PHP is a programming language widely used in Web development. It has powerful array operation functions. In PHP, arrays are one of the most important and commonly used data types. This data structure allows developers to store a series of related values and retrieve and manipulate those values from them.
In PHP, a two-dimensional array is a special array type in which each array element contains an array. This data structure is commonly used to store matrices, tables, and other complex data structures. Since a two-dimensional array contains multiple arrays, different approaches are required when querying.
This article will introduce different methods of querying values in a two-dimensional array in PHP.
Method 1: Use foreach loop
In PHP, you can easily traverse all array elements in a two-dimensional array by using foreach loop. The following is an example 2D array:
$students = array(
array('name' => 'John', 'age' => 20, 'grade' => 'A'), array('name' => 'Jane', 'age' => 21, 'grade' => 'B'), array('name' => 'Jim', 'age' => 22, 'grade' => 'C')
);
Now, we want to retrieve the name "Jim" from this 2D array students’ grades.
We can use a foreach loop to loop through each student and check if their name is "Jim". If a match is found, the student's grade can be returned. Here is the sample code to implement this method:
foreach ($students as $student) {
if ($student['name'] == 'Jim') { echo $student['grade']; break; }
}
In the above code, we iterate over the $students array and assign it to a new array named $student. Next, we check if the "name" key in $student is equal to "Jim". If a match is found, use the echo command to output the student's "grade" value.
It should be noted that since the foreach loop traverses the entire array, when the required value is found, we have to use the break command to stop the search. An obvious disadvantage of this approach is that it loops over the entire array, and can cause performance issues in large arrays.
Method 2: Use for loop
Another way to query values in a two-dimensional array is to use a for loop. This method is faster than using a foreach loop, and because we know the position of the array we are searching for, unnecessary loop iterations can be skipped. The following is sample code to implement this method using a for loop:
for ($i = 0; $i
if ($students[$i]['name'] == 'Jim') { echo $students[$i]['grade']; break; }
}
In the above code snippet, we use a for loop to iterate through each element in the $students array and use the $i variable to keep track of the current iterator. We check if each student's "name" key is equal to "Jim" and if a match is found, we use the echo command to output the "grade" value for that student.
It should be noted that the condition of $i
Method 3: Use the array_column function
There is a convenient function in PHP called array_column(), which can be used to return a single column value from an array. This function accepts two parameters: an array and the column name to be returned. Here is sample code to use the array_column() function to return the "grade" column from the $students array above:
$grades = array_column($students, 'grade');
Now, We can find the required value simply by checking if the returned $grades array contains a specific value. The following is sample code to implement this method:
$student_name = 'Jim';
$grades = array_column($students, 'grade', 'name');
if (array_key_exists($ student_name, $grades)) {
echo $grades[$student_name];
}
In this code, use the array_column() function to get the "grade" column in the $students array, and use the "name" column as the key An associative array $grades is created. Next, we check if the $grades array contains a specific key (i.e. student name) and output the corresponding "grade" value.
Summary
In PHP, there are many different ways to query values in a two-dimensional array. In this article, we cover the three most common methods: using a foreach loop, using a for loop, and using the array_column() function.
Using foreach or for loop is suitable for small arrays or large arrays that require more powerful search capabilities. In contrast, the array_column() function is more suitable for filtering a single column. In actual development, developers can choose the appropriate method to query the values in the two-dimensional array according to specific requirements.
The above is the detailed content of How to query the value in a two-dimensional array in php. For more information, please follow other related articles on the PHP Chinese website!
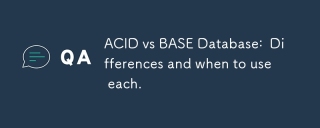
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
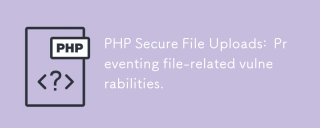
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
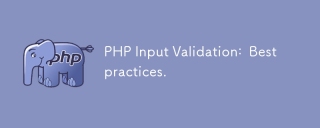
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
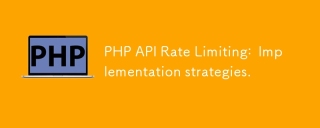
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
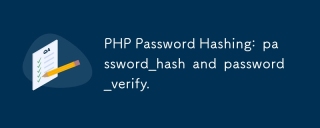
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
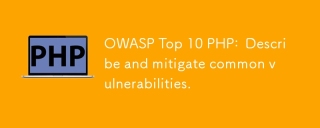
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
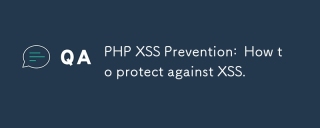
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
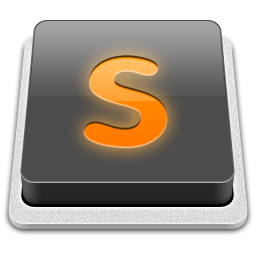
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
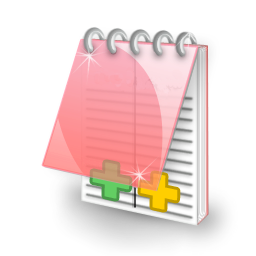
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function