AngularJS is a popular front-end framework that uses rich template statements and some powerful components to allow us to quickly develop high-quality web front-end applications. However, in many cases, we need to send requests and receive data through the backend server. In this case, we can use Node.js as the server and AngularJS for requests and responses. This article explains how to make requests to a Node.js server in AngularJS, and how to handle the response.
First, we need to define a service in the AngularJS application to handle requests and responses. It is possible to use the AngularJS built-in $http
service, but we can also customize the service to provide specific functionality. In this case, we will create a service called ServerRequestService
that will send the request and manage the returned response.
// 定义 ServerRequestService 服务 angular.module('myApp').factory('ServerRequestService', function($http) { var serverURL = 'http://localhost:3000'; // Node.js 服务器地址 return { // 发送 GET 请求到 Node.js 服务器 getRequest: function(route) { return $http.get(serverURL + '/' + route); }, // 发送 POST 请求到 Node.js 服务器 postRequest: function(route, data) { return $http.post(serverURL + '/' + route, data); } } });
The above code defines an AngularJS service named ServerRequestService
. The service accepts two parameters: the route to send the request and the request data. Other request types and parameters can be used as needed. In this case, we use the $http.get()
method and the $http.post()
method to send GET and POST requests. These methods take the request route and data as parameters and return the data Promise object.
Now we can send requests and handle responses using the ServerRequestService
service in our AngularJS application. Suppose we need to get all the user information in the database in the front-end application. Using the ServerRequestService
service you can do this:
// 获取所有用户信息 ServerRequestService.getRequest('users') .then(function(response) { $scope.users = response.data; }, function(error) { console.log(error); });
The above code uses the ServerRequestService
service to send a GET request to the route users
and return all user information the response to. After a successful response, the code assigns the response data to the $scope.users
object to display the user information in the application. When an error occurs, the code prints error information to the console.
By using the ServerRequestService
service, we can conveniently send requests and process responses in AngularJS applications. Using Node.js as backend server we can develop fast and reliable web applications.
The above is the detailed content of How to request Node.js server in Angular. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us

Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as. Data flow management: React's data flow is one-way, passed from the parent component to the child component, ensuring that the data flow is controllable, such as App components passing name to Greeting. Basic usage example: Use map function to render a list, you need to add a key attribute, such as rendering a fruit list. Advanced usage example: Use the useState hook to manage state and implement dynamics

React is the preferred tool for building single-page applications (SPAs) because it provides efficient and flexible ways to build user interfaces. 1) Component development: Split complex UI into independent and reusable parts to improve maintainability and reusability. 2) Virtual DOM: Optimize rendering performance by comparing the differences between virtual DOM and actual DOM. 3) State management: manage data flow through state and attributes to ensure data consistency and predictability.

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
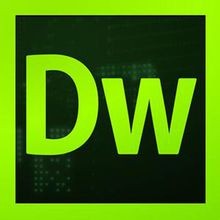
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor