Vim is a powerful text editor and the first choice of many programmers. In the process of developing Golang, proper annotations are very important. This article will introduce how to quickly write Golang comments in Vim.
1. Comment command in Vim
In Vim, you can use ":" in command mode to enter the command line mode, and then use the command to insert comments. The following are some common commands for inserting comments in Vim:
- Comment a single line: Enter "//" in command line mode, and then add "//" in front of the line to be commented.
- Comment multiple lines: first enter Visual mode, press "Shift v" within the line range that needs to be commented, then move the cursor to select the line to be commented, then enter the capital letter "I", and then enter "// ", and then press the Esc key. After this step, you will find that all the selected lines have been commented out.
- Uncomment: Enter ":" in command line mode, then enter "s/^//\s//" to remove the commented out single line comment. If you want to uncomment multiple lines, enter the command ":s/\v^\s(//|*)\s \zs//g".
2. Golang comment specifications
In Golang, comments have certain specifications. These specifications can help us read the code better and make it easier for others to understand our code. logic.
-
Single-line comments: Add "//" in front of the code that needs to be commented. For example:
// 这是一行注释 x := 1
-
Multi-line comments: add "/" and "/" before and after the code segment that needs to be commented. For example:
/* 这是一个多行注释 x := 1 y := 2 */
-
Function comments: Add a comment template in front of the function that needs to be commented. For example:
func SayHello(name string) { // SayHello is a function that takes in a name and returns a greeting string. fmt.Println("Hello, " + name) }
-
Parameter comment: Add a comment after the parameter. For example:
func SayHello(name string) { // name is a string representing the name of the person to greet. fmt.Println("Hello, " + name) }
-
Return value comment: Specify the return value type in the function definition, and add a return value comment after the function. For example:
// Add is a function that adds two integers and returns the result. func Add(x, y int) int { return x + y }
3. Golang automatically generates comments plug-in
For programmers who don’t want to write comments manually, you can use Golang automatically generate comments plug-in to quickly generate comments.
In Vim, you can use the vim-go plug-in to generate comments for functions and variables. Here's how to use the vim-go plug-in to automatically generate comments:
-
Install the vim-go plug-in: Run the following command in Vim:
:PluginInstall +vim-go
-
Generate function comments: Above the function that needs to be commented, enter ":" and enter the following command to automatically generate comments.
:GoDoc
The automatically generated results are as follows:
// SayHello is a function that takes in a name and returns a greeting string. func SayHello(name string) { fmt.Println("Hello, " + name) }
-
Generate variable comments: Move the cursor to the name of the variable that needs to be commented, and enter the following command to complete the automatic generation of comments.
:GoInfo
The generated results are as follows:
// name is a variable that holds a string representing the name of the person to greet. var name string = "Tom"
4. Summary
In Golang, appropriate comments contribute to the readability and maintainability of the code. Very important. This article introduces how to manually write comments in Vim and how to use the vim-go plug-in to automatically generate comments. I hope this article can help Golang developers better improve code quality.
The above is the detailed content of How to quickly write Golang comments in Vim. For more information, please follow other related articles on the PHP Chinese website!
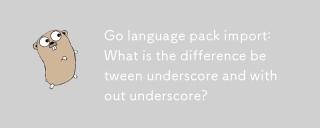
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
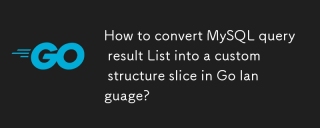
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
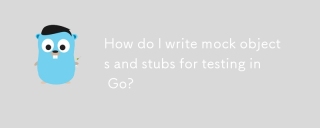
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
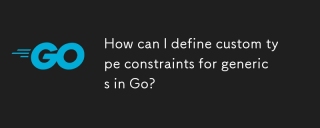
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
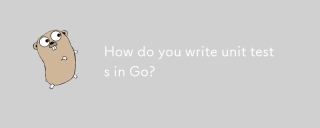
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
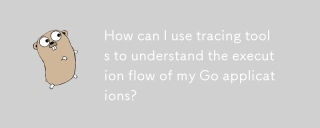
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
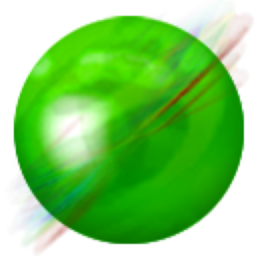
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
