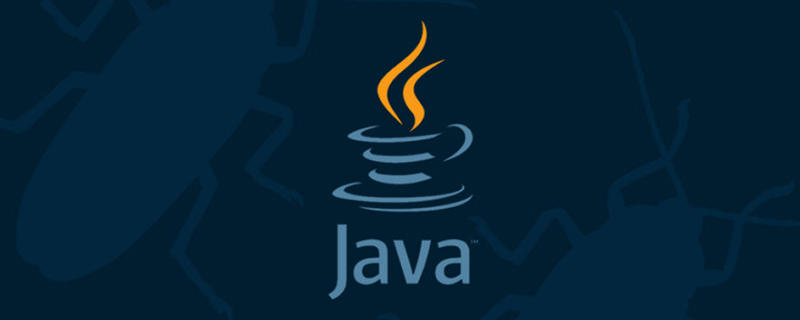
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
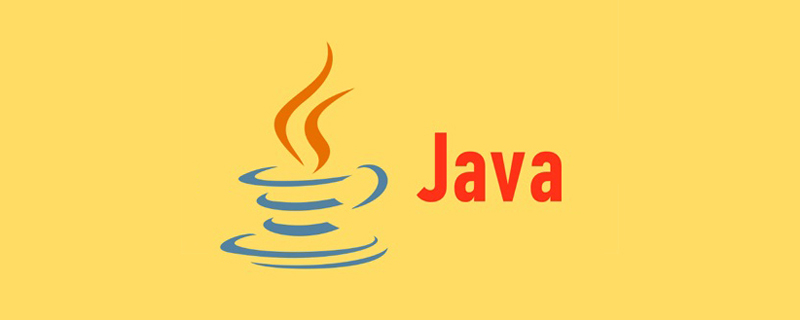
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
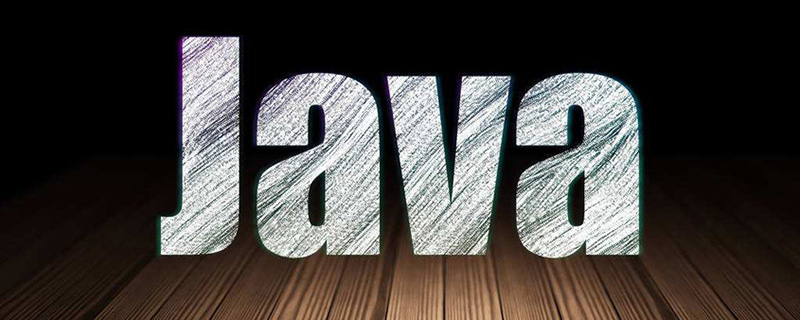
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
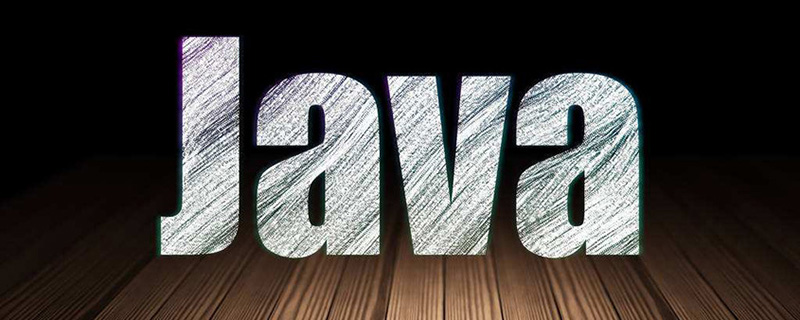
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
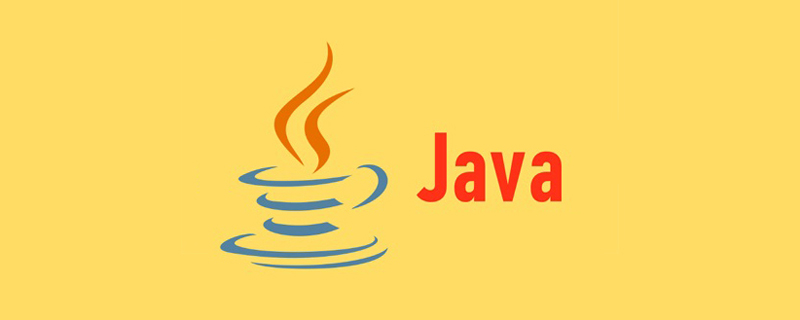
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
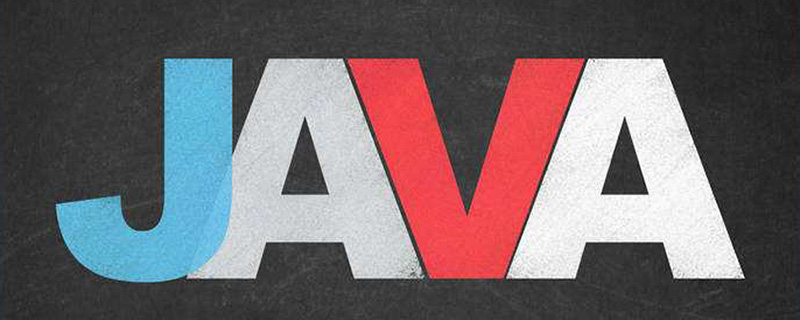
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
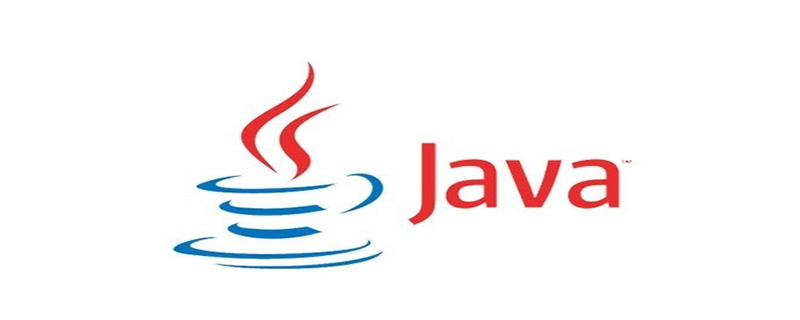
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
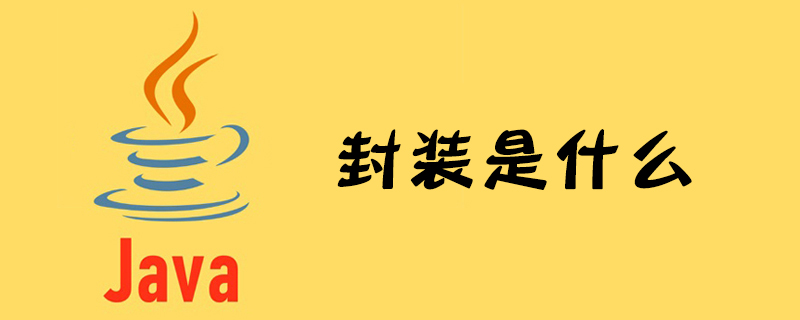
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
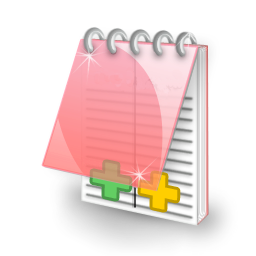
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
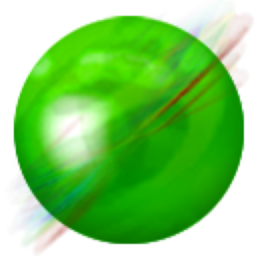
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
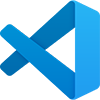
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
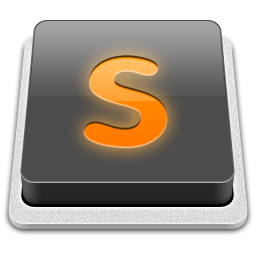
SublimeText3 Mac version
God-level code editing software (SublimeText3)
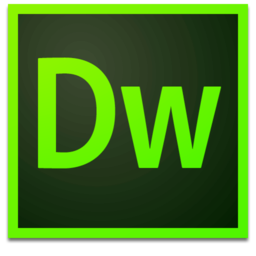
Dreamweaver Mac version
Visual web development tools