With the development of front-end technology, Vue has become an indispensable part of modern web development. As an MVVM framework, Vue mainly builds user interfaces through data binding and component systems.
Vue’s componentized system allows developers to develop web applications in a more modular and reusable way, which is even more essential in large applications. This article will introduce Vue componentization and demonstrate how to use components in Vue applications.
- Basic of Vue componentization
In Vue, a component is composed of three parts:
- Template
- Data
- Behavior
Components can be used alone or in combination with multiple components. Each component can have its own data, behavior and templates.
- How to define Vue components
Vue components can be defined through component options. Each Vue component must define at least one template and one data object. The following is a simple Vue component definition example:
Vue.component('my-component', { template: '<div>{{ message }}</div>', data() { return { message: 'Hello, Vue!' }; } });
In this example, we define a component named "my-component" whose template defines a <div> element, in which the value of the component data object <code>message
is rendered. We can also add other properties and methods to this component:
Vue.component('my-component', { template: '<div>{{ message }}</div>', data() { return { message: 'Hello, Vue!' }; }, methods: { showMessage() { alert(this.message); } } });
In this example, we added a method named "showMessage", which is used to pop up a message box and display the component data object (message) value.
- Register component
We need to register the component in the Vue instance before we can use it in the application.
var vm = new Vue({ el: '#app', components: { 'my-component': { template: '<div>{{ message }}</div>', data() { return { message: 'Hello, Vue!' }; } } } });
In this example, we make the component available in the application by registering the component my-component
in the component options of the Vue instance.
- Nested components
We can reference another component in one component. This component nesting method is an important means to achieve component development in Vue.
Vue.component('my-component', { template: '<div><my-child></my-child></div>', components: { 'my-child': { template: '<p>Child component</p>' } } });
In this example, we define a component named "my-component" and nest a sub-component named "my-child" in the template. Note that we used the <my-child></my-child>
tag on the child component, which is how the component is referenced.
- Component communication
In Vue applications, communication between components is very common. In Vue, the parent component can pass data to the child component through Props, and the child component can pass the emit event, and finally pass the event to the parent component. The following is an example of a parent component passing data to a child component:
Vue.component('child-component', { template: '<p>{{ message }}</p>', props: ['message'] }); Vue.component('parent-component', { template: '<div><child-component></child-component></div>', data() { return { parentMessage: 'Data from parent component' }; } });
In this example, the value of parentMessage
in the parent component is passed to the child component, and the child component is bound through the attribute to receive the data. In child components, we can specify which properties can be received from the parent component by using the props
attribute.
- Summary
Componentization is a very important concept in Vue. Through Vue’s component system, we can separate different parts of the web page from each other, thereby making the code more Modular, reusable and maintainable. In this article, we introduced how Vue components are defined, registered, nested and communicated. I hope this article can provide you with some help in the development of Vue.
The above is the detailed content of How to use vue componentization. For more information, please follow other related articles on the PHP Chinese website!
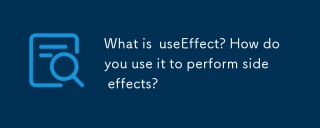
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
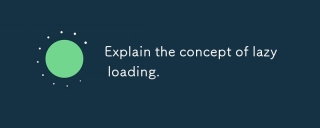
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
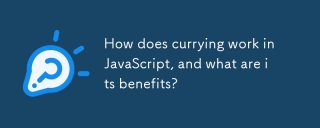
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
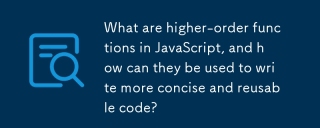
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
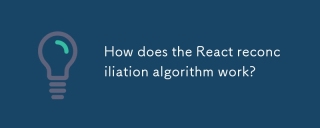
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
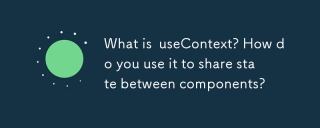
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
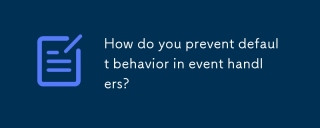
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
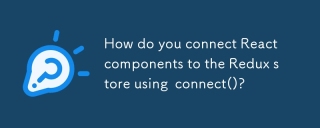
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
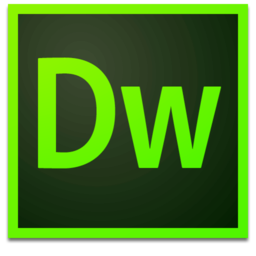
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
